refactor into modules
parent
82365d9fb0
commit
7c392e0dea
160
index.js
160
index.js
|
@ -1,25 +1,12 @@
|
|||
const https = require('https');
|
||||
const turndown = require('turndown');
|
||||
const { Readability } = require('@mozilla/readability');
|
||||
const JSDOM = require('jsdom').JSDOM;
|
||||
const common_filters = require('./url_to_markdown_common_filters');
|
||||
const apple_dev_parser = require('./url_to_markdown_apple_dev_docs.js');
|
||||
const table_to_markdown = require('./html_table_to_markdown.js');
|
||||
const readers = require('./url_to_markdown_readers.js');
|
||||
const processor = require('./url_to_markdown_processor.js');
|
||||
const validURL = require('@7c/validurl');
|
||||
const express = require('express');
|
||||
const rateLimit = require('express-rate-limit');
|
||||
const htmlEntities = require('html-entities');
|
||||
|
||||
const JSDOM = require('jsdom').JSDOM;
|
||||
const port = process.env.PORT;
|
||||
|
||||
const app = express();
|
||||
|
||||
const service = new turndown();
|
||||
|
||||
const apple_dev_prefix = "https://developer.apple.com";
|
||||
|
||||
const stackoverflow_prefix = "https://stackoverflow.com/questions";
|
||||
|
||||
const rateLimiter = rateLimit({
|
||||
windowMs: 30 * 1000,
|
||||
max: 5,
|
||||
|
@ -34,6 +21,18 @@ app.use(express.urlencoded({
|
|||
limit: '10mb'
|
||||
}));
|
||||
|
||||
function send_headers(res) {
|
||||
res.header("Access-Control-Allow-Origin", '*');
|
||||
res.header("Access-Control-Expose-Headers", 'X-Title');
|
||||
res.header("Content-Type", 'text/markdown');
|
||||
}
|
||||
|
||||
function read_url(url, res, inline_title, ignore_links) {
|
||||
reader = readers.reader_for_url(url);
|
||||
send_headers(res);
|
||||
reader.read_url(url, res, inline_title, ignore_links);
|
||||
}
|
||||
|
||||
app.get('/', (req, res) => {
|
||||
const url = req.query.url;
|
||||
const title = req.query.title;
|
||||
|
@ -47,14 +46,7 @@ app.get('/', (req, res) => {
|
|||
ignore_links = (links === 'false');
|
||||
}
|
||||
if (url && validURL(url)) {
|
||||
send_headers(res);
|
||||
if (url.startsWith(apple_dev_prefix)) {
|
||||
read_apple_url(url, res, inline_title, ignore_links);
|
||||
} else if (url.startsWith(stackoverflow_prefix)) {
|
||||
read_stack_url(url, res, inline_title, ignore_links);
|
||||
} else {
|
||||
read_url(url, res, inline_title, ignore_links);
|
||||
}
|
||||
read_url(url, res, inline_title, ignore_links);
|
||||
} else {
|
||||
res.status(400).send("Please specify a valid url query parameter");
|
||||
}
|
||||
|
@ -73,129 +65,23 @@ app.post('/', function(req, res) {
|
|||
if (links) {
|
||||
ignore_links = (links === 'false');
|
||||
}
|
||||
if (url && validURL(url) && url.startsWith(stackoverflow_prefix)) {
|
||||
send_headers(res);
|
||||
read_stack_url(url, res, inline_title, ignore_links);
|
||||
return;
|
||||
if (readers.ignore_post(url)) {
|
||||
read_url(url, res, inline_title, ignore_links);
|
||||
}
|
||||
if (!html) {
|
||||
res.status(400).send("Please provide a POST parameter called html");
|
||||
} else {
|
||||
try {
|
||||
//try {
|
||||
let document = new JSDOM(html);
|
||||
let markdown = process_dom(url, document, res, inline_title, ignore_links);
|
||||
let markdown = processor.process_dom(url, document, res, inline_title, ignore_links);
|
||||
send_headers(res);
|
||||
res.send(markdown);
|
||||
} catch (error) {
|
||||
res.status(400).send("Could not parse that document");
|
||||
}
|
||||
//} catch (error) {
|
||||
//res.status(400).send("Could not parse that document");
|
||||
//}
|
||||
}
|
||||
|
||||
});
|
||||
|
||||
app.listen(port, () => {
|
||||
})
|
||||
|
||||
function send_headers(res) {
|
||||
res.header("Access-Control-Allow-Origin", '*');
|
||||
res.header("Access-Control-Expose-Headers", 'X-Title');
|
||||
res.header("Content-Type", 'text/markdown');
|
||||
}
|
||||
|
||||
function process_dom(url, document, res, inline_title, ignore_links, id="") {
|
||||
let title = document.window.document.querySelector('title');
|
||||
if (title)
|
||||
res.header("X-Title", encodeURIComponent(title.textContent));
|
||||
if (id) {
|
||||
document = new JSDOM('<!DOCTYPE html>'+ document.window.document.querySelector("#"+id).innerHTML);
|
||||
}
|
||||
let reader = new Readability(document.window.document);
|
||||
let readable = reader.parse().content;
|
||||
let replacements = [];
|
||||
readable = format_codeblocks(readable, replacements);
|
||||
readable = format_tables(readable, replacements);
|
||||
let markdown = service.turndown(readable);
|
||||
for (let i=0;i<replacements.length;i++) {
|
||||
markdown = markdown.replace(replacements[i].placeholder, replacements[i].replacement);
|
||||
}
|
||||
let result = (url) ? common_filters.filter(url, markdown, ignore_links) : markdown;
|
||||
if (inline_title && title) {
|
||||
result = "# " + title.textContent + "\n" + result;
|
||||
}
|
||||
return result;
|
||||
}
|
||||
|
||||
function read_url(url, res, inline_title, ignore_links) {
|
||||
JSDOM.fromURL(url).then((document)=>{
|
||||
let markdown = process_dom(url, document, res, inline_title, ignore_links);
|
||||
res.send(markdown);
|
||||
}).catch((error)=> {
|
||||
res.status(400).send("Sorry, could not fetch and convert that URL");
|
||||
});
|
||||
}
|
||||
|
||||
function read_stack_url(url, res, inline_title, ignore_links) {
|
||||
JSDOM.fromURL(url).then((document)=>{
|
||||
let markdown_q = process_dom(url, document, res, inline_title, ignore_links, 'question');
|
||||
let markdown_a = process_dom(url, document, res, false, ignore_links, 'answers');
|
||||
if (markdown_a.startsWith('Your Answer')) {
|
||||
res.send(markdown_q);
|
||||
}
|
||||
else {
|
||||
res.send(markdown_q + "\n\n## Answer\n"+ markdown_a);
|
||||
}
|
||||
}).catch((error)=> {
|
||||
res.status(400).send("Sorry, could not fetch and convert that URL");
|
||||
});
|
||||
}
|
||||
|
||||
function read_apple_url(url, res, inline_title, ignore_links) {
|
||||
json_url = apple_dev_parser.dev_doc_url(url);
|
||||
https.get(json_url,(apple_res) => {
|
||||
let body = "";
|
||||
apple_res.on("data", (chunk) => {
|
||||
body += chunk;
|
||||
});
|
||||
apple_res.on("end", () => {
|
||||
let json = JSON.parse(body);
|
||||
let markdown = apple_dev_parser.parse_dev_doc_json(json, inline_title, ignore_links);
|
||||
res.send(markdown);
|
||||
});
|
||||
})
|
||||
}
|
||||
|
||||
function format_tables(html, replacements) {
|
||||
const start = replacements.length;
|
||||
const tables = html.match(/(<table[^>]*>(?:.|\n)*?<\/table>)/gi);
|
||||
if (tables) {
|
||||
for (let t=0;t<tables.length;t++) {
|
||||
const table = tables[t];
|
||||
let markdown = table_to_markdown.convert(table);
|
||||
let placeholder = "urltomarkdowntableplaceholder"+t+Math.random();
|
||||
replacements[start+t] = { placeholder: placeholder, replacement: markdown};
|
||||
html = html.replace(table, "<p>"+placeholder+"</p>");
|
||||
}
|
||||
}
|
||||
return html;
|
||||
}
|
||||
|
||||
function format_codeblocks(html, replacements) {
|
||||
const start = replacements.length;
|
||||
const codeblocks = html.match(/(<pre[^>]*>(?:.|\n)*?<\/pre>)/gi);
|
||||
if (codeblocks) {
|
||||
for (let c=0;c<codeblocks.length;c++) {
|
||||
const codeblock = codeblocks[c];
|
||||
let filtered = codeblock;
|
||||
filtered = filtered.replace(/<br[^>]*>/g, "\n");
|
||||
filtered = filtered.replace(/<p>/g, "\n");
|
||||
filtered = filtered.replace(/<\/?[^>]+(>|$)/g, "");
|
||||
filtered = htmlEntities.decode(filtered);
|
||||
let markdown = "```\n"+filtered+"\n```\n";
|
||||
let placeholder = "urltomarkdowncodeblockplaceholder"+c+Math.random();
|
||||
replacements[start+c] = { placeholder: placeholder, replacement: markdown};
|
||||
html = html.replace(codeblock, "<p>"+placeholder+"</p>");
|
||||
}
|
||||
}
|
||||
return html;
|
||||
}
|
||||
|
||||
|
|
|
@ -31,12 +31,12 @@
|
|||
"integrity": "sha512-9IK9EadsbHo6jLWIpxpR6pL0sazTXV6+SQv25ZB+F7Bj9mJNaOc4nCRabwd5M/JwmUa8idz6Eci6eKfJryPs6Q=="
|
||||
},
|
||||
"node_modules/accepts": {
|
||||
"version": "1.3.7",
|
||||
"resolved": "https://registry.npmjs.org/accepts/-/accepts-1.3.7.tgz",
|
||||
"integrity": "sha512-Il80Qs2WjYlJIBNzNkK6KYqlVMTbZLXgHx2oT0pU/fjRHyEp+PEfEPY0R3WCwAGVOtauxh1hOxNgIf5bv7dQpA==",
|
||||
"version": "1.3.8",
|
||||
"resolved": "https://registry.npmjs.org/accepts/-/accepts-1.3.8.tgz",
|
||||
"integrity": "sha512-PYAthTa2m2VKxuvSD3DPC/Gy+U+sOA1LAuT8mkmRuvw+NACSaeXEQ+NHcVF7rONl6qcaxV3Uuemwawk+7+SJLw==",
|
||||
"dependencies": {
|
||||
"mime-types": "~2.1.24",
|
||||
"negotiator": "0.6.2"
|
||||
"mime-types": "~2.1.34",
|
||||
"negotiator": "0.6.3"
|
||||
},
|
||||
"engines": {
|
||||
"node": ">= 0.6"
|
||||
|
@ -124,23 +124,26 @@
|
|||
"integrity": "sha1-x57Zf380y48robyXkLzDZkdLS3k="
|
||||
},
|
||||
"node_modules/body-parser": {
|
||||
"version": "1.19.1",
|
||||
"resolved": "https://registry.npmjs.org/body-parser/-/body-parser-1.19.1.tgz",
|
||||
"integrity": "sha512-8ljfQi5eBk8EJfECMrgqNGWPEY5jWP+1IzkzkGdFFEwFQZZyaZ21UqdaHktgiMlH0xLHqIFtE/u2OYE5dOtViA==",
|
||||
"version": "1.20.1",
|
||||
"resolved": "https://registry.npmjs.org/body-parser/-/body-parser-1.20.1.tgz",
|
||||
"integrity": "sha512-jWi7abTbYwajOytWCQc37VulmWiRae5RyTpaCyDcS5/lMdtwSz5lOpDE67srw/HYe35f1z3fDQw+3txg7gNtWw==",
|
||||
"dependencies": {
|
||||
"bytes": "3.1.1",
|
||||
"bytes": "3.1.2",
|
||||
"content-type": "~1.0.4",
|
||||
"debug": "2.6.9",
|
||||
"depd": "~1.1.2",
|
||||
"http-errors": "1.8.1",
|
||||
"depd": "2.0.0",
|
||||
"destroy": "1.2.0",
|
||||
"http-errors": "2.0.0",
|
||||
"iconv-lite": "0.4.24",
|
||||
"on-finished": "~2.3.0",
|
||||
"qs": "6.9.6",
|
||||
"raw-body": "2.4.2",
|
||||
"type-is": "~1.6.18"
|
||||
"on-finished": "2.4.1",
|
||||
"qs": "6.11.0",
|
||||
"raw-body": "2.5.1",
|
||||
"type-is": "~1.6.18",
|
||||
"unpipe": "1.0.0"
|
||||
},
|
||||
"engines": {
|
||||
"node": ">= 0.8"
|
||||
"node": ">= 0.8",
|
||||
"npm": "1.2.8000 || >= 1.4.16"
|
||||
}
|
||||
},
|
||||
"node_modules/browser-process-hrtime": {
|
||||
|
@ -149,13 +152,25 @@
|
|||
"integrity": "sha512-9o5UecI3GhkpM6DrXr69PblIuWxPKk9Y0jHBRhdocZ2y7YECBFCsHm79Pr3OyR2AvjhDkabFJaDJMYRazHgsow=="
|
||||
},
|
||||
"node_modules/bytes": {
|
||||
"version": "3.1.1",
|
||||
"resolved": "https://registry.npmjs.org/bytes/-/bytes-3.1.1.tgz",
|
||||
"integrity": "sha512-dWe4nWO/ruEOY7HkUJ5gFt1DCFV9zPRoJr8pV0/ASQermOZjtq8jMjOprC0Kd10GLN+l7xaUPvxzJFWtxGu8Fg==",
|
||||
"version": "3.1.2",
|
||||
"resolved": "https://registry.npmjs.org/bytes/-/bytes-3.1.2.tgz",
|
||||
"integrity": "sha512-/Nf7TyzTx6S3yRJObOAV7956r8cr2+Oj8AC5dt8wSP3BQAoeX58NoHyCU8P8zGkNXStjTSi6fzO6F0pBdcYbEg==",
|
||||
"engines": {
|
||||
"node": ">= 0.8"
|
||||
}
|
||||
},
|
||||
"node_modules/call-bind": {
|
||||
"version": "1.0.2",
|
||||
"resolved": "https://registry.npmjs.org/call-bind/-/call-bind-1.0.2.tgz",
|
||||
"integrity": "sha512-7O+FbCihrB5WGbFYesctwmTKae6rOiIzmz1icreWJ+0aA7LJfuqhEso2T9ncpcFtzMQtzXf2QGGueWJGTYsqrA==",
|
||||
"dependencies": {
|
||||
"function-bind": "^1.1.1",
|
||||
"get-intrinsic": "^1.0.2"
|
||||
},
|
||||
"funding": {
|
||||
"url": "https://github.com/sponsors/ljharb"
|
||||
}
|
||||
},
|
||||
"node_modules/combined-stream": {
|
||||
"version": "1.0.8",
|
||||
"resolved": "https://registry.npmjs.org/combined-stream/-/combined-stream-1.0.8.tgz",
|
||||
|
@ -187,9 +202,9 @@
|
|||
}
|
||||
},
|
||||
"node_modules/cookie": {
|
||||
"version": "0.4.1",
|
||||
"resolved": "https://registry.npmjs.org/cookie/-/cookie-0.4.1.tgz",
|
||||
"integrity": "sha512-ZwrFkGJxUR3EIoXtO+yVE69Eb7KlixbaeAWfBQB9vVsNn/o+Yw69gBWSSDK825hQNdN+wF8zELf3dFNl/kxkUA==",
|
||||
"version": "0.5.0",
|
||||
"resolved": "https://registry.npmjs.org/cookie/-/cookie-0.5.0.tgz",
|
||||
"integrity": "sha512-YZ3GUyn/o8gfKJlnlX7g7xq4gyO6OSuhGPKaaGssGB2qgDUS0gPgtTvoyZLTt9Ab6dC4hfc9dV5arkvc/OCmrw==",
|
||||
"engines": {
|
||||
"node": ">= 0.6"
|
||||
}
|
||||
|
@ -260,17 +275,21 @@
|
|||
}
|
||||
},
|
||||
"node_modules/depd": {
|
||||
"version": "1.1.2",
|
||||
"resolved": "https://registry.npmjs.org/depd/-/depd-1.1.2.tgz",
|
||||
"integrity": "sha1-m81S4UwJd2PnSbJ0xDRu0uVgtak=",
|
||||
"version": "2.0.0",
|
||||
"resolved": "https://registry.npmjs.org/depd/-/depd-2.0.0.tgz",
|
||||
"integrity": "sha512-g7nH6P6dyDioJogAAGprGpCtVImJhpPk/roCzdb3fIh61/s/nPsfR6onyMwkCAR/OlC3yBC0lESvUoQEAssIrw==",
|
||||
"engines": {
|
||||
"node": ">= 0.6"
|
||||
"node": ">= 0.8"
|
||||
}
|
||||
},
|
||||
"node_modules/destroy": {
|
||||
"version": "1.0.4",
|
||||
"resolved": "https://registry.npmjs.org/destroy/-/destroy-1.0.4.tgz",
|
||||
"integrity": "sha1-l4hXRCxEdJ5CBmE+N5RiBYJqvYA="
|
||||
"version": "1.2.0",
|
||||
"resolved": "https://registry.npmjs.org/destroy/-/destroy-1.2.0.tgz",
|
||||
"integrity": "sha512-2sJGJTaXIIaR1w4iJSNoN0hnMY7Gpc/n8D4qSCJw8QqFWXf7cuAgnEHxBpweaVcPevC2l3KpjYCx3NypQQgaJg==",
|
||||
"engines": {
|
||||
"node": ">= 0.8",
|
||||
"npm": "1.2.8000 || >= 1.4.16"
|
||||
}
|
||||
},
|
||||
"node_modules/domexception": {
|
||||
"version": "2.0.1",
|
||||
|
@ -299,12 +318,12 @@
|
|||
"node_modules/ee-first": {
|
||||
"version": "1.1.1",
|
||||
"resolved": "https://registry.npmjs.org/ee-first/-/ee-first-1.1.1.tgz",
|
||||
"integrity": "sha1-WQxhFWsK4vTwJVcyoViyZrxWsh0="
|
||||
"integrity": "sha512-WMwm9LhRUo+WUaRN+vRuETqG89IgZphVSNkdFgeb6sS/E4OrDIN7t48CAewSHXc6C8lefD8KKfr5vY61brQlow=="
|
||||
},
|
||||
"node_modules/encodeurl": {
|
||||
"version": "1.0.2",
|
||||
"resolved": "https://registry.npmjs.org/encodeurl/-/encodeurl-1.0.2.tgz",
|
||||
"integrity": "sha1-rT/0yG7C0CkyL1oCw6mmBslbP1k=",
|
||||
"integrity": "sha512-TPJXq8JqFaVYm2CWmPvnP2Iyo4ZSM7/QKcSmuMLDObfpH5fi7RUGmd/rTDf+rut/saiDiQEeVTNgAmJEdAOx0w==",
|
||||
"engines": {
|
||||
"node": ">= 0.8"
|
||||
}
|
||||
|
@ -312,7 +331,7 @@
|
|||
"node_modules/escape-html": {
|
||||
"version": "1.0.3",
|
||||
"resolved": "https://registry.npmjs.org/escape-html/-/escape-html-1.0.3.tgz",
|
||||
"integrity": "sha1-Aljq5NPQwJdN4cFpGI7wBR0dGYg="
|
||||
"integrity": "sha512-NiSupZ4OeuGwr68lGIeym/ksIZMJodUGOSCZ/FSnTxcrekbvqrgdUxlJOMpijaKZVjAJrWrGs/6Jy8OMuyj9ow=="
|
||||
},
|
||||
"node_modules/escodegen": {
|
||||
"version": "2.0.0",
|
||||
|
@ -366,43 +385,44 @@
|
|||
"node_modules/etag": {
|
||||
"version": "1.8.1",
|
||||
"resolved": "https://registry.npmjs.org/etag/-/etag-1.8.1.tgz",
|
||||
"integrity": "sha1-Qa4u62XvpiJorr/qg6x9eSmbCIc=",
|
||||
"integrity": "sha512-aIL5Fx7mawVa300al2BnEE4iNvo1qETxLrPI/o05L7z6go7fCw1J6EQmbK4FmJ2AS7kgVF/KEZWufBfdClMcPg==",
|
||||
"engines": {
|
||||
"node": ">= 0.6"
|
||||
}
|
||||
},
|
||||
"node_modules/express": {
|
||||
"version": "4.17.2",
|
||||
"resolved": "https://registry.npmjs.org/express/-/express-4.17.2.tgz",
|
||||
"integrity": "sha512-oxlxJxcQlYwqPWKVJJtvQiwHgosH/LrLSPA+H4UxpyvSS6jC5aH+5MoHFM+KABgTOt0APue4w66Ha8jCUo9QGg==",
|
||||
"version": "4.18.2",
|
||||
"resolved": "https://registry.npmjs.org/express/-/express-4.18.2.tgz",
|
||||
"integrity": "sha512-5/PsL6iGPdfQ/lKM1UuielYgv3BUoJfz1aUwU9vHZ+J7gyvwdQXFEBIEIaxeGf0GIcreATNyBExtalisDbuMqQ==",
|
||||
"dependencies": {
|
||||
"accepts": "~1.3.7",
|
||||
"accepts": "~1.3.8",
|
||||
"array-flatten": "1.1.1",
|
||||
"body-parser": "1.19.1",
|
||||
"body-parser": "1.20.1",
|
||||
"content-disposition": "0.5.4",
|
||||
"content-type": "~1.0.4",
|
||||
"cookie": "0.4.1",
|
||||
"cookie": "0.5.0",
|
||||
"cookie-signature": "1.0.6",
|
||||
"debug": "2.6.9",
|
||||
"depd": "~1.1.2",
|
||||
"depd": "2.0.0",
|
||||
"encodeurl": "~1.0.2",
|
||||
"escape-html": "~1.0.3",
|
||||
"etag": "~1.8.1",
|
||||
"finalhandler": "~1.1.2",
|
||||
"finalhandler": "1.2.0",
|
||||
"fresh": "0.5.2",
|
||||
"http-errors": "2.0.0",
|
||||
"merge-descriptors": "1.0.1",
|
||||
"methods": "~1.1.2",
|
||||
"on-finished": "~2.3.0",
|
||||
"on-finished": "2.4.1",
|
||||
"parseurl": "~1.3.3",
|
||||
"path-to-regexp": "0.1.7",
|
||||
"proxy-addr": "~2.0.7",
|
||||
"qs": "6.9.6",
|
||||
"qs": "6.11.0",
|
||||
"range-parser": "~1.2.1",
|
||||
"safe-buffer": "5.2.1",
|
||||
"send": "0.17.2",
|
||||
"serve-static": "1.14.2",
|
||||
"send": "0.18.0",
|
||||
"serve-static": "1.15.0",
|
||||
"setprototypeof": "1.2.0",
|
||||
"statuses": "~1.5.0",
|
||||
"statuses": "2.0.1",
|
||||
"type-is": "~1.6.18",
|
||||
"utils-merge": "1.0.1",
|
||||
"vary": "~1.1.2"
|
||||
|
@ -428,16 +448,16 @@
|
|||
"integrity": "sha1-PYpcZog6FqMMqGQ+hR8Zuqd5eRc="
|
||||
},
|
||||
"node_modules/finalhandler": {
|
||||
"version": "1.1.2",
|
||||
"resolved": "https://registry.npmjs.org/finalhandler/-/finalhandler-1.1.2.tgz",
|
||||
"integrity": "sha512-aAWcW57uxVNrQZqFXjITpW3sIUQmHGG3qSb9mUah9MgMC4NeWhNOlNjXEYq3HjRAvL6arUviZGGJsBg6z0zsWA==",
|
||||
"version": "1.2.0",
|
||||
"resolved": "https://registry.npmjs.org/finalhandler/-/finalhandler-1.2.0.tgz",
|
||||
"integrity": "sha512-5uXcUVftlQMFnWC9qu/svkWv3GTd2PfUhK/3PLkYNAe7FbqJMt3515HaxE6eRL74GdsriiwujiawdaB1BpEISg==",
|
||||
"dependencies": {
|
||||
"debug": "2.6.9",
|
||||
"encodeurl": "~1.0.2",
|
||||
"escape-html": "~1.0.3",
|
||||
"on-finished": "~2.3.0",
|
||||
"on-finished": "2.4.1",
|
||||
"parseurl": "~1.3.3",
|
||||
"statuses": "~1.5.0",
|
||||
"statuses": "2.0.1",
|
||||
"unpipe": "~1.0.0"
|
||||
},
|
||||
"engines": {
|
||||
|
@ -468,11 +488,51 @@
|
|||
"node_modules/fresh": {
|
||||
"version": "0.5.2",
|
||||
"resolved": "https://registry.npmjs.org/fresh/-/fresh-0.5.2.tgz",
|
||||
"integrity": "sha1-PYyt2Q2XZWn6g1qx+OSyOhBWBac=",
|
||||
"integrity": "sha512-zJ2mQYM18rEFOudeV4GShTGIQ7RbzA7ozbU9I/XBpm7kqgMywgmylMwXHxZJmkVoYkna9d2pVXVXPdYTP9ej8Q==",
|
||||
"engines": {
|
||||
"node": ">= 0.6"
|
||||
}
|
||||
},
|
||||
"node_modules/function-bind": {
|
||||
"version": "1.1.1",
|
||||
"resolved": "https://registry.npmjs.org/function-bind/-/function-bind-1.1.1.tgz",
|
||||
"integrity": "sha512-yIovAzMX49sF8Yl58fSCWJ5svSLuaibPxXQJFLmBObTuCr0Mf1KiPopGM9NiFjiYBCbfaa2Fh6breQ6ANVTI0A=="
|
||||
},
|
||||
"node_modules/get-intrinsic": {
|
||||
"version": "1.1.3",
|
||||
"resolved": "https://registry.npmjs.org/get-intrinsic/-/get-intrinsic-1.1.3.tgz",
|
||||
"integrity": "sha512-QJVz1Tj7MS099PevUG5jvnt9tSkXN8K14dxQlikJuPt4uD9hHAHjLyLBiLR5zELelBdD9QNRAXZzsJx0WaDL9A==",
|
||||
"dependencies": {
|
||||
"function-bind": "^1.1.1",
|
||||
"has": "^1.0.3",
|
||||
"has-symbols": "^1.0.3"
|
||||
},
|
||||
"funding": {
|
||||
"url": "https://github.com/sponsors/ljharb"
|
||||
}
|
||||
},
|
||||
"node_modules/has": {
|
||||
"version": "1.0.3",
|
||||
"resolved": "https://registry.npmjs.org/has/-/has-1.0.3.tgz",
|
||||
"integrity": "sha512-f2dvO0VU6Oej7RkWJGrehjbzMAjFp5/VKPp5tTpWIV4JHHZK1/BxbFRtf/siA2SWTe09caDmVtYYzWEIbBS4zw==",
|
||||
"dependencies": {
|
||||
"function-bind": "^1.1.1"
|
||||
},
|
||||
"engines": {
|
||||
"node": ">= 0.4.0"
|
||||
}
|
||||
},
|
||||
"node_modules/has-symbols": {
|
||||
"version": "1.0.3",
|
||||
"resolved": "https://registry.npmjs.org/has-symbols/-/has-symbols-1.0.3.tgz",
|
||||
"integrity": "sha512-l3LCuF6MgDNwTDKkdYGEihYjt5pRPbEg46rtlmnSPlUbgmB8LOIrKJbYYFBSbnPaJexMKtiPO8hmeRjRz2Td+A==",
|
||||
"engines": {
|
||||
"node": ">= 0.4"
|
||||
},
|
||||
"funding": {
|
||||
"url": "https://github.com/sponsors/ljharb"
|
||||
}
|
||||
},
|
||||
"node_modules/html-encoding-sniffer": {
|
||||
"version": "2.0.1",
|
||||
"resolved": "https://registry.npmjs.org/html-encoding-sniffer/-/html-encoding-sniffer-2.0.1.tgz",
|
||||
|
@ -490,18 +550,18 @@
|
|||
"integrity": "sha512-DV5Ln36z34NNTDgnz0EWGBLZENelNAtkiFA4kyNOG2tDI6Mz1uSWiq1wAKdyjnJwyDiDO7Fa2SO1CTxPXL8VxA=="
|
||||
},
|
||||
"node_modules/http-errors": {
|
||||
"version": "1.8.1",
|
||||
"resolved": "https://registry.npmjs.org/http-errors/-/http-errors-1.8.1.tgz",
|
||||
"integrity": "sha512-Kpk9Sm7NmI+RHhnj6OIWDI1d6fIoFAtFt9RLaTMRlg/8w49juAStsrBgp0Dp4OdxdVbRIeKhtCUvoi/RuAhO4g==",
|
||||
"version": "2.0.0",
|
||||
"resolved": "https://registry.npmjs.org/http-errors/-/http-errors-2.0.0.tgz",
|
||||
"integrity": "sha512-FtwrG/euBzaEjYeRqOgly7G0qviiXoJWnvEH2Z1plBdXgbyjv34pHTSb9zoeHMyDy33+DWy5Wt9Wo+TURtOYSQ==",
|
||||
"dependencies": {
|
||||
"depd": "~1.1.2",
|
||||
"depd": "2.0.0",
|
||||
"inherits": "2.0.4",
|
||||
"setprototypeof": "1.2.0",
|
||||
"statuses": ">= 1.5.0 < 2",
|
||||
"statuses": "2.0.1",
|
||||
"toidentifier": "1.0.1"
|
||||
},
|
||||
"engines": {
|
||||
"node": ">= 0.6"
|
||||
"node": ">= 0.8"
|
||||
}
|
||||
},
|
||||
"node_modules/http-proxy-agent": {
|
||||
|
@ -670,7 +730,7 @@
|
|||
"node_modules/media-typer": {
|
||||
"version": "0.3.0",
|
||||
"resolved": "https://registry.npmjs.org/media-typer/-/media-typer-0.3.0.tgz",
|
||||
"integrity": "sha1-hxDXrwqmJvj/+hzgAWhUUmMlV0g=",
|
||||
"integrity": "sha512-dq+qelQ9akHpcOl/gUVRTxVIOkAJ1wR3QAvb4RsVjS8oVoFjDGTc679wJYmUmknUF5HwMLOgb5O+a3KxfWapPQ==",
|
||||
"engines": {
|
||||
"node": ">= 0.6"
|
||||
}
|
||||
|
@ -721,12 +781,12 @@
|
|||
"node_modules/ms": {
|
||||
"version": "2.0.0",
|
||||
"resolved": "https://registry.npmjs.org/ms/-/ms-2.0.0.tgz",
|
||||
"integrity": "sha1-VgiurfwAvmwpAd9fmGF4jeDVl8g="
|
||||
"integrity": "sha512-Tpp60P6IUJDTuOq/5Z8cdskzJujfwqfOTkrwIwj7IRISpnkJnT6SyJ4PCPnGMoFjC9ddhal5KVIYtAt97ix05A=="
|
||||
},
|
||||
"node_modules/negotiator": {
|
||||
"version": "0.6.2",
|
||||
"resolved": "https://registry.npmjs.org/negotiator/-/negotiator-0.6.2.tgz",
|
||||
"integrity": "sha512-hZXc7K2e+PgeI1eDBe/10Ard4ekbfrrqG8Ep+8Jmf4JID2bNg7NvCPOZN+kfF574pFQI7mum2AUqDidoKqcTOw==",
|
||||
"version": "0.6.3",
|
||||
"resolved": "https://registry.npmjs.org/negotiator/-/negotiator-0.6.3.tgz",
|
||||
"integrity": "sha512-+EUsqGPLsM+j/zdChZjsnX51g4XrHFOIXwfnCVPGlQk/k5giakcKsuxCObBRu6DSm9opw/O6slWbJdghQM4bBg==",
|
||||
"engines": {
|
||||
"node": ">= 0.6"
|
||||
}
|
||||
|
@ -736,10 +796,18 @@
|
|||
"resolved": "https://registry.npmjs.org/nwsapi/-/nwsapi-2.2.0.tgz",
|
||||
"integrity": "sha512-h2AatdwYH+JHiZpv7pt/gSX1XoRGb7L/qSIeuqA6GwYoF9w1vP1cw42TO0aI2pNyshRK5893hNSl+1//vHK7hQ=="
|
||||
},
|
||||
"node_modules/object-inspect": {
|
||||
"version": "1.12.2",
|
||||
"resolved": "https://registry.npmjs.org/object-inspect/-/object-inspect-1.12.2.tgz",
|
||||
"integrity": "sha512-z+cPxW0QGUp0mcqcsgQyLVRDoXFQbXOwBaqyF7VIgI4TWNQsDHrBpUQslRmIfAoYWdYzs6UlKJtB2XJpTaNSpQ==",
|
||||
"funding": {
|
||||
"url": "https://github.com/sponsors/ljharb"
|
||||
}
|
||||
},
|
||||
"node_modules/on-finished": {
|
||||
"version": "2.3.0",
|
||||
"resolved": "https://registry.npmjs.org/on-finished/-/on-finished-2.3.0.tgz",
|
||||
"integrity": "sha1-IPEzZIGwg811M3mSoWlxqi2QaUc=",
|
||||
"version": "2.4.1",
|
||||
"resolved": "https://registry.npmjs.org/on-finished/-/on-finished-2.4.1.tgz",
|
||||
"integrity": "sha512-oVlzkg3ENAhCk2zdv7IJwd/QUD4z2RxRwpkcGY8psCVcCYZNq4wYnVWALHM+brtuJjePWiYF/ClmuDr8Ch5+kg==",
|
||||
"dependencies": {
|
||||
"ee-first": "1.1.1"
|
||||
},
|
||||
|
@ -815,9 +883,12 @@
|
|||
}
|
||||
},
|
||||
"node_modules/qs": {
|
||||
"version": "6.9.6",
|
||||
"resolved": "https://registry.npmjs.org/qs/-/qs-6.9.6.tgz",
|
||||
"integrity": "sha512-TIRk4aqYLNoJUbd+g2lEdz5kLWIuTMRagAXxl78Q0RiVjAOugHmeKNGdd3cwo/ktpf9aL9epCfFqWDEKysUlLQ==",
|
||||
"version": "6.11.0",
|
||||
"resolved": "https://registry.npmjs.org/qs/-/qs-6.11.0.tgz",
|
||||
"integrity": "sha512-MvjoMCJwEarSbUYk5O+nmoSzSutSsTwF85zcHPQ9OrlFoZOYIjaqBAJIqIXjptyD5vThxGq52Xu/MaJzRkIk4Q==",
|
||||
"dependencies": {
|
||||
"side-channel": "^1.0.4"
|
||||
},
|
||||
"engines": {
|
||||
"node": ">=0.6"
|
||||
},
|
||||
|
@ -843,12 +914,12 @@
|
|||
}
|
||||
},
|
||||
"node_modules/raw-body": {
|
||||
"version": "2.4.2",
|
||||
"resolved": "https://registry.npmjs.org/raw-body/-/raw-body-2.4.2.tgz",
|
||||
"integrity": "sha512-RPMAFUJP19WIet/99ngh6Iv8fzAbqum4Li7AD6DtGaW2RpMB/11xDoalPiJMTbu6I3hkbMVkATvZrqb9EEqeeQ==",
|
||||
"version": "2.5.1",
|
||||
"resolved": "https://registry.npmjs.org/raw-body/-/raw-body-2.5.1.tgz",
|
||||
"integrity": "sha512-qqJBtEyVgS0ZmPGdCFPWJ3FreoqvG4MVQln/kCgF7Olq95IbOp0/BWyMwbdtn4VTvkM8Y7khCQ2Xgk/tcrCXig==",
|
||||
"dependencies": {
|
||||
"bytes": "3.1.1",
|
||||
"http-errors": "1.8.1",
|
||||
"bytes": "3.1.2",
|
||||
"http-errors": "2.0.0",
|
||||
"iconv-lite": "0.4.24",
|
||||
"unpipe": "1.0.0"
|
||||
},
|
||||
|
@ -892,23 +963,23 @@
|
|||
}
|
||||
},
|
||||
"node_modules/send": {
|
||||
"version": "0.17.2",
|
||||
"resolved": "https://registry.npmjs.org/send/-/send-0.17.2.tgz",
|
||||
"integrity": "sha512-UJYB6wFSJE3G00nEivR5rgWp8c2xXvJ3OPWPhmuteU0IKj8nKbG3DrjiOmLwpnHGYWAVwA69zmTm++YG0Hmwww==",
|
||||
"version": "0.18.0",
|
||||
"resolved": "https://registry.npmjs.org/send/-/send-0.18.0.tgz",
|
||||
"integrity": "sha512-qqWzuOjSFOuqPjFe4NOsMLafToQQwBSOEpS+FwEt3A2V3vKubTquT3vmLTQpFgMXp8AlFWFuP1qKaJZOtPpVXg==",
|
||||
"dependencies": {
|
||||
"debug": "2.6.9",
|
||||
"depd": "~1.1.2",
|
||||
"destroy": "~1.0.4",
|
||||
"depd": "2.0.0",
|
||||
"destroy": "1.2.0",
|
||||
"encodeurl": "~1.0.2",
|
||||
"escape-html": "~1.0.3",
|
||||
"etag": "~1.8.1",
|
||||
"fresh": "0.5.2",
|
||||
"http-errors": "1.8.1",
|
||||
"http-errors": "2.0.0",
|
||||
"mime": "1.6.0",
|
||||
"ms": "2.1.3",
|
||||
"on-finished": "~2.3.0",
|
||||
"on-finished": "2.4.1",
|
||||
"range-parser": "~1.2.1",
|
||||
"statuses": "~1.5.0"
|
||||
"statuses": "2.0.1"
|
||||
},
|
||||
"engines": {
|
||||
"node": ">= 0.8.0"
|
||||
|
@ -920,14 +991,14 @@
|
|||
"integrity": "sha512-6FlzubTLZG3J2a/NVCAleEhjzq5oxgHyaCU9yYXvcLsvoVaHJq/s5xXI6/XXP6tz7R9xAOtHnSO/tXtF3WRTlA=="
|
||||
},
|
||||
"node_modules/serve-static": {
|
||||
"version": "1.14.2",
|
||||
"resolved": "https://registry.npmjs.org/serve-static/-/serve-static-1.14.2.tgz",
|
||||
"integrity": "sha512-+TMNA9AFxUEGuC0z2mevogSnn9MXKb4fa7ngeRMJaaGv8vTwnIEkKi+QGvPt33HSnf8pRS+WGM0EbMtCJLKMBQ==",
|
||||
"version": "1.15.0",
|
||||
"resolved": "https://registry.npmjs.org/serve-static/-/serve-static-1.15.0.tgz",
|
||||
"integrity": "sha512-XGuRDNjXUijsUL0vl6nSD7cwURuzEgglbOaFuZM9g3kwDXOWVTck0jLzjPzGD+TazWbboZYu52/9/XPdUgne9g==",
|
||||
"dependencies": {
|
||||
"encodeurl": "~1.0.2",
|
||||
"escape-html": "~1.0.3",
|
||||
"parseurl": "~1.3.3",
|
||||
"send": "0.17.2"
|
||||
"send": "0.18.0"
|
||||
},
|
||||
"engines": {
|
||||
"node": ">= 0.8.0"
|
||||
|
@ -938,6 +1009,19 @@
|
|||
"resolved": "https://registry.npmjs.org/setprototypeof/-/setprototypeof-1.2.0.tgz",
|
||||
"integrity": "sha512-E5LDX7Wrp85Kil5bhZv46j8jOeboKq5JMmYM3gVGdGH8xFpPWXUMsNrlODCrkoxMEeNi/XZIwuRvY4XNwYMJpw=="
|
||||
},
|
||||
"node_modules/side-channel": {
|
||||
"version": "1.0.4",
|
||||
"resolved": "https://registry.npmjs.org/side-channel/-/side-channel-1.0.4.tgz",
|
||||
"integrity": "sha512-q5XPytqFEIKHkGdiMIrY10mvLRvnQh42/+GoBlFW3b2LXLE2xxJpZFdm94we0BaoV3RwJyGqg5wS7epxTv0Zvw==",
|
||||
"dependencies": {
|
||||
"call-bind": "^1.0.0",
|
||||
"get-intrinsic": "^1.0.2",
|
||||
"object-inspect": "^1.9.0"
|
||||
},
|
||||
"funding": {
|
||||
"url": "https://github.com/sponsors/ljharb"
|
||||
}
|
||||
},
|
||||
"node_modules/source-map": {
|
||||
"version": "0.6.1",
|
||||
"resolved": "https://registry.npmjs.org/source-map/-/source-map-0.6.1.tgz",
|
||||
|
@ -948,11 +1032,11 @@
|
|||
}
|
||||
},
|
||||
"node_modules/statuses": {
|
||||
"version": "1.5.0",
|
||||
"resolved": "https://registry.npmjs.org/statuses/-/statuses-1.5.0.tgz",
|
||||
"integrity": "sha1-Fhx9rBd2Wf2YEfQ3cfqZOBR4Yow=",
|
||||
"version": "2.0.1",
|
||||
"resolved": "https://registry.npmjs.org/statuses/-/statuses-2.0.1.tgz",
|
||||
"integrity": "sha512-RwNA9Z/7PrK06rYLIzFMlaF+l73iwpzsqRIFgbMLbTcLD6cOao82TaWefPXQvB2fOC4AjuYSEndS7N/mTCbkdQ==",
|
||||
"engines": {
|
||||
"node": ">= 0.6"
|
||||
"node": ">= 0.8"
|
||||
}
|
||||
},
|
||||
"node_modules/symbol-tree": {
|
||||
|
@ -1034,7 +1118,7 @@
|
|||
"node_modules/unpipe": {
|
||||
"version": "1.0.0",
|
||||
"resolved": "https://registry.npmjs.org/unpipe/-/unpipe-1.0.0.tgz",
|
||||
"integrity": "sha1-sr9O6FFKrmFltIF4KdIbLvSZBOw=",
|
||||
"integrity": "sha512-pjy2bYhSsufwWlKwPc+l3cN7+wuJlK6uz0YdJEOlQDbl6jo/YlPi4mb8agUkVC8BF7V8NuzeyPNqRksA3hztKQ==",
|
||||
"engines": {
|
||||
"node": ">= 0.8"
|
||||
}
|
||||
|
|
|
@ -1,3 +1,10 @@
|
|||
1.3.8 / 2022-02-02
|
||||
==================
|
||||
|
||||
* deps: mime-types@~2.1.34
|
||||
- deps: mime-db@~1.51.0
|
||||
* deps: negotiator@0.6.3
|
||||
|
||||
1.3.7 / 2019-04-29
|
||||
==================
|
||||
|
||||
|
|
|
@ -3,7 +3,7 @@
|
|||
[![NPM Version][npm-version-image]][npm-url]
|
||||
[![NPM Downloads][npm-downloads-image]][npm-url]
|
||||
[![Node.js Version][node-version-image]][node-version-url]
|
||||
[![Build Status][travis-image]][travis-url]
|
||||
[![Build Status][github-actions-ci-image]][github-actions-ci-url]
|
||||
[![Test Coverage][coveralls-image]][coveralls-url]
|
||||
|
||||
Higher level content negotiation based on [negotiator](https://www.npmjs.com/package/negotiator).
|
||||
|
@ -29,8 +29,6 @@ $ npm install accepts
|
|||
|
||||
## API
|
||||
|
||||
<!-- eslint-disable no-unused-vars -->
|
||||
|
||||
```js
|
||||
var accepts = require('accepts')
|
||||
```
|
||||
|
@ -133,10 +131,10 @@ curl -I -H'Accept: text/html' http://localhost:3000/
|
|||
|
||||
[coveralls-image]: https://badgen.net/coveralls/c/github/jshttp/accepts/master
|
||||
[coveralls-url]: https://coveralls.io/r/jshttp/accepts?branch=master
|
||||
[github-actions-ci-image]: https://badgen.net/github/checks/jshttp/accepts/master?label=ci
|
||||
[github-actions-ci-url]: https://github.com/jshttp/accepts/actions/workflows/ci.yml
|
||||
[node-version-image]: https://badgen.net/npm/node/accepts
|
||||
[node-version-url]: https://nodejs.org/en/download
|
||||
[npm-downloads-image]: https://badgen.net/npm/dm/accepts
|
||||
[npm-url]: https://npmjs.org/package/accepts
|
||||
[npm-version-image]: https://badgen.net/npm/v/accepts
|
||||
[travis-image]: https://badgen.net/travis/jshttp/accepts/master
|
||||
[travis-url]: https://travis-ci.org/jshttp/accepts
|
||||
|
|
|
@ -1,7 +1,7 @@
|
|||
{
|
||||
"name": "accepts",
|
||||
"description": "Higher-level content negotiation",
|
||||
"version": "1.3.7",
|
||||
"version": "1.3.8",
|
||||
"contributors": [
|
||||
"Douglas Christopher Wilson <doug@somethingdoug.com>",
|
||||
"Jonathan Ong <me@jongleberry.com> (http://jongleberry.com)"
|
||||
|
@ -9,20 +9,20 @@
|
|||
"license": "MIT",
|
||||
"repository": "jshttp/accepts",
|
||||
"dependencies": {
|
||||
"mime-types": "~2.1.24",
|
||||
"negotiator": "0.6.2"
|
||||
"mime-types": "~2.1.34",
|
||||
"negotiator": "0.6.3"
|
||||
},
|
||||
"devDependencies": {
|
||||
"deep-equal": "1.0.1",
|
||||
"eslint": "5.16.0",
|
||||
"eslint-config-standard": "12.0.0",
|
||||
"eslint-plugin-import": "2.17.2",
|
||||
"eslint-plugin-markdown": "1.0.0",
|
||||
"eslint-plugin-node": "8.0.1",
|
||||
"eslint-plugin-promise": "4.1.1",
|
||||
"eslint-plugin-standard": "4.0.0",
|
||||
"mocha": "6.1.4",
|
||||
"nyc": "14.0.0"
|
||||
"eslint": "7.32.0",
|
||||
"eslint-config-standard": "14.1.1",
|
||||
"eslint-plugin-import": "2.25.4",
|
||||
"eslint-plugin-markdown": "2.2.1",
|
||||
"eslint-plugin-node": "11.1.0",
|
||||
"eslint-plugin-promise": "4.3.1",
|
||||
"eslint-plugin-standard": "4.1.0",
|
||||
"mocha": "9.2.0",
|
||||
"nyc": "15.1.0"
|
||||
},
|
||||
"files": [
|
||||
"LICENSE",
|
||||
|
@ -33,10 +33,10 @@
|
|||
"node": ">= 0.6"
|
||||
},
|
||||
"scripts": {
|
||||
"lint": "eslint --plugin markdown --ext js,md .",
|
||||
"lint": "eslint .",
|
||||
"test": "mocha --reporter spec --check-leaks --bail test/",
|
||||
"test-cov": "nyc --reporter=html --reporter=text npm test",
|
||||
"test-travis": "nyc --reporter=text npm test"
|
||||
"test-ci": "nyc --reporter=lcov --reporter=text npm test",
|
||||
"test-cov": "nyc --reporter=html --reporter=text npm test"
|
||||
},
|
||||
"keywords": [
|
||||
"content",
|
||||
|
|
|
@ -1,3 +1,36 @@
|
|||
1.20.1 / 2022-10-06
|
||||
===================
|
||||
|
||||
* deps: qs@6.11.0
|
||||
* perf: remove unnecessary object clone
|
||||
|
||||
1.20.0 / 2022-04-02
|
||||
===================
|
||||
|
||||
* Fix error message for json parse whitespace in `strict`
|
||||
* Fix internal error when inflated body exceeds limit
|
||||
* Prevent loss of async hooks context
|
||||
* Prevent hanging when request already read
|
||||
* deps: depd@2.0.0
|
||||
- Replace internal `eval` usage with `Function` constructor
|
||||
- Use instance methods on `process` to check for listeners
|
||||
* deps: http-errors@2.0.0
|
||||
- deps: depd@2.0.0
|
||||
- deps: statuses@2.0.1
|
||||
* deps: on-finished@2.4.1
|
||||
* deps: qs@6.10.3
|
||||
* deps: raw-body@2.5.1
|
||||
- deps: http-errors@2.0.0
|
||||
|
||||
1.19.2 / 2022-02-15
|
||||
===================
|
||||
|
||||
* deps: bytes@3.1.2
|
||||
* deps: qs@6.9.7
|
||||
* Fix handling of `__proto__` keys
|
||||
* deps: raw-body@2.4.3
|
||||
- deps: bytes@3.1.2
|
||||
|
||||
1.19.1 / 2021-12-10
|
||||
===================
|
||||
|
||||
|
|
|
@ -342,6 +342,14 @@ to this middleware. This module operates directly on bytes only and you cannot
|
|||
call `req.setEncoding` when using this module. The `status` property is set to
|
||||
`500` and the `type` property is set to `'stream.encoding.set'`.
|
||||
|
||||
### stream is not readable
|
||||
|
||||
This error will occur when the request is no longer readable when this middleware
|
||||
attempts to read it. This typically means something other than a middleware from
|
||||
this module read the request body already and the middleware was also configured to
|
||||
read the same request. The `status` property is set to `500` and the `type`
|
||||
property is set to `'stream.not.readable'`.
|
||||
|
||||
### too many parameters
|
||||
|
||||
This error will occur when the content of the request exceeds the configured
|
||||
|
@ -453,4 +461,4 @@ app.use(bodyParser.text({ type: 'text/html' }))
|
|||
[downloads-image]: https://img.shields.io/npm/dm/body-parser.svg
|
||||
[downloads-url]: https://npmjs.org/package/body-parser
|
||||
[github-actions-ci-image]: https://img.shields.io/github/workflow/status/expressjs/body-parser/ci/master?label=ci
|
||||
[github-actions-ci-url]: https://github.com/expressjs/body-parser?query=workflow%3Aci
|
||||
[github-actions-ci-url]: https://github.com/expressjs/body-parser/actions/workflows/ci.yml
|
||||
|
|
|
@ -0,0 +1,25 @@
|
|||
# Security Policies and Procedures
|
||||
|
||||
## Reporting a Bug
|
||||
|
||||
The Express team and community take all security bugs seriously. Thank you
|
||||
for improving the security of Express. We appreciate your efforts and
|
||||
responsible disclosure and will make every effort to acknowledge your
|
||||
contributions.
|
||||
|
||||
Report security bugs by emailing the current owner(s) of `body-parser`. This
|
||||
information can be found in the npm registry using the command
|
||||
`npm owner ls body-parser`.
|
||||
If unsure or unable to get the information from the above, open an issue
|
||||
in the [project issue tracker](https://github.com/expressjs/body-parser/issues)
|
||||
asking for the current contact information.
|
||||
|
||||
To ensure the timely response to your report, please ensure that the entirety
|
||||
of the report is contained within the email body and not solely behind a web
|
||||
link or an attachment.
|
||||
|
||||
At least one owner will acknowledge your email within 48 hours, and will send a
|
||||
more detailed response within 48 hours indicating the next steps in handling
|
||||
your report. After the initial reply to your report, the owners will
|
||||
endeavor to keep you informed of the progress towards a fix and full
|
||||
announcement, and may ask for additional information or guidance.
|
|
@ -91,16 +91,15 @@ Object.defineProperty(exports, 'urlencoded', {
|
|||
*/
|
||||
|
||||
function bodyParser (options) {
|
||||
var opts = {}
|
||||
|
||||
// exclude type option
|
||||
if (options) {
|
||||
for (var prop in options) {
|
||||
if (prop !== 'type') {
|
||||
opts[prop] = options[prop]
|
||||
}
|
||||
// use default type for parsers
|
||||
var opts = Object.create(options || null, {
|
||||
type: {
|
||||
configurable: true,
|
||||
enumerable: true,
|
||||
value: undefined,
|
||||
writable: true
|
||||
}
|
||||
}
|
||||
})
|
||||
|
||||
var _urlencoded = exports.urlencoded(opts)
|
||||
var _json = exports.json(opts)
|
||||
|
|
|
@ -12,9 +12,11 @@
|
|||
*/
|
||||
|
||||
var createError = require('http-errors')
|
||||
var destroy = require('destroy')
|
||||
var getBody = require('raw-body')
|
||||
var iconv = require('iconv-lite')
|
||||
var onFinished = require('on-finished')
|
||||
var unpipe = require('unpipe')
|
||||
var zlib = require('zlib')
|
||||
|
||||
/**
|
||||
|
@ -89,9 +91,14 @@ function read (req, res, next, parse, debug, options) {
|
|||
_error = createError(400, error)
|
||||
}
|
||||
|
||||
// unpipe from stream and destroy
|
||||
if (stream !== req) {
|
||||
unpipe(req)
|
||||
destroy(stream, true)
|
||||
}
|
||||
|
||||
// read off entire request
|
||||
stream.resume()
|
||||
onFinished(req, function onfinished () {
|
||||
dump(req, function onfinished () {
|
||||
next(createError(400, _error))
|
||||
})
|
||||
return
|
||||
|
@ -179,3 +186,20 @@ function contentstream (req, debug, inflate) {
|
|||
|
||||
return stream
|
||||
}
|
||||
|
||||
/**
|
||||
* Dump the contents of a request.
|
||||
*
|
||||
* @param {object} req
|
||||
* @param {function} callback
|
||||
* @api private
|
||||
*/
|
||||
|
||||
function dump (req, callback) {
|
||||
if (onFinished.isFinished(req)) {
|
||||
callback(null)
|
||||
} else {
|
||||
onFinished(req, callback)
|
||||
req.resume()
|
||||
}
|
||||
}
|
||||
|
|
|
@ -37,7 +37,7 @@ module.exports = json
|
|||
* %x0D ) ; Carriage return
|
||||
*/
|
||||
|
||||
var FIRST_CHAR_REGEXP = /^[\x20\x09\x0a\x0d]*(.)/ // eslint-disable-line no-control-regex
|
||||
var FIRST_CHAR_REGEXP = /^[\x20\x09\x0a\x0d]*([^\x20\x09\x0a\x0d])/ // eslint-disable-line no-control-regex
|
||||
|
||||
/**
|
||||
* Create a middleware to parse JSON bodies.
|
||||
|
@ -122,7 +122,7 @@ function json (options) {
|
|||
|
||||
// assert charset per RFC 7159 sec 8.1
|
||||
var charset = getCharset(req) || 'utf-8'
|
||||
if (charset.substr(0, 4) !== 'utf-') {
|
||||
if (charset.slice(0, 4) !== 'utf-') {
|
||||
debug('invalid charset')
|
||||
next(createError(415, 'unsupported charset "' + charset.toUpperCase() + '"', {
|
||||
charset: charset,
|
||||
|
@ -152,7 +152,9 @@ function json (options) {
|
|||
|
||||
function createStrictSyntaxError (str, char) {
|
||||
var index = str.indexOf(char)
|
||||
var partial = str.substring(0, index) + '#'
|
||||
var partial = index !== -1
|
||||
? str.substring(0, index) + '#'
|
||||
: ''
|
||||
|
||||
try {
|
||||
JSON.parse(partial); /* istanbul ignore next */ throw new SyntaxError('strict violation')
|
||||
|
@ -173,7 +175,11 @@ function createStrictSyntaxError (str, char) {
|
|||
*/
|
||||
|
||||
function firstchar (str) {
|
||||
return FIRST_CHAR_REGEXP.exec(str)[1]
|
||||
var match = FIRST_CHAR_REGEXP.exec(str)
|
||||
|
||||
return match
|
||||
? match[1]
|
||||
: undefined
|
||||
}
|
||||
|
||||
/**
|
||||
|
|
|
@ -1,7 +1,7 @@
|
|||
{
|
||||
"name": "body-parser",
|
||||
"description": "Node.js body parsing middleware",
|
||||
"version": "1.19.1",
|
||||
"version": "1.20.1",
|
||||
"contributors": [
|
||||
"Douglas Christopher Wilson <doug@somethingdoug.com>",
|
||||
"Jonathan Ong <me@jongleberry.com> (http://jongleberry.com)"
|
||||
|
@ -9,39 +9,43 @@
|
|||
"license": "MIT",
|
||||
"repository": "expressjs/body-parser",
|
||||
"dependencies": {
|
||||
"bytes": "3.1.1",
|
||||
"bytes": "3.1.2",
|
||||
"content-type": "~1.0.4",
|
||||
"debug": "2.6.9",
|
||||
"depd": "~1.1.2",
|
||||
"http-errors": "1.8.1",
|
||||
"depd": "2.0.0",
|
||||
"destroy": "1.2.0",
|
||||
"http-errors": "2.0.0",
|
||||
"iconv-lite": "0.4.24",
|
||||
"on-finished": "~2.3.0",
|
||||
"qs": "6.9.6",
|
||||
"raw-body": "2.4.2",
|
||||
"type-is": "~1.6.18"
|
||||
"on-finished": "2.4.1",
|
||||
"qs": "6.11.0",
|
||||
"raw-body": "2.5.1",
|
||||
"type-is": "~1.6.18",
|
||||
"unpipe": "1.0.0"
|
||||
},
|
||||
"devDependencies": {
|
||||
"eslint": "7.32.0",
|
||||
"eslint": "8.24.0",
|
||||
"eslint-config-standard": "14.1.1",
|
||||
"eslint-plugin-import": "2.25.3",
|
||||
"eslint-plugin-markdown": "2.2.1",
|
||||
"eslint-plugin-import": "2.26.0",
|
||||
"eslint-plugin-markdown": "3.0.0",
|
||||
"eslint-plugin-node": "11.1.0",
|
||||
"eslint-plugin-promise": "5.2.0",
|
||||
"eslint-plugin-promise": "6.0.1",
|
||||
"eslint-plugin-standard": "4.1.0",
|
||||
"methods": "1.1.2",
|
||||
"mocha": "9.1.3",
|
||||
"mocha": "10.0.0",
|
||||
"nyc": "15.1.0",
|
||||
"safe-buffer": "5.2.1",
|
||||
"supertest": "6.1.6"
|
||||
"supertest": "6.3.0"
|
||||
},
|
||||
"files": [
|
||||
"lib/",
|
||||
"LICENSE",
|
||||
"HISTORY.md",
|
||||
"SECURITY.md",
|
||||
"index.js"
|
||||
],
|
||||
"engines": {
|
||||
"node": ">= 0.8"
|
||||
"node": ">= 0.8",
|
||||
"npm": "1.2.8000 || >= 1.4.16"
|
||||
},
|
||||
"scripts": {
|
||||
"lint": "eslint .",
|
||||
|
|
|
@ -1,3 +1,8 @@
|
|||
3.1.2 / 2022-01-27
|
||||
==================
|
||||
|
||||
* Fix return value for un-parsable strings
|
||||
|
||||
3.1.1 / 2021-11-15
|
||||
==================
|
||||
|
||||
|
|
|
@ -162,5 +162,9 @@ function parse(val) {
|
|||
unit = results[4].toLowerCase();
|
||||
}
|
||||
|
||||
if (isNaN(floatValue)) {
|
||||
return null;
|
||||
}
|
||||
|
||||
return Math.floor(map[unit] * floatValue);
|
||||
}
|
||||
|
|
|
@ -1,7 +1,7 @@
|
|||
{
|
||||
"name": "bytes",
|
||||
"description": "Utility to parse a string bytes to bytes and vice-versa",
|
||||
"version": "3.1.1",
|
||||
"version": "3.1.2",
|
||||
"author": "TJ Holowaychuk <tj@vision-media.ca> (http://tjholowaychuk.com)",
|
||||
"contributors": [
|
||||
"Jed Watson <jed.watson@me.com>",
|
||||
|
@ -21,7 +21,7 @@
|
|||
"devDependencies": {
|
||||
"eslint": "7.32.0",
|
||||
"eslint-plugin-markdown": "2.2.1",
|
||||
"mocha": "9.1.3",
|
||||
"mocha": "9.2.0",
|
||||
"nyc": "15.1.0"
|
||||
},
|
||||
"files": [
|
||||
|
|
|
@ -1,2 +1 @@
|
|||
dist/
|
||||
coverage/
|
|
@ -0,0 +1,17 @@
|
|||
{
|
||||
"root": true,
|
||||
|
||||
"extends": "@ljharb",
|
||||
|
||||
"rules": {
|
||||
"func-name-matching": 0,
|
||||
"id-length": 0,
|
||||
"new-cap": [2, {
|
||||
"capIsNewExceptions": [
|
||||
"GetIntrinsic",
|
||||
],
|
||||
}],
|
||||
"no-magic-numbers": 0,
|
||||
"operator-linebreak": [2, "before"],
|
||||
},
|
||||
}
|
|
@ -0,0 +1,12 @@
|
|||
# These are supported funding model platforms
|
||||
|
||||
github: [ljharb]
|
||||
patreon: # Replace with a single Patreon username
|
||||
open_collective: # Replace with a single Open Collective username
|
||||
ko_fi: # Replace with a single Ko-fi username
|
||||
tidelift: npm/call-bind
|
||||
community_bridge: # Replace with a single Community Bridge project-name e.g., cloud-foundry
|
||||
liberapay: # Replace with a single Liberapay username
|
||||
issuehunt: # Replace with a single IssueHunt username
|
||||
otechie: # Replace with a single Otechie username
|
||||
custom: # Replace with up to 4 custom sponsorship URLs e.g., ['link1', 'link2']
|
|
@ -0,0 +1,13 @@
|
|||
{
|
||||
"all": true,
|
||||
"check-coverage": false,
|
||||
"reporter": ["text-summary", "text", "html", "json"],
|
||||
"lines": 86,
|
||||
"statements": 85.93,
|
||||
"functions": 82.43,
|
||||
"branches": 76.06,
|
||||
"exclude": [
|
||||
"coverage",
|
||||
"test"
|
||||
]
|
||||
}
|
|
@ -0,0 +1,42 @@
|
|||
# Changelog
|
||||
|
||||
All notable changes to this project will be documented in this file.
|
||||
|
||||
The format is based on [Keep a Changelog](https://keepachangelog.com/en/1.0.0/)
|
||||
and this project adheres to [Semantic Versioning](https://semver.org/spec/v2.0.0.html).
|
||||
|
||||
## [v1.0.2](https://github.com/ljharb/call-bind/compare/v1.0.1...v1.0.2) - 2021-01-11
|
||||
|
||||
### Commits
|
||||
|
||||
- [Fix] properly include the receiver in the bound length [`dbae7bc`](https://github.com/ljharb/call-bind/commit/dbae7bc676c079a0d33c0a43e9ef92cb7b01345d)
|
||||
|
||||
## [v1.0.1](https://github.com/ljharb/call-bind/compare/v1.0.0...v1.0.1) - 2021-01-08
|
||||
|
||||
### Commits
|
||||
|
||||
- [Tests] migrate tests to Github Actions [`b6db284`](https://github.com/ljharb/call-bind/commit/b6db284c36f8ccd195b88a6764fe84b7223a0da1)
|
||||
- [meta] do not publish github action workflow files [`ec7fe46`](https://github.com/ljharb/call-bind/commit/ec7fe46e60cfa4764ee943d2755f5e5a366e578e)
|
||||
- [Fix] preserve original function’s length when possible [`adbceaa`](https://github.com/ljharb/call-bind/commit/adbceaa3cac4b41ea78bb19d7ccdbaaf7e0bdadb)
|
||||
- [Tests] gather coverage data on every job [`d69e23c`](https://github.com/ljharb/call-bind/commit/d69e23cc65f101ba1d4c19bb07fa8eb0ec624be8)
|
||||
- [Dev Deps] update `eslint`, `@ljharb/eslint-config`, `aud`, `tape` [`2fd3586`](https://github.com/ljharb/call-bind/commit/2fd3586c5d47b335364c14293114c6b625ae1f71)
|
||||
- [Deps] update `get-intrinsic` [`f23e931`](https://github.com/ljharb/call-bind/commit/f23e9318cc271c2add8bb38cfded85ee7baf8eee)
|
||||
- [Deps] update `get-intrinsic` [`72d9f44`](https://github.com/ljharb/call-bind/commit/72d9f44e184465ba8dd3fb48260bbcff234985f2)
|
||||
- [meta] fix FUNDING.yml [`e723573`](https://github.com/ljharb/call-bind/commit/e723573438c5a68dcec31fb5d96ea6b7e4a93be8)
|
||||
- [eslint] ignore coverage output [`15e76d2`](https://github.com/ljharb/call-bind/commit/15e76d28a5f43e504696401e5b31ebb78ee1b532)
|
||||
- [meta] add Automatic Rebase and Require Allow Edits workflows [`8fa4dab`](https://github.com/ljharb/call-bind/commit/8fa4dabb23ba3dd7bb92c9571c1241c08b56e4b6)
|
||||
|
||||
## v1.0.0 - 2020-10-30
|
||||
|
||||
### Commits
|
||||
|
||||
- Initial commit [`306cf98`](https://github.com/ljharb/call-bind/commit/306cf98c7ec9e7ef66b653ec152277ac1381eb50)
|
||||
- Tests [`e10d0bb`](https://github.com/ljharb/call-bind/commit/e10d0bbdadc7a10ecedc9a1c035112d3e368b8df)
|
||||
- Implementation [`43852ed`](https://github.com/ljharb/call-bind/commit/43852eda0f187327b7fad2423ca972149a52bd65)
|
||||
- npm init [`408f860`](https://github.com/ljharb/call-bind/commit/408f860b773a2f610805fd3613d0d71bac1b6249)
|
||||
- [meta] add Automatic Rebase and Require Allow Edits workflows [`fb349b2`](https://github.com/ljharb/call-bind/commit/fb349b2e48defbec8b5ec8a8395cc8f69f220b13)
|
||||
- [meta] add `auto-changelog` [`c4001fc`](https://github.com/ljharb/call-bind/commit/c4001fc43031799ef908211c98d3b0fb2b60fde4)
|
||||
- [meta] add "funding"; create `FUNDING.yml` [`d4d6d29`](https://github.com/ljharb/call-bind/commit/d4d6d2974a14bc2e98830468eda7fe6d6a776717)
|
||||
- [Tests] add `npm run lint` [`dedfb98`](https://github.com/ljharb/call-bind/commit/dedfb98bd0ecefb08ddb9a94061bd10cde4332af)
|
||||
- Only apps should have lockfiles [`54ac776`](https://github.com/ljharb/call-bind/commit/54ac77653db45a7361dc153d2f478e743f110650)
|
||||
- [meta] add `safe-publish-latest` [`9ea8e43`](https://github.com/ljharb/call-bind/commit/9ea8e435b950ce9b705559cd651039f9bf40140f)
|
|
@ -0,0 +1,21 @@
|
|||
MIT License
|
||||
|
||||
Copyright (c) 2020 Jordan Harband
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
of this software and associated documentation files (the "Software"), to deal
|
||||
in the Software without restriction, including without limitation the rights
|
||||
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
copies of the Software, and to permit persons to whom the Software is
|
||||
furnished to do so, subject to the following conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be included in all
|
||||
copies or substantial portions of the Software.
|
||||
|
||||
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
SOFTWARE.
|
|
@ -0,0 +1,2 @@
|
|||
# call-bind
|
||||
Robustly `.call.bind()` a function.
|
|
@ -0,0 +1,15 @@
|
|||
'use strict';
|
||||
|
||||
var GetIntrinsic = require('get-intrinsic');
|
||||
|
||||
var callBind = require('./');
|
||||
|
||||
var $indexOf = callBind(GetIntrinsic('String.prototype.indexOf'));
|
||||
|
||||
module.exports = function callBoundIntrinsic(name, allowMissing) {
|
||||
var intrinsic = GetIntrinsic(name, !!allowMissing);
|
||||
if (typeof intrinsic === 'function' && $indexOf(name, '.prototype.') > -1) {
|
||||
return callBind(intrinsic);
|
||||
}
|
||||
return intrinsic;
|
||||
};
|
|
@ -0,0 +1,47 @@
|
|||
'use strict';
|
||||
|
||||
var bind = require('function-bind');
|
||||
var GetIntrinsic = require('get-intrinsic');
|
||||
|
||||
var $apply = GetIntrinsic('%Function.prototype.apply%');
|
||||
var $call = GetIntrinsic('%Function.prototype.call%');
|
||||
var $reflectApply = GetIntrinsic('%Reflect.apply%', true) || bind.call($call, $apply);
|
||||
|
||||
var $gOPD = GetIntrinsic('%Object.getOwnPropertyDescriptor%', true);
|
||||
var $defineProperty = GetIntrinsic('%Object.defineProperty%', true);
|
||||
var $max = GetIntrinsic('%Math.max%');
|
||||
|
||||
if ($defineProperty) {
|
||||
try {
|
||||
$defineProperty({}, 'a', { value: 1 });
|
||||
} catch (e) {
|
||||
// IE 8 has a broken defineProperty
|
||||
$defineProperty = null;
|
||||
}
|
||||
}
|
||||
|
||||
module.exports = function callBind(originalFunction) {
|
||||
var func = $reflectApply(bind, $call, arguments);
|
||||
if ($gOPD && $defineProperty) {
|
||||
var desc = $gOPD(func, 'length');
|
||||
if (desc.configurable) {
|
||||
// original length, plus the receiver, minus any additional arguments (after the receiver)
|
||||
$defineProperty(
|
||||
func,
|
||||
'length',
|
||||
{ value: 1 + $max(0, originalFunction.length - (arguments.length - 1)) }
|
||||
);
|
||||
}
|
||||
}
|
||||
return func;
|
||||
};
|
||||
|
||||
var applyBind = function applyBind() {
|
||||
return $reflectApply(bind, $apply, arguments);
|
||||
};
|
||||
|
||||
if ($defineProperty) {
|
||||
$defineProperty(module.exports, 'apply', { value: applyBind });
|
||||
} else {
|
||||
module.exports.apply = applyBind;
|
||||
}
|
|
@ -0,0 +1,80 @@
|
|||
{
|
||||
"name": "call-bind",
|
||||
"version": "1.0.2",
|
||||
"description": "Robustly `.call.bind()` a function",
|
||||
"main": "index.js",
|
||||
"exports": {
|
||||
".": [
|
||||
{
|
||||
"default": "./index.js"
|
||||
},
|
||||
"./index.js"
|
||||
],
|
||||
"./callBound": [
|
||||
{
|
||||
"default": "./callBound.js"
|
||||
},
|
||||
"./callBound.js"
|
||||
],
|
||||
"./package.json": "./package.json"
|
||||
},
|
||||
"scripts": {
|
||||
"prepublish": "safe-publish-latest",
|
||||
"lint": "eslint --ext=.js,.mjs .",
|
||||
"pretest": "npm run lint",
|
||||
"tests-only": "nyc tape 'test/*'",
|
||||
"test": "npm run tests-only",
|
||||
"posttest": "aud --production",
|
||||
"version": "auto-changelog && git add CHANGELOG.md",
|
||||
"postversion": "auto-changelog && git add CHANGELOG.md && git commit --no-edit --amend && git tag -f \"v$(node -e \"console.log(require('./package.json').version)\")\""
|
||||
},
|
||||
"repository": {
|
||||
"type": "git",
|
||||
"url": "git+https://github.com/ljharb/call-bind.git"
|
||||
},
|
||||
"keywords": [
|
||||
"javascript",
|
||||
"ecmascript",
|
||||
"es",
|
||||
"js",
|
||||
"callbind",
|
||||
"callbound",
|
||||
"call",
|
||||
"bind",
|
||||
"bound",
|
||||
"call-bind",
|
||||
"call-bound",
|
||||
"function",
|
||||
"es-abstract"
|
||||
],
|
||||
"author": "Jordan Harband <ljharb@gmail.com>",
|
||||
"funding": {
|
||||
"url": "https://github.com/sponsors/ljharb"
|
||||
},
|
||||
"license": "MIT",
|
||||
"bugs": {
|
||||
"url": "https://github.com/ljharb/call-bind/issues"
|
||||
},
|
||||
"homepage": "https://github.com/ljharb/call-bind#readme",
|
||||
"devDependencies": {
|
||||
"@ljharb/eslint-config": "^17.3.0",
|
||||
"aud": "^1.1.3",
|
||||
"auto-changelog": "^2.2.1",
|
||||
"eslint": "^7.17.0",
|
||||
"nyc": "^10.3.2",
|
||||
"safe-publish-latest": "^1.1.4",
|
||||
"tape": "^5.1.1"
|
||||
},
|
||||
"dependencies": {
|
||||
"function-bind": "^1.1.1",
|
||||
"get-intrinsic": "^1.0.2"
|
||||
},
|
||||
"auto-changelog": {
|
||||
"output": "CHANGELOG.md",
|
||||
"template": "keepachangelog",
|
||||
"unreleased": false,
|
||||
"commitLimit": false,
|
||||
"backfillLimit": false,
|
||||
"hideCredit": true
|
||||
}
|
||||
}
|
|
@ -0,0 +1,55 @@
|
|||
'use strict';
|
||||
|
||||
var test = require('tape');
|
||||
|
||||
var callBound = require('../callBound');
|
||||
|
||||
test('callBound', function (t) {
|
||||
// static primitive
|
||||
t.equal(callBound('Array.length'), Array.length, 'Array.length yields itself');
|
||||
t.equal(callBound('%Array.length%'), Array.length, '%Array.length% yields itself');
|
||||
|
||||
// static non-function object
|
||||
t.equal(callBound('Array.prototype'), Array.prototype, 'Array.prototype yields itself');
|
||||
t.equal(callBound('%Array.prototype%'), Array.prototype, '%Array.prototype% yields itself');
|
||||
t.equal(callBound('Array.constructor'), Array.constructor, 'Array.constructor yields itself');
|
||||
t.equal(callBound('%Array.constructor%'), Array.constructor, '%Array.constructor% yields itself');
|
||||
|
||||
// static function
|
||||
t.equal(callBound('Date.parse'), Date.parse, 'Date.parse yields itself');
|
||||
t.equal(callBound('%Date.parse%'), Date.parse, '%Date.parse% yields itself');
|
||||
|
||||
// prototype primitive
|
||||
t.equal(callBound('Error.prototype.message'), Error.prototype.message, 'Error.prototype.message yields itself');
|
||||
t.equal(callBound('%Error.prototype.message%'), Error.prototype.message, '%Error.prototype.message% yields itself');
|
||||
|
||||
// prototype function
|
||||
t.notEqual(callBound('Object.prototype.toString'), Object.prototype.toString, 'Object.prototype.toString does not yield itself');
|
||||
t.notEqual(callBound('%Object.prototype.toString%'), Object.prototype.toString, '%Object.prototype.toString% does not yield itself');
|
||||
t.equal(callBound('Object.prototype.toString')(true), Object.prototype.toString.call(true), 'call-bound Object.prototype.toString calls into the original');
|
||||
t.equal(callBound('%Object.prototype.toString%')(true), Object.prototype.toString.call(true), 'call-bound %Object.prototype.toString% calls into the original');
|
||||
|
||||
t['throws'](
|
||||
function () { callBound('does not exist'); },
|
||||
SyntaxError,
|
||||
'nonexistent intrinsic throws'
|
||||
);
|
||||
t['throws'](
|
||||
function () { callBound('does not exist', true); },
|
||||
SyntaxError,
|
||||
'allowMissing arg still throws for unknown intrinsic'
|
||||
);
|
||||
|
||||
/* globals WeakRef: false */
|
||||
t.test('real but absent intrinsic', { skip: typeof WeakRef !== 'undefined' }, function (st) {
|
||||
st['throws'](
|
||||
function () { callBound('WeakRef'); },
|
||||
TypeError,
|
||||
'real but absent intrinsic throws'
|
||||
);
|
||||
st.equal(callBound('WeakRef', true), undefined, 'allowMissing arg avoids exception');
|
||||
st.end();
|
||||
});
|
||||
|
||||
t.end();
|
||||
});
|
|
@ -0,0 +1,66 @@
|
|||
'use strict';
|
||||
|
||||
var callBind = require('../');
|
||||
var bind = require('function-bind');
|
||||
|
||||
var test = require('tape');
|
||||
|
||||
/*
|
||||
* older engines have length nonconfigurable
|
||||
* in io.js v3, it is configurable except on bound functions, hence the .bind()
|
||||
*/
|
||||
var functionsHaveConfigurableLengths = !!(
|
||||
Object.getOwnPropertyDescriptor
|
||||
&& Object.getOwnPropertyDescriptor(bind.call(function () {}), 'length').configurable
|
||||
);
|
||||
|
||||
test('callBind', function (t) {
|
||||
var sentinel = { sentinel: true };
|
||||
var func = function (a, b) {
|
||||
// eslint-disable-next-line no-invalid-this
|
||||
return [this, a, b];
|
||||
};
|
||||
t.equal(func.length, 2, 'original function length is 2');
|
||||
t.deepEqual(func(), [undefined, undefined, undefined], 'unbound func with too few args');
|
||||
t.deepEqual(func(1, 2), [undefined, 1, 2], 'unbound func with right args');
|
||||
t.deepEqual(func(1, 2, 3), [undefined, 1, 2], 'unbound func with too many args');
|
||||
|
||||
var bound = callBind(func);
|
||||
t.equal(bound.length, func.length + 1, 'function length is preserved', { skip: !functionsHaveConfigurableLengths });
|
||||
t.deepEqual(bound(), [undefined, undefined, undefined], 'bound func with too few args');
|
||||
t.deepEqual(bound(1, 2), [1, 2, undefined], 'bound func with right args');
|
||||
t.deepEqual(bound(1, 2, 3), [1, 2, 3], 'bound func with too many args');
|
||||
|
||||
var boundR = callBind(func, sentinel);
|
||||
t.equal(boundR.length, func.length, 'function length is preserved', { skip: !functionsHaveConfigurableLengths });
|
||||
t.deepEqual(boundR(), [sentinel, undefined, undefined], 'bound func with receiver, with too few args');
|
||||
t.deepEqual(boundR(1, 2), [sentinel, 1, 2], 'bound func with receiver, with right args');
|
||||
t.deepEqual(boundR(1, 2, 3), [sentinel, 1, 2], 'bound func with receiver, with too many args');
|
||||
|
||||
var boundArg = callBind(func, sentinel, 1);
|
||||
t.equal(boundArg.length, func.length - 1, 'function length is preserved', { skip: !functionsHaveConfigurableLengths });
|
||||
t.deepEqual(boundArg(), [sentinel, 1, undefined], 'bound func with receiver and arg, with too few args');
|
||||
t.deepEqual(boundArg(2), [sentinel, 1, 2], 'bound func with receiver and arg, with right arg');
|
||||
t.deepEqual(boundArg(2, 3), [sentinel, 1, 2], 'bound func with receiver and arg, with too many args');
|
||||
|
||||
t.test('callBind.apply', function (st) {
|
||||
var aBound = callBind.apply(func);
|
||||
st.deepEqual(aBound(sentinel), [sentinel, undefined, undefined], 'apply-bound func with no args');
|
||||
st.deepEqual(aBound(sentinel, [1], 4), [sentinel, 1, undefined], 'apply-bound func with too few args');
|
||||
st.deepEqual(aBound(sentinel, [1, 2], 4), [sentinel, 1, 2], 'apply-bound func with right args');
|
||||
|
||||
var aBoundArg = callBind.apply(func);
|
||||
st.deepEqual(aBoundArg(sentinel, [1, 2, 3], 4), [sentinel, 1, 2], 'apply-bound func with too many args');
|
||||
st.deepEqual(aBoundArg(sentinel, [1, 2], 4), [sentinel, 1, 2], 'apply-bound func with right args');
|
||||
st.deepEqual(aBoundArg(sentinel, [1], 4), [sentinel, 1, undefined], 'apply-bound func with too few args');
|
||||
|
||||
var aBoundR = callBind.apply(func, sentinel);
|
||||
st.deepEqual(aBoundR([1, 2, 3], 4), [sentinel, 1, 2], 'apply-bound func with receiver and too many args');
|
||||
st.deepEqual(aBoundR([1, 2], 4), [sentinel, 1, 2], 'apply-bound func with receiver and right args');
|
||||
st.deepEqual(aBoundR([1], 4), [sentinel, 1, undefined], 'apply-bound func with receiver and too few args');
|
||||
|
||||
st.end();
|
||||
});
|
||||
|
||||
t.end();
|
||||
});
|
|
@ -1,3 +1,17 @@
|
|||
0.5.0 / 2022-04-11
|
||||
==================
|
||||
|
||||
* Add `priority` option
|
||||
* Fix `expires` option to reject invalid dates
|
||||
* pref: improve default decode speed
|
||||
* pref: remove slow string split in parse
|
||||
|
||||
0.4.2 / 2022-02-02
|
||||
==================
|
||||
|
||||
* pref: read value only when assigning in parse
|
||||
* pref: remove unnecessary regexp in parse
|
||||
|
||||
0.4.1 / 2020-04-21
|
||||
==================
|
||||
|
||||
|
|
|
@ -3,7 +3,7 @@
|
|||
[![NPM Version][npm-version-image]][npm-url]
|
||||
[![NPM Downloads][npm-downloads-image]][npm-url]
|
||||
[![Node.js Version][node-version-image]][node-version-url]
|
||||
[![Build Status][travis-image]][travis-url]
|
||||
[![Build Status][github-actions-ci-image]][github-actions-ci-url]
|
||||
[![Test Coverage][coveralls-image]][coveralls-url]
|
||||
|
||||
Basic HTTP cookie parser and serializer for HTTP servers.
|
||||
|
@ -112,9 +112,23 @@ so if both are set, they should point to the same date and time.
|
|||
Specifies the value for the [`Path` `Set-Cookie` attribute][rfc-6265-5.2.4]. By default, the path
|
||||
is considered the ["default path"][rfc-6265-5.1.4].
|
||||
|
||||
##### priority
|
||||
|
||||
Specifies the `string` to be the value for the [`Priority` `Set-Cookie` attribute][rfc-west-cookie-priority-00-4.1].
|
||||
|
||||
- `'low'` will set the `Priority` attribute to `Low`.
|
||||
- `'medium'` will set the `Priority` attribute to `Medium`, the default priority when not set.
|
||||
- `'high'` will set the `Priority` attribute to `High`.
|
||||
|
||||
More information about the different priority levels can be found in
|
||||
[the specification][rfc-west-cookie-priority-00-4.1].
|
||||
|
||||
**note** This is an attribute that has not yet been fully standardized, and may change in the future.
|
||||
This also means many clients may ignore this attribute until they understand it.
|
||||
|
||||
##### sameSite
|
||||
|
||||
Specifies the `boolean` or `string` to be the value for the [`SameSite` `Set-Cookie` attribute][rfc-6265bis-03-4.1.2.7].
|
||||
Specifies the `boolean` or `string` to be the value for the [`SameSite` `Set-Cookie` attribute][rfc-6265bis-09-5.4.7].
|
||||
|
||||
- `true` will set the `SameSite` attribute to `Strict` for strict same site enforcement.
|
||||
- `false` will not set the `SameSite` attribute.
|
||||
|
@ -123,7 +137,7 @@ Specifies the `boolean` or `string` to be the value for the [`SameSite` `Set-Coo
|
|||
- `'strict'` will set the `SameSite` attribute to `Strict` for strict same site enforcement.
|
||||
|
||||
More information about the different enforcement levels can be found in
|
||||
[the specification][rfc-6265bis-03-4.1.2.7].
|
||||
[the specification][rfc-6265bis-09-5.4.7].
|
||||
|
||||
**note** This is an attribute that has not yet been fully standardized, and may change in the future.
|
||||
This also means many clients may ignore this attribute until they understand it.
|
||||
|
@ -198,40 +212,71 @@ $ npm test
|
|||
```
|
||||
$ npm run bench
|
||||
|
||||
> cookie@0.3.1 bench cookie
|
||||
> cookie@0.4.2 bench
|
||||
> node benchmark/index.js
|
||||
|
||||
http_parser@2.8.0
|
||||
node@6.14.2
|
||||
v8@5.1.281.111
|
||||
uv@1.16.1
|
||||
node@16.14.0
|
||||
v8@9.4.146.24-node.20
|
||||
uv@1.43.0
|
||||
zlib@1.2.11
|
||||
ares@1.10.1-DEV
|
||||
icu@58.2
|
||||
modules@48
|
||||
napi@3
|
||||
openssl@1.0.2o
|
||||
brotli@1.0.9
|
||||
ares@1.18.1
|
||||
modules@93
|
||||
nghttp2@1.45.1
|
||||
napi@8
|
||||
llhttp@6.0.4
|
||||
openssl@1.1.1m+quic
|
||||
cldr@40.0
|
||||
icu@70.1
|
||||
tz@2021a3
|
||||
unicode@14.0
|
||||
ngtcp2@0.1.0-DEV
|
||||
nghttp3@0.1.0-DEV
|
||||
|
||||
> node benchmark/parse-top.js
|
||||
|
||||
cookie.parse - top sites
|
||||
|
||||
15 tests completed.
|
||||
|
||||
parse accounts.google.com x 2,421,245 ops/sec ±0.80% (188 runs sampled)
|
||||
parse apple.com x 2,684,710 ops/sec ±0.59% (189 runs sampled)
|
||||
parse cloudflare.com x 2,231,418 ops/sec ±0.76% (186 runs sampled)
|
||||
parse docs.google.com x 2,316,357 ops/sec ±1.28% (187 runs sampled)
|
||||
parse drive.google.com x 2,363,543 ops/sec ±0.49% (189 runs sampled)
|
||||
parse en.wikipedia.org x 839,414 ops/sec ±0.53% (189 runs sampled)
|
||||
parse linkedin.com x 553,797 ops/sec ±0.63% (190 runs sampled)
|
||||
parse maps.google.com x 1,314,779 ops/sec ±0.72% (189 runs sampled)
|
||||
parse microsoft.com x 153,783 ops/sec ±0.53% (190 runs sampled)
|
||||
parse play.google.com x 2,249,574 ops/sec ±0.59% (187 runs sampled)
|
||||
parse plus.google.com x 2,258,682 ops/sec ±0.60% (188 runs sampled)
|
||||
parse sites.google.com x 2,247,069 ops/sec ±0.68% (189 runs sampled)
|
||||
parse support.google.com x 1,456,840 ops/sec ±0.70% (187 runs sampled)
|
||||
parse www.google.com x 1,046,028 ops/sec ±0.58% (188 runs sampled)
|
||||
parse youtu.be x 937,428 ops/sec ±1.47% (190 runs sampled)
|
||||
parse youtube.com x 963,878 ops/sec ±0.59% (190 runs sampled)
|
||||
|
||||
> node benchmark/parse.js
|
||||
|
||||
cookie.parse
|
||||
cookie.parse - generic
|
||||
|
||||
6 tests completed.
|
||||
|
||||
simple x 1,200,691 ops/sec ±1.12% (189 runs sampled)
|
||||
decode x 1,012,994 ops/sec ±0.97% (186 runs sampled)
|
||||
unquote x 1,074,174 ops/sec ±2.43% (186 runs sampled)
|
||||
duplicates x 438,424 ops/sec ±2.17% (184 runs sampled)
|
||||
10 cookies x 147,154 ops/sec ±1.01% (186 runs sampled)
|
||||
100 cookies x 14,274 ops/sec ±1.07% (187 runs sampled)
|
||||
simple x 2,745,604 ops/sec ±0.77% (185 runs sampled)
|
||||
decode x 557,287 ops/sec ±0.60% (188 runs sampled)
|
||||
unquote x 2,498,475 ops/sec ±0.55% (189 runs sampled)
|
||||
duplicates x 868,591 ops/sec ±0.89% (187 runs sampled)
|
||||
10 cookies x 306,745 ops/sec ±0.49% (190 runs sampled)
|
||||
100 cookies x 22,414 ops/sec ±2.38% (182 runs sampled)
|
||||
```
|
||||
|
||||
## References
|
||||
|
||||
- [RFC 6265: HTTP State Management Mechanism][rfc-6265]
|
||||
- [Same-site Cookies][rfc-6265bis-03-4.1.2.7]
|
||||
- [Same-site Cookies][rfc-6265bis-09-5.4.7]
|
||||
|
||||
[rfc-6265bis-03-4.1.2.7]: https://tools.ietf.org/html/draft-ietf-httpbis-rfc6265bis-03#section-4.1.2.7
|
||||
[rfc-west-cookie-priority-00-4.1]: https://tools.ietf.org/html/draft-west-cookie-priority-00#section-4.1
|
||||
[rfc-6265bis-09-5.4.7]: https://tools.ietf.org/html/draft-ietf-httpbis-rfc6265bis-09#section-5.4.7
|
||||
[rfc-6265]: https://tools.ietf.org/html/rfc6265
|
||||
[rfc-6265-5.1.4]: https://tools.ietf.org/html/rfc6265#section-5.1.4
|
||||
[rfc-6265-5.2.1]: https://tools.ietf.org/html/rfc6265#section-5.2.1
|
||||
|
@ -248,10 +293,10 @@ $ npm run bench
|
|||
|
||||
[coveralls-image]: https://badgen.net/coveralls/c/github/jshttp/cookie/master
|
||||
[coveralls-url]: https://coveralls.io/r/jshttp/cookie?branch=master
|
||||
[github-actions-ci-image]: https://img.shields.io/github/workflow/status/jshttp/cookie/ci/master?label=ci
|
||||
[github-actions-ci-url]: https://github.com/jshttp/cookie/actions/workflows/ci.yml
|
||||
[node-version-image]: https://badgen.net/npm/node/cookie
|
||||
[node-version-url]: https://nodejs.org/en/download
|
||||
[npm-downloads-image]: https://badgen.net/npm/dm/cookie
|
||||
[npm-url]: https://npmjs.org/package/cookie
|
||||
[npm-version-image]: https://badgen.net/npm/v/cookie
|
||||
[travis-image]: https://badgen.net/travis/jshttp/cookie/master
|
||||
[travis-url]: https://travis-ci.org/jshttp/cookie
|
||||
|
|
|
@ -0,0 +1,25 @@
|
|||
# Security Policies and Procedures
|
||||
|
||||
## Reporting a Bug
|
||||
|
||||
The `cookie` team and community take all security bugs seriously. Thank
|
||||
you for improving the security of the project. We appreciate your efforts and
|
||||
responsible disclosure and will make every effort to acknowledge your
|
||||
contributions.
|
||||
|
||||
Report security bugs by emailing the current owner(s) of `cookie`. This
|
||||
information can be found in the npm registry using the command
|
||||
`npm owner ls cookie`.
|
||||
If unsure or unable to get the information from the above, open an issue
|
||||
in the [project issue tracker](https://github.com/jshttp/cookie/issues)
|
||||
asking for the current contact information.
|
||||
|
||||
To ensure the timely response to your report, please ensure that the entirety
|
||||
of the report is contained within the email body and not solely behind a web
|
||||
link or an attachment.
|
||||
|
||||
At least one owner will acknowledge your email within 48 hours, and will send a
|
||||
more detailed response within 48 hours indicating the next steps in handling
|
||||
your report. After the initial reply to your report, the owners will
|
||||
endeavor to keep you informed of the progress towards a fix and full
|
||||
announcement, and may ask for additional information or guidance.
|
|
@ -20,9 +20,7 @@ exports.serialize = serialize;
|
|||
* @private
|
||||
*/
|
||||
|
||||
var decode = decodeURIComponent;
|
||||
var encode = encodeURIComponent;
|
||||
var pairSplitRegExp = /; */;
|
||||
var __toString = Object.prototype.toString
|
||||
|
||||
/**
|
||||
* RegExp to match field-content in RFC 7230 sec 3.2
|
||||
|
@ -53,30 +51,42 @@ function parse(str, options) {
|
|||
|
||||
var obj = {}
|
||||
var opt = options || {};
|
||||
var pairs = str.split(pairSplitRegExp);
|
||||
var dec = opt.decode || decode;
|
||||
|
||||
for (var i = 0; i < pairs.length; i++) {
|
||||
var pair = pairs[i];
|
||||
var eq_idx = pair.indexOf('=');
|
||||
var index = 0
|
||||
while (index < str.length) {
|
||||
var eqIdx = str.indexOf('=', index)
|
||||
|
||||
// skip things that don't look like key=value
|
||||
if (eq_idx < 0) {
|
||||
continue;
|
||||
// no more cookie pairs
|
||||
if (eqIdx === -1) {
|
||||
break
|
||||
}
|
||||
|
||||
var key = pair.substr(0, eq_idx).trim()
|
||||
var val = pair.substr(++eq_idx, pair.length).trim();
|
||||
var endIdx = str.indexOf(';', index)
|
||||
|
||||
// quoted values
|
||||
if ('"' == val[0]) {
|
||||
val = val.slice(1, -1);
|
||||
if (endIdx === -1) {
|
||||
endIdx = str.length
|
||||
} else if (endIdx < eqIdx) {
|
||||
// backtrack on prior semicolon
|
||||
index = str.lastIndexOf(';', eqIdx - 1) + 1
|
||||
continue
|
||||
}
|
||||
|
||||
var key = str.slice(index, eqIdx).trim()
|
||||
|
||||
// only assign once
|
||||
if (undefined == obj[key]) {
|
||||
if (undefined === obj[key]) {
|
||||
var val = str.slice(eqIdx + 1, endIdx).trim()
|
||||
|
||||
// quoted values
|
||||
if (val.charCodeAt(0) === 0x22) {
|
||||
val = val.slice(1, -1)
|
||||
}
|
||||
|
||||
obj[key] = tryDecode(val, dec);
|
||||
}
|
||||
|
||||
index = endIdx + 1
|
||||
}
|
||||
|
||||
return obj;
|
||||
|
@ -145,11 +155,13 @@ function serialize(name, val, options) {
|
|||
}
|
||||
|
||||
if (opt.expires) {
|
||||
if (typeof opt.expires.toUTCString !== 'function') {
|
||||
var expires = opt.expires
|
||||
|
||||
if (!isDate(expires) || isNaN(expires.valueOf())) {
|
||||
throw new TypeError('option expires is invalid');
|
||||
}
|
||||
|
||||
str += '; Expires=' + opt.expires.toUTCString();
|
||||
str += '; Expires=' + expires.toUTCString()
|
||||
}
|
||||
|
||||
if (opt.httpOnly) {
|
||||
|
@ -160,6 +172,26 @@ function serialize(name, val, options) {
|
|||
str += '; Secure';
|
||||
}
|
||||
|
||||
if (opt.priority) {
|
||||
var priority = typeof opt.priority === 'string'
|
||||
? opt.priority.toLowerCase()
|
||||
: opt.priority
|
||||
|
||||
switch (priority) {
|
||||
case 'low':
|
||||
str += '; Priority=Low'
|
||||
break
|
||||
case 'medium':
|
||||
str += '; Priority=Medium'
|
||||
break
|
||||
case 'high':
|
||||
str += '; Priority=High'
|
||||
break
|
||||
default:
|
||||
throw new TypeError('option priority is invalid')
|
||||
}
|
||||
}
|
||||
|
||||
if (opt.sameSite) {
|
||||
var sameSite = typeof opt.sameSite === 'string'
|
||||
? opt.sameSite.toLowerCase() : opt.sameSite;
|
||||
|
@ -185,6 +217,42 @@ function serialize(name, val, options) {
|
|||
return str;
|
||||
}
|
||||
|
||||
/**
|
||||
* URL-decode string value. Optimized to skip native call when no %.
|
||||
*
|
||||
* @param {string} str
|
||||
* @returns {string}
|
||||
*/
|
||||
|
||||
function decode (str) {
|
||||
return str.indexOf('%') !== -1
|
||||
? decodeURIComponent(str)
|
||||
: str
|
||||
}
|
||||
|
||||
/**
|
||||
* URL-encode value.
|
||||
*
|
||||
* @param {string} str
|
||||
* @returns {string}
|
||||
*/
|
||||
|
||||
function encode (val) {
|
||||
return encodeURIComponent(val)
|
||||
}
|
||||
|
||||
/**
|
||||
* Determine if value is a Date.
|
||||
*
|
||||
* @param {*} val
|
||||
* @private
|
||||
*/
|
||||
|
||||
function isDate (val) {
|
||||
return __toString.call(val) === '[object Date]' ||
|
||||
val instanceof Date
|
||||
}
|
||||
|
||||
/**
|
||||
* Try decoding a string using a decoding function.
|
||||
*
|
||||
|
|
|
@ -1,7 +1,7 @@
|
|||
{
|
||||
"name": "cookie",
|
||||
"description": "HTTP server cookie parsing and serialization",
|
||||
"version": "0.4.1",
|
||||
"version": "0.5.0",
|
||||
"author": "Roman Shtylman <shtylman@gmail.com>",
|
||||
"contributors": [
|
||||
"Douglas Christopher Wilson <doug@somethingdoug.com>"
|
||||
|
@ -15,15 +15,18 @@
|
|||
"devDependencies": {
|
||||
"beautify-benchmark": "0.2.4",
|
||||
"benchmark": "2.1.4",
|
||||
"eslint": "6.8.0",
|
||||
"eslint-plugin-markdown": "1.0.2",
|
||||
"mocha": "7.1.1",
|
||||
"nyc": "15.0.1"
|
||||
"eslint": "7.32.0",
|
||||
"eslint-plugin-markdown": "2.2.1",
|
||||
"mocha": "9.2.2",
|
||||
"nyc": "15.1.0",
|
||||
"safe-buffer": "5.2.1",
|
||||
"top-sites": "1.1.97"
|
||||
},
|
||||
"files": [
|
||||
"HISTORY.md",
|
||||
"LICENSE",
|
||||
"README.md",
|
||||
"SECURITY.md",
|
||||
"index.js"
|
||||
],
|
||||
"engines": {
|
||||
|
@ -31,10 +34,11 @@
|
|||
},
|
||||
"scripts": {
|
||||
"bench": "node benchmark/index.js",
|
||||
"lint": "eslint --plugin markdown --ext js,md .",
|
||||
"test": "mocha --reporter spec --bail --check-leaks --ui qunit test/",
|
||||
"test-ci": "nyc --reporter=text npm test",
|
||||
"lint": "eslint .",
|
||||
"test": "mocha --reporter spec --bail --check-leaks test/",
|
||||
"test-ci": "nyc --reporter=lcov --reporter=text npm test",
|
||||
"test-cov": "nyc --reporter=html --reporter=text npm test",
|
||||
"update-bench": "node scripts/update-benchmark.js",
|
||||
"version": "node scripts/version-history.js && git add HISTORY.md"
|
||||
}
|
||||
}
|
||||
|
|
|
@ -1,3 +1,10 @@
|
|||
2.0.0 / 2018-10-26
|
||||
==================
|
||||
|
||||
* Drop support for Node.js 0.6
|
||||
* Replace internal `eval` usage with `Function` constructor
|
||||
* Use instance methods on `process` to check for listeners
|
||||
|
||||
1.1.2 / 2018-01-11
|
||||
==================
|
||||
|
||||
|
|
|
@ -1,6 +1,6 @@
|
|||
(The MIT License)
|
||||
|
||||
Copyright (c) 2014-2017 Douglas Christopher Wilson
|
||||
Copyright (c) 2014-2018 Douglas Christopher Wilson
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining
|
||||
a copy of this software and associated documentation files (the
|
||||
|
|
|
@ -267,14 +267,14 @@ deprecate.property(exports, 'oldprop', 'oldprop >= 0.10')
|
|||
|
||||
[MIT](LICENSE)
|
||||
|
||||
[npm-version-image]: https://img.shields.io/npm/v/depd.svg
|
||||
[npm-downloads-image]: https://img.shields.io/npm/dm/depd.svg
|
||||
[npm-url]: https://npmjs.org/package/depd
|
||||
[travis-image]: https://img.shields.io/travis/dougwilson/nodejs-depd/master.svg?label=linux
|
||||
[travis-url]: https://travis-ci.org/dougwilson/nodejs-depd
|
||||
[appveyor-image]: https://img.shields.io/appveyor/ci/dougwilson/nodejs-depd/master.svg?label=windows
|
||||
[appveyor-image]: https://badgen.net/appveyor/ci/dougwilson/nodejs-depd/master?label=windows
|
||||
[appveyor-url]: https://ci.appveyor.com/project/dougwilson/nodejs-depd
|
||||
[coveralls-image]: https://img.shields.io/coveralls/dougwilson/nodejs-depd/master.svg
|
||||
[coveralls-image]: https://badgen.net/coveralls/c/github/dougwilson/nodejs-depd/master
|
||||
[coveralls-url]: https://coveralls.io/r/dougwilson/nodejs-depd?branch=master
|
||||
[node-image]: https://img.shields.io/node/v/depd.svg
|
||||
[node-image]: https://badgen.net/npm/node/depd
|
||||
[node-url]: https://nodejs.org/en/download/
|
||||
[npm-downloads-image]: https://badgen.net/npm/dm/depd
|
||||
[npm-url]: https://npmjs.org/package/depd
|
||||
[npm-version-image]: https://badgen.net/npm/v/depd
|
||||
[travis-image]: https://badgen.net/travis/dougwilson/nodejs-depd/master?label=linux
|
||||
[travis-url]: https://travis-ci.org/dougwilson/nodejs-depd
|
||||
|
|
|
@ -1,6 +1,6 @@
|
|||
/*!
|
||||
* depd
|
||||
* Copyright(c) 2014-2017 Douglas Christopher Wilson
|
||||
* Copyright(c) 2014-2018 Douglas Christopher Wilson
|
||||
* MIT Licensed
|
||||
*/
|
||||
|
||||
|
@ -8,8 +8,6 @@
|
|||
* Module dependencies.
|
||||
*/
|
||||
|
||||
var callSiteToString = require('./lib/compat').callSiteToString
|
||||
var eventListenerCount = require('./lib/compat').eventListenerCount
|
||||
var relative = require('path').relative
|
||||
|
||||
/**
|
||||
|
@ -92,7 +90,7 @@ function createStackString (stack) {
|
|||
}
|
||||
|
||||
for (var i = 0; i < stack.length; i++) {
|
||||
str += '\n at ' + callSiteToString(stack[i])
|
||||
str += '\n at ' + stack[i].toString()
|
||||
}
|
||||
|
||||
return str
|
||||
|
@ -128,12 +126,31 @@ function depd (namespace) {
|
|||
return deprecate
|
||||
}
|
||||
|
||||
/**
|
||||
* Determine if event emitter has listeners of a given type.
|
||||
*
|
||||
* The way to do this check is done three different ways in Node.js >= 0.8
|
||||
* so this consolidates them into a minimal set using instance methods.
|
||||
*
|
||||
* @param {EventEmitter} emitter
|
||||
* @param {string} type
|
||||
* @returns {boolean}
|
||||
* @private
|
||||
*/
|
||||
|
||||
function eehaslisteners (emitter, type) {
|
||||
var count = typeof emitter.listenerCount !== 'function'
|
||||
? emitter.listeners(type).length
|
||||
: emitter.listenerCount(type)
|
||||
|
||||
return count > 0
|
||||
}
|
||||
|
||||
/**
|
||||
* Determine if namespace is ignored.
|
||||
*/
|
||||
|
||||
function isignored (namespace) {
|
||||
/* istanbul ignore next: tested in a child processs */
|
||||
if (process.noDeprecation) {
|
||||
// --no-deprecation support
|
||||
return true
|
||||
|
@ -150,7 +167,6 @@ function isignored (namespace) {
|
|||
*/
|
||||
|
||||
function istraced (namespace) {
|
||||
/* istanbul ignore next: tested in a child processs */
|
||||
if (process.traceDeprecation) {
|
||||
// --trace-deprecation support
|
||||
return true
|
||||
|
@ -167,7 +183,7 @@ function istraced (namespace) {
|
|||
*/
|
||||
|
||||
function log (message, site) {
|
||||
var haslisteners = eventListenerCount(process, 'deprecation') !== 0
|
||||
var haslisteners = eehaslisteners(process, 'deprecation')
|
||||
|
||||
// abort early if no destination
|
||||
if (!haslisteners && this._ignored) {
|
||||
|
@ -310,7 +326,7 @@ function formatPlain (msg, caller, stack) {
|
|||
// add stack trace
|
||||
if (this._traced) {
|
||||
for (var i = 0; i < stack.length; i++) {
|
||||
formatted += '\n at ' + callSiteToString(stack[i])
|
||||
formatted += '\n at ' + stack[i].toString()
|
||||
}
|
||||
|
||||
return formatted
|
||||
|
@ -335,7 +351,7 @@ function formatColor (msg, caller, stack) {
|
|||
// add stack trace
|
||||
if (this._traced) {
|
||||
for (var i = 0; i < stack.length; i++) {
|
||||
formatted += '\n \x1b[36mat ' + callSiteToString(stack[i]) + '\x1b[39m' // cyan
|
||||
formatted += '\n \x1b[36mat ' + stack[i].toString() + '\x1b[39m' // cyan
|
||||
}
|
||||
|
||||
return formatted
|
||||
|
@ -400,18 +416,18 @@ function wrapfunction (fn, message) {
|
|||
}
|
||||
|
||||
var args = createArgumentsString(fn.length)
|
||||
var deprecate = this // eslint-disable-line no-unused-vars
|
||||
var stack = getStack()
|
||||
var site = callSiteLocation(stack[1])
|
||||
|
||||
site.name = fn.name
|
||||
|
||||
// eslint-disable-next-line no-eval
|
||||
var deprecatedfn = eval('(function (' + args + ') {\n' +
|
||||
// eslint-disable-next-line no-new-func
|
||||
var deprecatedfn = new Function('fn', 'log', 'deprecate', 'message', 'site',
|
||||
'"use strict"\n' +
|
||||
'return function (' + args + ') {' +
|
||||
'log.call(deprecate, message, site)\n' +
|
||||
'return fn.apply(this, arguments)\n' +
|
||||
'})')
|
||||
'}')(fn, log, this, message, site)
|
||||
|
||||
return deprecatedfn
|
||||
}
|
||||
|
|
|
@ -1,103 +0,0 @@
|
|||
/*!
|
||||
* depd
|
||||
* Copyright(c) 2014 Douglas Christopher Wilson
|
||||
* MIT Licensed
|
||||
*/
|
||||
|
||||
'use strict'
|
||||
|
||||
/**
|
||||
* Module exports.
|
||||
*/
|
||||
|
||||
module.exports = callSiteToString
|
||||
|
||||
/**
|
||||
* Format a CallSite file location to a string.
|
||||
*/
|
||||
|
||||
function callSiteFileLocation (callSite) {
|
||||
var fileName
|
||||
var fileLocation = ''
|
||||
|
||||
if (callSite.isNative()) {
|
||||
fileLocation = 'native'
|
||||
} else if (callSite.isEval()) {
|
||||
fileName = callSite.getScriptNameOrSourceURL()
|
||||
if (!fileName) {
|
||||
fileLocation = callSite.getEvalOrigin()
|
||||
}
|
||||
} else {
|
||||
fileName = callSite.getFileName()
|
||||
}
|
||||
|
||||
if (fileName) {
|
||||
fileLocation += fileName
|
||||
|
||||
var lineNumber = callSite.getLineNumber()
|
||||
if (lineNumber != null) {
|
||||
fileLocation += ':' + lineNumber
|
||||
|
||||
var columnNumber = callSite.getColumnNumber()
|
||||
if (columnNumber) {
|
||||
fileLocation += ':' + columnNumber
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
return fileLocation || 'unknown source'
|
||||
}
|
||||
|
||||
/**
|
||||
* Format a CallSite to a string.
|
||||
*/
|
||||
|
||||
function callSiteToString (callSite) {
|
||||
var addSuffix = true
|
||||
var fileLocation = callSiteFileLocation(callSite)
|
||||
var functionName = callSite.getFunctionName()
|
||||
var isConstructor = callSite.isConstructor()
|
||||
var isMethodCall = !(callSite.isToplevel() || isConstructor)
|
||||
var line = ''
|
||||
|
||||
if (isMethodCall) {
|
||||
var methodName = callSite.getMethodName()
|
||||
var typeName = getConstructorName(callSite)
|
||||
|
||||
if (functionName) {
|
||||
if (typeName && functionName.indexOf(typeName) !== 0) {
|
||||
line += typeName + '.'
|
||||
}
|
||||
|
||||
line += functionName
|
||||
|
||||
if (methodName && functionName.lastIndexOf('.' + methodName) !== functionName.length - methodName.length - 1) {
|
||||
line += ' [as ' + methodName + ']'
|
||||
}
|
||||
} else {
|
||||
line += typeName + '.' + (methodName || '<anonymous>')
|
||||
}
|
||||
} else if (isConstructor) {
|
||||
line += 'new ' + (functionName || '<anonymous>')
|
||||
} else if (functionName) {
|
||||
line += functionName
|
||||
} else {
|
||||
addSuffix = false
|
||||
line += fileLocation
|
||||
}
|
||||
|
||||
if (addSuffix) {
|
||||
line += ' (' + fileLocation + ')'
|
||||
}
|
||||
|
||||
return line
|
||||
}
|
||||
|
||||
/**
|
||||
* Get constructor name of reviver.
|
||||
*/
|
||||
|
||||
function getConstructorName (obj) {
|
||||
var receiver = obj.receiver
|
||||
return (receiver.constructor && receiver.constructor.name) || null
|
||||
}
|
|
@ -1,22 +0,0 @@
|
|||
/*!
|
||||
* depd
|
||||
* Copyright(c) 2015 Douglas Christopher Wilson
|
||||
* MIT Licensed
|
||||
*/
|
||||
|
||||
'use strict'
|
||||
|
||||
/**
|
||||
* Module exports.
|
||||
* @public
|
||||
*/
|
||||
|
||||
module.exports = eventListenerCount
|
||||
|
||||
/**
|
||||
* Get the count of listeners on an event emitter of a specific type.
|
||||
*/
|
||||
|
||||
function eventListenerCount (emitter, type) {
|
||||
return emitter.listeners(type).length
|
||||
}
|
|
@ -1,79 +0,0 @@
|
|||
/*!
|
||||
* depd
|
||||
* Copyright(c) 2014-2015 Douglas Christopher Wilson
|
||||
* MIT Licensed
|
||||
*/
|
||||
|
||||
'use strict'
|
||||
|
||||
/**
|
||||
* Module dependencies.
|
||||
* @private
|
||||
*/
|
||||
|
||||
var EventEmitter = require('events').EventEmitter
|
||||
|
||||
/**
|
||||
* Module exports.
|
||||
* @public
|
||||
*/
|
||||
|
||||
lazyProperty(module.exports, 'callSiteToString', function callSiteToString () {
|
||||
var limit = Error.stackTraceLimit
|
||||
var obj = {}
|
||||
var prep = Error.prepareStackTrace
|
||||
|
||||
function prepareObjectStackTrace (obj, stack) {
|
||||
return stack
|
||||
}
|
||||
|
||||
Error.prepareStackTrace = prepareObjectStackTrace
|
||||
Error.stackTraceLimit = 2
|
||||
|
||||
// capture the stack
|
||||
Error.captureStackTrace(obj)
|
||||
|
||||
// slice the stack
|
||||
var stack = obj.stack.slice()
|
||||
|
||||
Error.prepareStackTrace = prep
|
||||
Error.stackTraceLimit = limit
|
||||
|
||||
return stack[0].toString ? toString : require('./callsite-tostring')
|
||||
})
|
||||
|
||||
lazyProperty(module.exports, 'eventListenerCount', function eventListenerCount () {
|
||||
return EventEmitter.listenerCount || require('./event-listener-count')
|
||||
})
|
||||
|
||||
/**
|
||||
* Define a lazy property.
|
||||
*/
|
||||
|
||||
function lazyProperty (obj, prop, getter) {
|
||||
function get () {
|
||||
var val = getter()
|
||||
|
||||
Object.defineProperty(obj, prop, {
|
||||
configurable: true,
|
||||
enumerable: true,
|
||||
value: val
|
||||
})
|
||||
|
||||
return val
|
||||
}
|
||||
|
||||
Object.defineProperty(obj, prop, {
|
||||
configurable: true,
|
||||
enumerable: true,
|
||||
get: get
|
||||
})
|
||||
}
|
||||
|
||||
/**
|
||||
* Call toString() on the obj
|
||||
*/
|
||||
|
||||
function toString (obj) {
|
||||
return obj.toString()
|
||||
}
|
|
@ -1,7 +1,7 @@
|
|||
{
|
||||
"name": "depd",
|
||||
"description": "Deprecate all the things",
|
||||
"version": "1.1.2",
|
||||
"version": "2.0.0",
|
||||
"author": "Douglas Christopher Wilson <doug@somethingdoug.com>",
|
||||
"license": "MIT",
|
||||
"keywords": [
|
||||
|
@ -13,13 +13,17 @@
|
|||
"devDependencies": {
|
||||
"benchmark": "2.1.4",
|
||||
"beautify-benchmark": "0.2.4",
|
||||
"eslint": "3.19.0",
|
||||
"eslint-config-standard": "7.1.0",
|
||||
"eslint": "5.7.0",
|
||||
"eslint-config-standard": "12.0.0",
|
||||
"eslint-plugin-import": "2.14.0",
|
||||
"eslint-plugin-markdown": "1.0.0-beta.7",
|
||||
"eslint-plugin-promise": "3.6.0",
|
||||
"eslint-plugin-standard": "3.0.1",
|
||||
"eslint-plugin-node": "7.0.1",
|
||||
"eslint-plugin-promise": "4.0.1",
|
||||
"eslint-plugin-standard": "4.0.0",
|
||||
"istanbul": "0.4.5",
|
||||
"mocha": "~1.21.5"
|
||||
"mocha": "5.2.0",
|
||||
"safe-buffer": "5.1.2",
|
||||
"uid-safe": "2.1.5"
|
||||
},
|
||||
"files": [
|
||||
"lib/",
|
||||
|
@ -29,13 +33,13 @@
|
|||
"Readme.md"
|
||||
],
|
||||
"engines": {
|
||||
"node": ">= 0.6"
|
||||
"node": ">= 0.8"
|
||||
},
|
||||
"scripts": {
|
||||
"bench": "node benchmark/index.js",
|
||||
"lint": "eslint --plugin markdown --ext js,md .",
|
||||
"test": "mocha --reporter spec --bail test/",
|
||||
"test-ci": "istanbul cover node_modules/mocha/bin/_mocha --report lcovonly -- --reporter spec --no-exit test/",
|
||||
"test-cov": "istanbul cover node_modules/mocha/bin/_mocha -- --reporter dot test/"
|
||||
"test-ci": "istanbul cover --print=none node_modules/mocha/bin/_mocha -- --reporter spec test/ && istanbul report lcovonly text-summary",
|
||||
"test-cov": "istanbul cover --print=none node_modules/mocha/bin/_mocha -- --reporter dot test/ && istanbul report lcov text-summary"
|
||||
}
|
||||
}
|
||||
|
|
|
@ -2,6 +2,7 @@
|
|||
The MIT License (MIT)
|
||||
|
||||
Copyright (c) 2014 Jonathan Ong me@jongleberry.com
|
||||
Copyright (c) 2015-2022 Douglas Christopher Wilson doug@somethingdoug.com
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
of this software and associated documentation files (the "Software"), to deal
|
||||
|
|
|
@ -1,11 +1,10 @@
|
|||
# Destroy
|
||||
# destroy
|
||||
|
||||
[![NPM version][npm-image]][npm-url]
|
||||
[![Build status][travis-image]][travis-url]
|
||||
[![Build Status][github-actions-ci-image]][github-actions-ci-url]
|
||||
[![Test coverage][coveralls-image]][coveralls-url]
|
||||
[![License][license-image]][license-url]
|
||||
[![Downloads][downloads-image]][downloads-url]
|
||||
[![Gittip][gittip-image]][gittip-url]
|
||||
|
||||
Destroy a stream.
|
||||
|
||||
|
@ -18,17 +17,23 @@ and Node.js bugs.
|
|||
var destroy = require('destroy')
|
||||
```
|
||||
|
||||
### destroy(stream)
|
||||
### destroy(stream [, suppress])
|
||||
|
||||
Destroy the given stream. In most cases, this is identical to a simple
|
||||
`stream.destroy()` call. The rules are as follows for a given stream:
|
||||
Destroy the given stream, and optionally suppress any future `error` events.
|
||||
|
||||
In most cases, this is identical to a simple `stream.destroy()` call. The rules
|
||||
are as follows for a given stream:
|
||||
|
||||
1. If the `stream` is an instance of `ReadStream`, then call `stream.destroy()`
|
||||
and add a listener to the `open` event to call `stream.close()` if it is
|
||||
fired. This is for a Node.js bug that will leak a file descriptor if
|
||||
`.destroy()` is called before `open`.
|
||||
2. If the `stream` is not an instance of `Stream`, then nothing happens.
|
||||
3. If the `stream` has a `.destroy()` method, then call it.
|
||||
2. If the `stream` is an instance of a zlib stream, then call `stream.destroy()`
|
||||
and close the underlying zlib handle if open, otherwise call `stream.close()`.
|
||||
This is for consistency across Node.js versions and a Node.js bug that will
|
||||
leak a native zlib handle.
|
||||
3. If the `stream` is not an instance of `Stream`, then nothing happens.
|
||||
4. If the `stream` has a `.destroy()` method, then call it.
|
||||
|
||||
The function returns the `stream` passed in as the argument.
|
||||
|
||||
|
@ -48,13 +53,11 @@ destroy(stream)
|
|||
[npm-url]: https://npmjs.org/package/destroy
|
||||
[github-tag]: http://img.shields.io/github/tag/stream-utils/destroy.svg?style=flat-square
|
||||
[github-url]: https://github.com/stream-utils/destroy/tags
|
||||
[travis-image]: https://img.shields.io/travis/stream-utils/destroy.svg?style=flat-square
|
||||
[travis-url]: https://travis-ci.org/stream-utils/destroy
|
||||
[coveralls-image]: https://img.shields.io/coveralls/stream-utils/destroy.svg?style=flat-square
|
||||
[coveralls-url]: https://coveralls.io/r/stream-utils/destroy?branch=master
|
||||
[license-image]: http://img.shields.io/npm/l/destroy.svg?style=flat-square
|
||||
[license-url]: LICENSE.md
|
||||
[downloads-image]: http://img.shields.io/npm/dm/destroy.svg?style=flat-square
|
||||
[downloads-url]: https://npmjs.org/package/destroy
|
||||
[gittip-image]: https://img.shields.io/gittip/jonathanong.svg?style=flat-square
|
||||
[gittip-url]: https://www.gittip.com/jonathanong/
|
||||
[github-actions-ci-image]: https://img.shields.io/github/workflow/status/stream-utils/destroy/ci/master?label=ci&style=flat-square
|
||||
[github-actions-ci-url]: https://github.com/stream-utils/destroy/actions/workflows/ci.yml
|
||||
|
|
|
@ -1,6 +1,7 @@
|
|||
/*!
|
||||
* destroy
|
||||
* Copyright(c) 2014 Jonathan Ong
|
||||
* Copyright(c) 2015-2022 Douglas Christopher Wilson
|
||||
* MIT Licensed
|
||||
*/
|
||||
|
||||
|
@ -11,8 +12,10 @@
|
|||
* @private
|
||||
*/
|
||||
|
||||
var EventEmitter = require('events').EventEmitter
|
||||
var ReadStream = require('fs').ReadStream
|
||||
var Stream = require('stream')
|
||||
var Zlib = require('zlib')
|
||||
|
||||
/**
|
||||
* Module exports.
|
||||
|
@ -22,25 +25,27 @@ var Stream = require('stream')
|
|||
module.exports = destroy
|
||||
|
||||
/**
|
||||
* Destroy a stream.
|
||||
* Destroy the given stream, and optionally suppress any future `error` events.
|
||||
*
|
||||
* @param {object} stream
|
||||
* @param {boolean} suppress
|
||||
* @public
|
||||
*/
|
||||
|
||||
function destroy(stream) {
|
||||
if (stream instanceof ReadStream) {
|
||||
return destroyReadStream(stream)
|
||||
}
|
||||
|
||||
if (!(stream instanceof Stream)) {
|
||||
return stream
|
||||
}
|
||||
|
||||
if (typeof stream.destroy === 'function') {
|
||||
function destroy (stream, suppress) {
|
||||
if (isFsReadStream(stream)) {
|
||||
destroyReadStream(stream)
|
||||
} else if (isZlibStream(stream)) {
|
||||
destroyZlibStream(stream)
|
||||
} else if (hasDestroy(stream)) {
|
||||
stream.destroy()
|
||||
}
|
||||
|
||||
if (isEventEmitter(stream) && suppress) {
|
||||
stream.removeAllListeners('error')
|
||||
stream.addListener('error', noop)
|
||||
}
|
||||
|
||||
return stream
|
||||
}
|
||||
|
||||
|
@ -51,15 +56,144 @@ function destroy(stream) {
|
|||
* @private
|
||||
*/
|
||||
|
||||
function destroyReadStream(stream) {
|
||||
function destroyReadStream (stream) {
|
||||
stream.destroy()
|
||||
|
||||
if (typeof stream.close === 'function') {
|
||||
// node.js core bug work-around
|
||||
stream.on('open', onOpenClose)
|
||||
}
|
||||
}
|
||||
|
||||
return stream
|
||||
/**
|
||||
* Close a Zlib stream.
|
||||
*
|
||||
* Zlib streams below Node.js 4.5.5 have a buggy implementation
|
||||
* of .close() when zlib encountered an error.
|
||||
*
|
||||
* @param {object} stream
|
||||
* @private
|
||||
*/
|
||||
|
||||
function closeZlibStream (stream) {
|
||||
if (stream._hadError === true) {
|
||||
var prop = stream._binding === null
|
||||
? '_binding'
|
||||
: '_handle'
|
||||
|
||||
stream[prop] = {
|
||||
close: function () { this[prop] = null }
|
||||
}
|
||||
}
|
||||
|
||||
stream.close()
|
||||
}
|
||||
|
||||
/**
|
||||
* Destroy a Zlib stream.
|
||||
*
|
||||
* Zlib streams don't have a destroy function in Node.js 6. On top of that
|
||||
* simply calling destroy on a zlib stream in Node.js 8+ will result in a
|
||||
* memory leak. So until that is fixed, we need to call both close AND destroy.
|
||||
*
|
||||
* PR to fix memory leak: https://github.com/nodejs/node/pull/23734
|
||||
*
|
||||
* In Node.js 6+8, it's important that destroy is called before close as the
|
||||
* stream would otherwise emit the error 'zlib binding closed'.
|
||||
*
|
||||
* @param {object} stream
|
||||
* @private
|
||||
*/
|
||||
|
||||
function destroyZlibStream (stream) {
|
||||
if (typeof stream.destroy === 'function') {
|
||||
// node.js core bug work-around
|
||||
// istanbul ignore if: node.js 0.8
|
||||
if (stream._binding) {
|
||||
// node.js < 0.10.0
|
||||
stream.destroy()
|
||||
if (stream._processing) {
|
||||
stream._needDrain = true
|
||||
stream.once('drain', onDrainClearBinding)
|
||||
} else {
|
||||
stream._binding.clear()
|
||||
}
|
||||
} else if (stream._destroy && stream._destroy !== Stream.Transform.prototype._destroy) {
|
||||
// node.js >= 12, ^11.1.0, ^10.15.1
|
||||
stream.destroy()
|
||||
} else if (stream._destroy && typeof stream.close === 'function') {
|
||||
// node.js 7, 8
|
||||
stream.destroyed = true
|
||||
stream.close()
|
||||
} else {
|
||||
// fallback
|
||||
// istanbul ignore next
|
||||
stream.destroy()
|
||||
}
|
||||
} else if (typeof stream.close === 'function') {
|
||||
// node.js < 8 fallback
|
||||
closeZlibStream(stream)
|
||||
}
|
||||
}
|
||||
|
||||
/**
|
||||
* Determine if stream has destroy.
|
||||
* @private
|
||||
*/
|
||||
|
||||
function hasDestroy (stream) {
|
||||
return stream instanceof Stream &&
|
||||
typeof stream.destroy === 'function'
|
||||
}
|
||||
|
||||
/**
|
||||
* Determine if val is EventEmitter.
|
||||
* @private
|
||||
*/
|
||||
|
||||
function isEventEmitter (val) {
|
||||
return val instanceof EventEmitter
|
||||
}
|
||||
|
||||
/**
|
||||
* Determine if stream is fs.ReadStream stream.
|
||||
* @private
|
||||
*/
|
||||
|
||||
function isFsReadStream (stream) {
|
||||
return stream instanceof ReadStream
|
||||
}
|
||||
|
||||
/**
|
||||
* Determine if stream is Zlib stream.
|
||||
* @private
|
||||
*/
|
||||
|
||||
function isZlibStream (stream) {
|
||||
return stream instanceof Zlib.Gzip ||
|
||||
stream instanceof Zlib.Gunzip ||
|
||||
stream instanceof Zlib.Deflate ||
|
||||
stream instanceof Zlib.DeflateRaw ||
|
||||
stream instanceof Zlib.Inflate ||
|
||||
stream instanceof Zlib.InflateRaw ||
|
||||
stream instanceof Zlib.Unzip
|
||||
}
|
||||
|
||||
/**
|
||||
* No-op function.
|
||||
* @private
|
||||
*/
|
||||
|
||||
function noop () {}
|
||||
|
||||
/**
|
||||
* On drain handler to clear binding.
|
||||
* @private
|
||||
*/
|
||||
|
||||
// istanbul ignore next: node.js 0.8
|
||||
function onDrainClearBinding () {
|
||||
this._binding.clear()
|
||||
}
|
||||
|
||||
/**
|
||||
|
@ -67,7 +201,7 @@ function destroyReadStream(stream) {
|
|||
* @private
|
||||
*/
|
||||
|
||||
function onOpenClose() {
|
||||
function onOpenClose () {
|
||||
if (typeof this.fd === 'number') {
|
||||
// actually close down the fd
|
||||
this.close()
|
||||
|
|
|
@ -1,7 +1,7 @@
|
|||
{
|
||||
"name": "destroy",
|
||||
"description": "destroy a stream if possible",
|
||||
"version": "1.0.4",
|
||||
"version": "1.2.0",
|
||||
"author": {
|
||||
"name": "Jonathan Ong",
|
||||
"email": "me@jongleberry.com",
|
||||
|
@ -14,13 +14,24 @@
|
|||
"license": "MIT",
|
||||
"repository": "stream-utils/destroy",
|
||||
"devDependencies": {
|
||||
"istanbul": "0.4.2",
|
||||
"mocha": "2.3.4"
|
||||
"eslint": "7.32.0",
|
||||
"eslint-config-standard": "14.1.1",
|
||||
"eslint-plugin-import": "2.25.4",
|
||||
"eslint-plugin-node": "11.1.0",
|
||||
"eslint-plugin-promise": "5.2.0",
|
||||
"eslint-plugin-standard": "4.1.0",
|
||||
"mocha": "9.2.2",
|
||||
"nyc": "15.1.0"
|
||||
},
|
||||
"engines": {
|
||||
"node": ">= 0.8",
|
||||
"npm": "1.2.8000 || >= 1.4.16"
|
||||
},
|
||||
"scripts": {
|
||||
"lint": "eslint .",
|
||||
"test": "mocha --reporter spec",
|
||||
"test-cov": "istanbul cover node_modules/mocha/bin/_mocha -- --reporter dot",
|
||||
"test-travis": "istanbul cover node_modules/mocha/bin/_mocha --report lcovonly -- --reporter dot"
|
||||
"test-ci": "nyc --reporter=lcovonly --reporter=text npm test",
|
||||
"test-cov": "nyc --reporter=html --reporter=text npm test"
|
||||
},
|
||||
"files": [
|
||||
"index.js",
|
||||
|
|
|
@ -1,3 +1,81 @@
|
|||
4.18.2 / 2022-10-08
|
||||
===================
|
||||
|
||||
* Fix regression routing a large stack in a single route
|
||||
* deps: body-parser@1.20.1
|
||||
- deps: qs@6.11.0
|
||||
- perf: remove unnecessary object clone
|
||||
* deps: qs@6.11.0
|
||||
|
||||
4.18.1 / 2022-04-29
|
||||
===================
|
||||
|
||||
* Fix hanging on large stack of sync routes
|
||||
|
||||
4.18.0 / 2022-04-25
|
||||
===================
|
||||
|
||||
* Add "root" option to `res.download`
|
||||
* Allow `options` without `filename` in `res.download`
|
||||
* Deprecate string and non-integer arguments to `res.status`
|
||||
* Fix behavior of `null`/`undefined` as `maxAge` in `res.cookie`
|
||||
* Fix handling very large stacks of sync middleware
|
||||
* Ignore `Object.prototype` values in settings through `app.set`/`app.get`
|
||||
* Invoke `default` with same arguments as types in `res.format`
|
||||
* Support proper 205 responses using `res.send`
|
||||
* Use `http-errors` for `res.format` error
|
||||
* deps: body-parser@1.20.0
|
||||
- Fix error message for json parse whitespace in `strict`
|
||||
- Fix internal error when inflated body exceeds limit
|
||||
- Prevent loss of async hooks context
|
||||
- Prevent hanging when request already read
|
||||
- deps: depd@2.0.0
|
||||
- deps: http-errors@2.0.0
|
||||
- deps: on-finished@2.4.1
|
||||
- deps: qs@6.10.3
|
||||
- deps: raw-body@2.5.1
|
||||
* deps: cookie@0.5.0
|
||||
- Add `priority` option
|
||||
- Fix `expires` option to reject invalid dates
|
||||
* deps: depd@2.0.0
|
||||
- Replace internal `eval` usage with `Function` constructor
|
||||
- Use instance methods on `process` to check for listeners
|
||||
* deps: finalhandler@1.2.0
|
||||
- Remove set content headers that break response
|
||||
- deps: on-finished@2.4.1
|
||||
- deps: statuses@2.0.1
|
||||
* deps: on-finished@2.4.1
|
||||
- Prevent loss of async hooks context
|
||||
* deps: qs@6.10.3
|
||||
* deps: send@0.18.0
|
||||
- Fix emitted 416 error missing headers property
|
||||
- Limit the headers removed for 304 response
|
||||
- deps: depd@2.0.0
|
||||
- deps: destroy@1.2.0
|
||||
- deps: http-errors@2.0.0
|
||||
- deps: on-finished@2.4.1
|
||||
- deps: statuses@2.0.1
|
||||
* deps: serve-static@1.15.0
|
||||
- deps: send@0.18.0
|
||||
* deps: statuses@2.0.1
|
||||
- Remove code 306
|
||||
- Rename `425 Unordered Collection` to standard `425 Too Early`
|
||||
|
||||
4.17.3 / 2022-02-16
|
||||
===================
|
||||
|
||||
* deps: accepts@~1.3.8
|
||||
- deps: mime-types@~2.1.34
|
||||
- deps: negotiator@0.6.3
|
||||
* deps: body-parser@1.19.2
|
||||
- deps: bytes@3.1.2
|
||||
- deps: qs@6.9.7
|
||||
- deps: raw-body@2.4.3
|
||||
* deps: cookie@0.4.2
|
||||
* deps: qs@6.9.7
|
||||
* Fix handling of `__proto__` keys
|
||||
* pref: remove unnecessary regexp for trust proxy
|
||||
|
||||
4.17.2 / 2021-12-16
|
||||
===================
|
||||
|
||||
|
|
|
@ -1,12 +1,10 @@
|
|||
[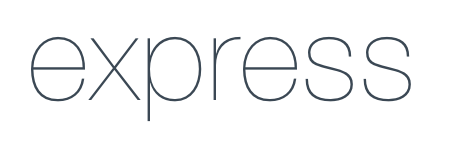](http://expressjs.com/)
|
||||
|
||||
Fast, unopinionated, minimalist web framework for [node](http://nodejs.org).
|
||||
Fast, unopinionated, minimalist web framework for [Node.js](http://nodejs.org).
|
||||
|
||||
[![NPM Version][npm-image]][npm-url]
|
||||
[![NPM Downloads][downloads-image]][downloads-url]
|
||||
[![Linux Build][ci-image]][ci-url]
|
||||
[![Windows Build][appveyor-image]][appveyor-url]
|
||||
[![Test Coverage][coveralls-image]][coveralls-url]
|
||||
[![NPM Version][npm-version-image]][npm-url]
|
||||
[![NPM Install Size][npm-install-size-image]][npm-install-size-url]
|
||||
[![NPM Downloads][npm-downloads-image]][npm-downloads-url]
|
||||
|
||||
```js
|
||||
const express = require('express')
|
||||
|
@ -33,7 +31,7 @@ the [`npm init` command](https://docs.npmjs.com/creating-a-package-json-file).
|
|||
Installation is done using the
|
||||
[`npm install` command](https://docs.npmjs.com/getting-started/installing-npm-packages-locally):
|
||||
|
||||
```bash
|
||||
```console
|
||||
$ npm install express
|
||||
```
|
||||
|
||||
|
@ -53,7 +51,7 @@ for more information.
|
|||
## Docs & Community
|
||||
|
||||
* [Website and Documentation](http://expressjs.com/) - [[website repo](https://github.com/expressjs/expressjs.com)]
|
||||
* [#express](https://webchat.freenode.net/?channels=express) on freenode IRC
|
||||
* [#express](https://web.libera.chat/#express) on [Libera Chat](https://libera.chat) IRC
|
||||
* [GitHub Organization](https://github.com/expressjs) for Official Middleware & Modules
|
||||
* Visit the [Wiki](https://github.com/expressjs/express/wiki)
|
||||
* [Google Group](https://groups.google.com/group/express-js) for discussion
|
||||
|
@ -61,35 +59,31 @@ for more information.
|
|||
|
||||
**PROTIP** Be sure to read [Migrating from 3.x to 4.x](https://github.com/expressjs/express/wiki/Migrating-from-3.x-to-4.x) as well as [New features in 4.x](https://github.com/expressjs/express/wiki/New-features-in-4.x).
|
||||
|
||||
### Security Issues
|
||||
|
||||
If you discover a security vulnerability in Express, please see [Security Policies and Procedures](Security.md).
|
||||
|
||||
## Quick Start
|
||||
|
||||
The quickest way to get started with express is to utilize the executable [`express(1)`](https://github.com/expressjs/generator) to generate an application as shown below:
|
||||
|
||||
Install the executable. The executable's major version will match Express's:
|
||||
|
||||
```bash
|
||||
```console
|
||||
$ npm install -g express-generator@4
|
||||
```
|
||||
|
||||
Create the app:
|
||||
|
||||
```bash
|
||||
```console
|
||||
$ express /tmp/foo && cd /tmp/foo
|
||||
```
|
||||
|
||||
Install dependencies:
|
||||
|
||||
```bash
|
||||
```console
|
||||
$ npm install
|
||||
```
|
||||
|
||||
Start the server:
|
||||
|
||||
```bash
|
||||
```console
|
||||
$ npm start
|
||||
```
|
||||
|
||||
|
@ -109,7 +103,7 @@ $ npm start
|
|||
|
||||
To view the examples, clone the Express repo and install the dependencies:
|
||||
|
||||
```bash
|
||||
```console
|
||||
$ git clone git://github.com/expressjs/express.git --depth 1
|
||||
$ cd express
|
||||
$ npm install
|
||||
|
@ -117,22 +111,34 @@ $ npm install
|
|||
|
||||
Then run whichever example you want:
|
||||
|
||||
```bash
|
||||
```console
|
||||
$ node examples/content-negotiation
|
||||
```
|
||||
|
||||
## Tests
|
||||
|
||||
To run the test suite, first install the dependencies, then run `npm test`:
|
||||
|
||||
```bash
|
||||
$ npm install
|
||||
$ npm test
|
||||
```
|
||||
|
||||
## Contributing
|
||||
|
||||
[Contributing Guide](Contributing.md)
|
||||
[![Linux Build][github-actions-ci-image]][github-actions-ci-url]
|
||||
[![Windows Build][appveyor-image]][appveyor-url]
|
||||
[![Test Coverage][coveralls-image]][coveralls-url]
|
||||
|
||||
The Express.js project welcomes all constructive contributions. Contributions take many forms,
|
||||
from code for bug fixes and enhancements, to additions and fixes to documentation, additional
|
||||
tests, triaging incoming pull requests and issues, and more!
|
||||
|
||||
See the [Contributing Guide](Contributing.md) for more technical details on contributing.
|
||||
|
||||
### Security Issues
|
||||
|
||||
If you discover a security vulnerability in Express, please see [Security Policies and Procedures](Security.md).
|
||||
|
||||
### Running Tests
|
||||
|
||||
To run the test suite, first install the dependencies, then run `npm test`:
|
||||
|
||||
```console
|
||||
$ npm install
|
||||
$ npm test
|
||||
```
|
||||
|
||||
## People
|
||||
|
||||
|
@ -146,13 +152,15 @@ The current lead maintainer is [Douglas Christopher Wilson](https://github.com/d
|
|||
|
||||
[MIT](LICENSE)
|
||||
|
||||
[ci-image]: https://img.shields.io/github/workflow/status/expressjs/express/ci/master.svg?label=linux
|
||||
[ci-url]: https://github.com/expressjs/express/actions?query=workflow%3Aci
|
||||
[npm-image]: https://img.shields.io/npm/v/express.svg
|
||||
[npm-url]: https://npmjs.org/package/express
|
||||
[downloads-image]: https://img.shields.io/npm/dm/express.svg
|
||||
[downloads-url]: https://npmcharts.com/compare/express?minimal=true
|
||||
[appveyor-image]: https://img.shields.io/appveyor/ci/dougwilson/express/master.svg?label=windows
|
||||
[appveyor-image]: https://badgen.net/appveyor/ci/dougwilson/express/master?label=windows
|
||||
[appveyor-url]: https://ci.appveyor.com/project/dougwilson/express
|
||||
[coveralls-image]: https://img.shields.io/coveralls/expressjs/express/master.svg
|
||||
[coveralls-image]: https://badgen.net/coveralls/c/github/expressjs/express/master
|
||||
[coveralls-url]: https://coveralls.io/r/expressjs/express?branch=master
|
||||
[github-actions-ci-image]: https://badgen.net/github/checks/expressjs/express/master?label=linux
|
||||
[github-actions-ci-url]: https://github.com/expressjs/express/actions/workflows/ci.yml
|
||||
[npm-downloads-image]: https://badgen.net/npm/dm/express
|
||||
[npm-downloads-url]: https://npmcharts.com/compare/express?minimal=true
|
||||
[npm-install-size-image]: https://badgen.net/packagephobia/install/express
|
||||
[npm-install-size-url]: https://packagephobia.com/result?p=express
|
||||
[npm-url]: https://npmjs.org/package/express
|
||||
[npm-version-image]: https://badgen.net/npm/v/express
|
||||
|
|
|
@ -29,6 +29,13 @@ var flatten = require('array-flatten');
|
|||
var merge = require('utils-merge');
|
||||
var resolve = require('path').resolve;
|
||||
var setPrototypeOf = require('setprototypeof')
|
||||
|
||||
/**
|
||||
* Module variables.
|
||||
* @private
|
||||
*/
|
||||
|
||||
var hasOwnProperty = Object.prototype.hasOwnProperty
|
||||
var slice = Array.prototype.slice;
|
||||
|
||||
/**
|
||||
|
@ -352,7 +359,17 @@ app.param = function param(name, fn) {
|
|||
app.set = function set(setting, val) {
|
||||
if (arguments.length === 1) {
|
||||
// app.get(setting)
|
||||
return this.settings[setting];
|
||||
var settings = this.settings
|
||||
|
||||
while (settings && settings !== Object.prototype) {
|
||||
if (hasOwnProperty.call(settings, setting)) {
|
||||
return settings[setting]
|
||||
}
|
||||
|
||||
settings = Object.getPrototypeOf(settings)
|
||||
}
|
||||
|
||||
return undefined
|
||||
}
|
||||
|
||||
debug('set "%s" to %o', setting, val);
|
||||
|
|
|
@ -14,6 +14,7 @@
|
|||
|
||||
var Buffer = require('safe-buffer').Buffer
|
||||
var contentDisposition = require('content-disposition');
|
||||
var createError = require('http-errors')
|
||||
var deprecate = require('depd')('express');
|
||||
var encodeUrl = require('encodeurl');
|
||||
var escapeHtml = require('escape-html');
|
||||
|
@ -64,6 +65,9 @@ var charsetRegExp = /;\s*charset\s*=/;
|
|||
*/
|
||||
|
||||
res.status = function status(code) {
|
||||
if ((typeof code === 'string' || Math.floor(code) !== code) && code > 99 && code < 1000) {
|
||||
deprecate('res.status(' + JSON.stringify(code) + '): use res.status(' + Math.floor(code) + ') instead')
|
||||
}
|
||||
this.statusCode = code;
|
||||
return this;
|
||||
};
|
||||
|
@ -135,7 +139,7 @@ res.send = function send(body) {
|
|||
|
||||
deprecate('res.send(status): Use res.sendStatus(status) instead');
|
||||
this.statusCode = chunk;
|
||||
chunk = statuses[chunk]
|
||||
chunk = statuses.message[chunk]
|
||||
}
|
||||
|
||||
switch (typeof chunk) {
|
||||
|
@ -213,6 +217,13 @@ res.send = function send(body) {
|
|||
chunk = '';
|
||||
}
|
||||
|
||||
// alter headers for 205
|
||||
if (this.statusCode === 205) {
|
||||
this.set('Content-Length', '0')
|
||||
this.removeHeader('Transfer-Encoding')
|
||||
chunk = ''
|
||||
}
|
||||
|
||||
if (req.method === 'HEAD') {
|
||||
// skip body for HEAD
|
||||
this.end();
|
||||
|
@ -356,7 +367,7 @@ res.jsonp = function jsonp(obj) {
|
|||
*/
|
||||
|
||||
res.sendStatus = function sendStatus(statusCode) {
|
||||
var body = statuses[statusCode] || String(statusCode)
|
||||
var body = statuses.message[statusCode] || String(statusCode)
|
||||
|
||||
this.statusCode = statusCode;
|
||||
this.type('txt');
|
||||
|
@ -524,7 +535,7 @@ res.sendfile = deprecate.function(res.sendfile,
|
|||
* Optionally providing an alternate attachment `filename`,
|
||||
* and optional callback `callback(err)`. The callback is invoked
|
||||
* when the data transfer is complete, or when an error has
|
||||
* ocurred. Be sure to check `res.headersSent` if you plan to respond.
|
||||
* occurred. Be sure to check `res.headersSent` if you plan to respond.
|
||||
*
|
||||
* Optionally providing an `options` object to use with `res.sendFile()`.
|
||||
* This function will set the `Content-Disposition` header, overriding
|
||||
|
@ -551,6 +562,13 @@ res.download = function download (path, filename, options, callback) {
|
|||
opts = null
|
||||
}
|
||||
|
||||
// support optional filename, where options may be in it's place
|
||||
if (typeof filename === 'object' &&
|
||||
(typeof options === 'function' || options === undefined)) {
|
||||
name = null
|
||||
opts = filename
|
||||
}
|
||||
|
||||
// set Content-Disposition when file is sent
|
||||
var headers = {
|
||||
'Content-Disposition': contentDisposition(name || path)
|
||||
|
@ -572,7 +590,9 @@ res.download = function download (path, filename, options, callback) {
|
|||
opts.headers = headers
|
||||
|
||||
// Resolve the full path for sendFile
|
||||
var fullPath = resolve(path);
|
||||
var fullPath = !opts.root
|
||||
? resolve(path)
|
||||
: path
|
||||
|
||||
// send file
|
||||
return this.sendFile(fullPath, opts, done)
|
||||
|
@ -665,9 +685,8 @@ res.format = function(obj){
|
|||
var req = this.req;
|
||||
var next = req.next;
|
||||
|
||||
var fn = obj.default;
|
||||
if (fn) delete obj.default;
|
||||
var keys = Object.keys(obj);
|
||||
var keys = Object.keys(obj)
|
||||
.filter(function (v) { return v !== 'default' })
|
||||
|
||||
var key = keys.length > 0
|
||||
? req.accepts(keys)
|
||||
|
@ -678,13 +697,12 @@ res.format = function(obj){
|
|||
if (key) {
|
||||
this.set('Content-Type', normalizeType(key).value);
|
||||
obj[key](req, this, next);
|
||||
} else if (fn) {
|
||||
fn();
|
||||
} else if (obj.default) {
|
||||
obj.default(req, this, next)
|
||||
} else {
|
||||
var err = new Error('Not Acceptable');
|
||||
err.status = err.statusCode = 406;
|
||||
err.types = normalizeTypes(keys).map(function(o){ return o.value });
|
||||
next(err);
|
||||
next(createError(406, {
|
||||
types: normalizeTypes(keys).map(function (o) { return o.value })
|
||||
}))
|
||||
}
|
||||
|
||||
return this;
|
||||
|
@ -850,9 +868,13 @@ res.cookie = function (name, value, options) {
|
|||
val = 's:' + sign(val, secret);
|
||||
}
|
||||
|
||||
if ('maxAge' in opts) {
|
||||
opts.expires = new Date(Date.now() + opts.maxAge);
|
||||
opts.maxAge /= 1000;
|
||||
if (opts.maxAge != null) {
|
||||
var maxAge = opts.maxAge - 0
|
||||
|
||||
if (!isNaN(maxAge)) {
|
||||
opts.expires = new Date(Date.now() + maxAge)
|
||||
opts.maxAge = Math.floor(maxAge / 1000)
|
||||
}
|
||||
}
|
||||
|
||||
if (opts.path == null) {
|
||||
|
@ -933,12 +955,12 @@ res.redirect = function redirect(url) {
|
|||
// Support text/{plain,html} by default
|
||||
this.format({
|
||||
text: function(){
|
||||
body = statuses[status] + '. Redirecting to ' + address
|
||||
body = statuses.message[status] + '. Redirecting to ' + address
|
||||
},
|
||||
|
||||
html: function(){
|
||||
var u = escapeHtml(address);
|
||||
body = '<p>' + statuses[status] + '. Redirecting to <a href="' + u + '">' + u + '</a></p>'
|
||||
body = '<p>' + statuses.message[status] + '. Redirecting to <a href="' + u + '">' + u + '</a></p>'
|
||||
},
|
||||
|
||||
default: function(){
|
||||
|
@ -1113,7 +1135,7 @@ function sendfile(res, file, options, callback) {
|
|||
* ability to escape characters that can trigger HTML sniffing.
|
||||
*
|
||||
* @param {*} value
|
||||
* @param {function} replaces
|
||||
* @param {function} replacer
|
||||
* @param {number} spaces
|
||||
* @param {boolean} escape
|
||||
* @returns {string}
|
||||
|
|
|
@ -108,8 +108,8 @@ proto.param = function param(name, fn) {
|
|||
var ret;
|
||||
|
||||
if (name[0] === ':') {
|
||||
deprecate('router.param(' + JSON.stringify(name) + ', fn): Use router.param(' + JSON.stringify(name.substr(1)) + ', fn) instead');
|
||||
name = name.substr(1);
|
||||
deprecate('router.param(' + JSON.stringify(name) + ', fn): Use router.param(' + JSON.stringify(name.slice(1)) + ', fn) instead')
|
||||
name = name.slice(1)
|
||||
}
|
||||
|
||||
for (var i = 0; i < len; ++i) {
|
||||
|
@ -142,6 +142,7 @@ proto.handle = function handle(req, res, out) {
|
|||
var protohost = getProtohost(req.url) || ''
|
||||
var removed = '';
|
||||
var slashAdded = false;
|
||||
var sync = 0
|
||||
var paramcalled = {};
|
||||
|
||||
// store options for OPTIONS request
|
||||
|
@ -180,14 +181,14 @@ proto.handle = function handle(req, res, out) {
|
|||
|
||||
// remove added slash
|
||||
if (slashAdded) {
|
||||
req.url = req.url.substr(1);
|
||||
req.url = req.url.slice(1)
|
||||
slashAdded = false;
|
||||
}
|
||||
|
||||
// restore altered req.url
|
||||
if (removed.length !== 0) {
|
||||
req.baseUrl = parentUrl;
|
||||
req.url = protohost + removed + req.url.substr(protohost.length);
|
||||
req.url = protohost + removed + req.url.slice(protohost.length)
|
||||
removed = '';
|
||||
}
|
||||
|
||||
|
@ -203,6 +204,11 @@ proto.handle = function handle(req, res, out) {
|
|||
return;
|
||||
}
|
||||
|
||||
// max sync stack
|
||||
if (++sync > 100) {
|
||||
return setImmediate(next, err)
|
||||
}
|
||||
|
||||
// get pathname of request
|
||||
var path = getPathname(req);
|
||||
|
||||
|
@ -251,7 +257,6 @@ proto.handle = function handle(req, res, out) {
|
|||
// don't even bother matching route
|
||||
if (!has_method && method !== 'HEAD') {
|
||||
match = false;
|
||||
continue;
|
||||
}
|
||||
}
|
||||
|
||||
|
@ -274,21 +279,21 @@ proto.handle = function handle(req, res, out) {
|
|||
// this should be done for the layer
|
||||
self.process_params(layer, paramcalled, req, res, function (err) {
|
||||
if (err) {
|
||||
return next(layerError || err);
|
||||
next(layerError || err)
|
||||
} else if (route) {
|
||||
layer.handle_request(req, res, next)
|
||||
} else {
|
||||
trim_prefix(layer, layerError, layerPath, path)
|
||||
}
|
||||
|
||||
if (route) {
|
||||
return layer.handle_request(req, res, next);
|
||||
}
|
||||
|
||||
trim_prefix(layer, layerError, layerPath, path);
|
||||
sync = 0
|
||||
});
|
||||
}
|
||||
|
||||
function trim_prefix(layer, layerError, layerPath, path) {
|
||||
if (layerPath.length !== 0) {
|
||||
// Validate path is a prefix match
|
||||
if (layerPath !== path.substr(0, layerPath.length)) {
|
||||
if (layerPath !== path.slice(0, layerPath.length)) {
|
||||
next(layerError)
|
||||
return
|
||||
}
|
||||
|
@ -301,7 +306,7 @@ proto.handle = function handle(req, res, out) {
|
|||
// middleware (.use stuff) needs to have the path stripped
|
||||
debug('trim prefix (%s) from url %s', layerPath, req.url);
|
||||
removed = layerPath;
|
||||
req.url = protohost + req.url.substr(protohost.length + removed.length);
|
||||
req.url = protohost + req.url.slice(protohost.length + removed.length)
|
||||
|
||||
// Ensure leading slash
|
||||
if (!protohost && req.url[0] !== '/') {
|
||||
|
@ -547,10 +552,10 @@ function getProtohost(url) {
|
|||
var pathLength = searchIndex !== -1
|
||||
? searchIndex
|
||||
: url.length
|
||||
var fqdnIndex = url.substr(0, pathLength).indexOf('://')
|
||||
var fqdnIndex = url.slice(0, pathLength).indexOf('://')
|
||||
|
||||
return fqdnIndex !== -1
|
||||
? url.substr(0, url.indexOf('/', 3 + fqdnIndex))
|
||||
? url.substring(0, url.indexOf('/', 3 + fqdnIndex))
|
||||
: undefined
|
||||
}
|
||||
|
||||
|
|
|
@ -98,6 +98,8 @@ Route.prototype._options = function _options() {
|
|||
Route.prototype.dispatch = function dispatch(req, res, done) {
|
||||
var idx = 0;
|
||||
var stack = this.stack;
|
||||
var sync = 0
|
||||
|
||||
if (stack.length === 0) {
|
||||
return done();
|
||||
}
|
||||
|
@ -122,20 +124,27 @@ Route.prototype.dispatch = function dispatch(req, res, done) {
|
|||
return done(err)
|
||||
}
|
||||
|
||||
var layer = stack[idx++];
|
||||
// max sync stack
|
||||
if (++sync > 100) {
|
||||
return setImmediate(next, err)
|
||||
}
|
||||
|
||||
var layer = stack[idx++]
|
||||
|
||||
// end of layers
|
||||
if (!layer) {
|
||||
return done(err);
|
||||
return done(err)
|
||||
}
|
||||
|
||||
if (layer.method && layer.method !== method) {
|
||||
return next(err);
|
||||
}
|
||||
|
||||
if (err) {
|
||||
next(err)
|
||||
} else if (err) {
|
||||
layer.handle_error(err, req, res, next);
|
||||
} else {
|
||||
layer.handle_request(req, res, next);
|
||||
}
|
||||
|
||||
sync = 0
|
||||
}
|
||||
};
|
||||
|
||||
|
|
|
@ -120,6 +120,7 @@ exports.contentDisposition = deprecate.function(contentDisposition,
|
|||
* also includes `.originalIndex` for stable sorting
|
||||
*
|
||||
* @param {String} str
|
||||
* @param {Number} index
|
||||
* @return {Object}
|
||||
* @api private
|
||||
*/
|
||||
|
@ -228,7 +229,8 @@ exports.compileTrust = function(val) {
|
|||
|
||||
if (typeof val === 'string') {
|
||||
// Support comma-separated values
|
||||
val = val.split(/ *, */);
|
||||
val = val.split(',')
|
||||
.map(function (v) { return v.trim() })
|
||||
}
|
||||
|
||||
return proxyaddr.compile(val || []);
|
||||
|
|
|
@ -74,7 +74,7 @@ function View(name, options) {
|
|||
|
||||
if (!opts.engines[this.ext]) {
|
||||
// load engine
|
||||
var mod = this.ext.substr(1)
|
||||
var mod = this.ext.slice(1)
|
||||
debug('require "%s"', mod)
|
||||
|
||||
// default engine export
|
||||
|
|
|
@ -1,7 +1,7 @@
|
|||
{
|
||||
"name": "express",
|
||||
"description": "Fast, unopinionated, minimalist web framework",
|
||||
"version": "4.17.2",
|
||||
"version": "4.18.2",
|
||||
"author": "TJ Holowaychuk <tj@vision-media.ca>",
|
||||
"contributors": [
|
||||
"Aaron Heckmann <aaron.heckmann+github@gmail.com>",
|
||||
|
@ -28,33 +28,34 @@
|
|||
"api"
|
||||
],
|
||||
"dependencies": {
|
||||
"accepts": "~1.3.7",
|
||||
"accepts": "~1.3.8",
|
||||
"array-flatten": "1.1.1",
|
||||
"body-parser": "1.19.1",
|
||||
"body-parser": "1.20.1",
|
||||
"content-disposition": "0.5.4",
|
||||
"content-type": "~1.0.4",
|
||||
"cookie": "0.4.1",
|
||||
"cookie": "0.5.0",
|
||||
"cookie-signature": "1.0.6",
|
||||
"debug": "2.6.9",
|
||||
"depd": "~1.1.2",
|
||||
"depd": "2.0.0",
|
||||
"encodeurl": "~1.0.2",
|
||||
"escape-html": "~1.0.3",
|
||||
"etag": "~1.8.1",
|
||||
"finalhandler": "~1.1.2",
|
||||
"finalhandler": "1.2.0",
|
||||
"fresh": "0.5.2",
|
||||
"http-errors": "2.0.0",
|
||||
"merge-descriptors": "1.0.1",
|
||||
"methods": "~1.1.2",
|
||||
"on-finished": "~2.3.0",
|
||||
"on-finished": "2.4.1",
|
||||
"parseurl": "~1.3.3",
|
||||
"path-to-regexp": "0.1.7",
|
||||
"proxy-addr": "~2.0.7",
|
||||
"qs": "6.9.6",
|
||||
"qs": "6.11.0",
|
||||
"range-parser": "~1.2.1",
|
||||
"safe-buffer": "5.2.1",
|
||||
"send": "0.17.2",
|
||||
"serve-static": "1.14.2",
|
||||
"send": "0.18.0",
|
||||
"serve-static": "1.15.0",
|
||||
"setprototypeof": "1.2.0",
|
||||
"statuses": "~1.5.0",
|
||||
"statuses": "2.0.1",
|
||||
"type-is": "~1.6.18",
|
||||
"utils-merge": "1.0.1",
|
||||
"vary": "~1.1.2"
|
||||
|
@ -64,19 +65,18 @@
|
|||
"connect-redis": "3.4.2",
|
||||
"cookie-parser": "1.4.6",
|
||||
"cookie-session": "2.0.0",
|
||||
"ejs": "3.1.6",
|
||||
"eslint": "7.32.0",
|
||||
"ejs": "3.1.8",
|
||||
"eslint": "8.24.0",
|
||||
"express-session": "1.17.2",
|
||||
"hbs": "4.2.0",
|
||||
"istanbul": "0.4.5",
|
||||
"marked": "0.7.0",
|
||||
"method-override": "3.0.0",
|
||||
"mocha": "9.1.3",
|
||||
"mocha": "10.0.0",
|
||||
"morgan": "1.10.0",
|
||||
"multiparty": "4.2.2",
|
||||
"multiparty": "4.2.3",
|
||||
"nyc": "15.1.0",
|
||||
"pbkdf2-password": "1.2.1",
|
||||
"should": "13.2.3",
|
||||
"supertest": "6.1.6",
|
||||
"supertest": "6.3.0",
|
||||
"vhost": "~3.0.2"
|
||||
},
|
||||
"engines": {
|
||||
|
@ -92,8 +92,8 @@
|
|||
"scripts": {
|
||||
"lint": "eslint .",
|
||||
"test": "mocha --require test/support/env --reporter spec --bail --check-leaks test/ test/acceptance/",
|
||||
"test-ci": "istanbul cover node_modules/mocha/bin/_mocha --report lcovonly -- --require test/support/env --reporter spec --check-leaks test/ test/acceptance/",
|
||||
"test-cov": "istanbul cover node_modules/mocha/bin/_mocha -- --require test/support/env --reporter dot --check-leaks test/ test/acceptance/",
|
||||
"test-ci": "nyc --reporter=lcovonly --reporter=text npm test",
|
||||
"test-cov": "nyc --reporter=html --reporter=text npm test",
|
||||
"test-tap": "mocha --require test/support/env --reporter tap --check-leaks test/ test/acceptance/"
|
||||
}
|
||||
}
|
||||
|
|
|
@ -1,3 +1,11 @@
|
|||
1.2.0 / 2022-03-22
|
||||
==================
|
||||
|
||||
* Remove set content headers that break response
|
||||
* deps: on-finished@2.4.1
|
||||
* deps: statuses@2.0.1
|
||||
- Rename `425 Unordered Collection` to standard `425 Too Early`
|
||||
|
||||
1.1.2 / 2019-05-09
|
||||
==================
|
||||
|
||||
|
|
|
@ -1,6 +1,6 @@
|
|||
(The MIT License)
|
||||
|
||||
Copyright (c) 2014-2017 Douglas Christopher Wilson <doug@somethingdoug.com>
|
||||
Copyright (c) 2014-2022 Douglas Christopher Wilson <doug@somethingdoug.com>
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining
|
||||
a copy of this software and associated documentation files (the
|
||||
|
|
|
@ -3,7 +3,7 @@
|
|||
[![NPM Version][npm-image]][npm-url]
|
||||
[![NPM Downloads][downloads-image]][downloads-url]
|
||||
[![Node.js Version][node-image]][node-url]
|
||||
[![Build Status][travis-image]][travis-url]
|
||||
[![Build Status][github-actions-ci-image]][github-actions-ci-url]
|
||||
[![Test Coverage][coveralls-image]][coveralls-url]
|
||||
|
||||
Node.js function to invoke as the final step to respond to HTTP request.
|
||||
|
@ -20,8 +20,6 @@ $ npm install finalhandler
|
|||
|
||||
## API
|
||||
|
||||
<!-- eslint-disable no-unused-vars -->
|
||||
|
||||
```js
|
||||
var finalhandler = require('finalhandler')
|
||||
```
|
||||
|
@ -31,7 +29,8 @@ var finalhandler = require('finalhandler')
|
|||
Returns function to be invoked as the final step for the given `req` and `res`.
|
||||
This function is to be invoked as `fn(err)`. If `err` is falsy, the handler will
|
||||
write out a 404 response to the `res`. If it is truthy, an error response will
|
||||
be written out to the `res`.
|
||||
be written out to the `res` or `res` will be terminated if a response has already
|
||||
started.
|
||||
|
||||
When an error is written, the following information is added to the response:
|
||||
|
||||
|
@ -140,9 +139,9 @@ function logerror (err) {
|
|||
[npm-url]: https://npmjs.org/package/finalhandler
|
||||
[node-image]: https://img.shields.io/node/v/finalhandler.svg
|
||||
[node-url]: https://nodejs.org/en/download
|
||||
[travis-image]: https://img.shields.io/travis/pillarjs/finalhandler.svg
|
||||
[travis-url]: https://travis-ci.org/pillarjs/finalhandler
|
||||
[coveralls-image]: https://img.shields.io/coveralls/pillarjs/finalhandler.svg
|
||||
[coveralls-url]: https://coveralls.io/r/pillarjs/finalhandler?branch=master
|
||||
[downloads-image]: https://img.shields.io/npm/dm/finalhandler.svg
|
||||
[downloads-url]: https://npmjs.org/package/finalhandler
|
||||
[github-actions-ci-image]: https://img.shields.io/github/workflow/status/pillarjs/finalhandler/ci/master?label=ci
|
||||
[github-actions-ci-url]: https://github.com/jshttp/pillarjs/finalhandler?query=workflow%3Aci
|
||||
|
|
|
@ -0,0 +1,25 @@
|
|||
# Security Policies and Procedures
|
||||
|
||||
## Reporting a Bug
|
||||
|
||||
The `finalhandler` team and community take all security bugs seriously. Thank
|
||||
you for improving the security of Express. We appreciate your efforts and
|
||||
responsible disclosure and will make every effort to acknowledge your
|
||||
contributions.
|
||||
|
||||
Report security bugs by emailing the current owner(s) of `finalhandler`. This
|
||||
information can be found in the npm registry using the command
|
||||
`npm owner ls finalhandler`.
|
||||
If unsure or unable to get the information from the above, open an issue
|
||||
in the [project issue tracker](https://github.com/pillarjs/finalhandler/issues)
|
||||
asking for the current contact information.
|
||||
|
||||
To ensure the timely response to your report, please ensure that the entirety
|
||||
of the report is contained within the email body and not solely behind a web
|
||||
link or an attachment.
|
||||
|
||||
At least one owner will acknowledge your email within 48 hours, and will send a
|
||||
more detailed response within 48 hours indicating the next steps in handling
|
||||
your report. After the initial reply to your report, the owners will
|
||||
endeavor to keep you informed of the progress towards a fix and full
|
||||
announcement, and may ask for additional information or guidance.
|
|
@ -1,6 +1,6 @@
|
|||
/*!
|
||||
* finalhandler
|
||||
* Copyright(c) 2014-2017 Douglas Christopher Wilson
|
||||
* Copyright(c) 2014-2022 Douglas Christopher Wilson
|
||||
* MIT Licensed
|
||||
*/
|
||||
|
||||
|
@ -181,7 +181,7 @@ function getErrorMessage (err, status, env) {
|
|||
}
|
||||
}
|
||||
|
||||
return msg || statuses[status]
|
||||
return msg || statuses.message[status]
|
||||
}
|
||||
|
||||
/**
|
||||
|
@ -276,7 +276,12 @@ function send (req, res, status, headers, message) {
|
|||
|
||||
// response status
|
||||
res.statusCode = status
|
||||
res.statusMessage = statuses[status]
|
||||
res.statusMessage = statuses.message[status]
|
||||
|
||||
// remove any content headers
|
||||
res.removeHeader('Content-Encoding')
|
||||
res.removeHeader('Content-Language')
|
||||
res.removeHeader('Content-Range')
|
||||
|
||||
// response headers
|
||||
setHeaders(res, headers)
|
||||
|
|
|
@ -1,7 +1,7 @@
|
|||
{
|
||||
"name": "finalhandler",
|
||||
"description": "Node.js final http responder",
|
||||
"version": "1.1.2",
|
||||
"version": "1.2.0",
|
||||
"author": "Douglas Christopher Wilson <doug@somethingdoug.com>",
|
||||
"license": "MIT",
|
||||
"repository": "pillarjs/finalhandler",
|
||||
|
@ -9,37 +9,38 @@
|
|||
"debug": "2.6.9",
|
||||
"encodeurl": "~1.0.2",
|
||||
"escape-html": "~1.0.3",
|
||||
"on-finished": "~2.3.0",
|
||||
"on-finished": "2.4.1",
|
||||
"parseurl": "~1.3.3",
|
||||
"statuses": "~1.5.0",
|
||||
"statuses": "2.0.1",
|
||||
"unpipe": "~1.0.0"
|
||||
},
|
||||
"devDependencies": {
|
||||
"eslint": "5.16.0",
|
||||
"eslint-config-standard": "12.0.0",
|
||||
"eslint-plugin-import": "2.17.2",
|
||||
"eslint-plugin-markdown": "1.0.0",
|
||||
"eslint-plugin-node": "8.0.1",
|
||||
"eslint-plugin-promise": "4.1.1",
|
||||
"eslint-plugin-standard": "4.0.0",
|
||||
"istanbul": "0.4.5",
|
||||
"mocha": "6.1.4",
|
||||
"eslint": "7.32.0",
|
||||
"eslint-config-standard": "14.1.1",
|
||||
"eslint-plugin-import": "2.25.4",
|
||||
"eslint-plugin-markdown": "2.2.1",
|
||||
"eslint-plugin-node": "11.1.0",
|
||||
"eslint-plugin-promise": "5.2.0",
|
||||
"eslint-plugin-standard": "4.1.0",
|
||||
"mocha": "9.2.2",
|
||||
"nyc": "15.1.0",
|
||||
"readable-stream": "2.3.6",
|
||||
"safe-buffer": "5.1.2",
|
||||
"supertest": "4.0.2"
|
||||
"safe-buffer": "5.2.1",
|
||||
"supertest": "6.2.2"
|
||||
},
|
||||
"files": [
|
||||
"LICENSE",
|
||||
"HISTORY.md",
|
||||
"SECURITY.md",
|
||||
"index.js"
|
||||
],
|
||||
"engines": {
|
||||
"node": ">= 0.8"
|
||||
},
|
||||
"scripts": {
|
||||
"lint": "eslint --plugin markdown --ext js,md .",
|
||||
"lint": "eslint .",
|
||||
"test": "mocha --reporter spec --bail --check-leaks test/",
|
||||
"test-ci": "istanbul cover node_modules/mocha/bin/_mocha --report lcovonly -- --reporter spec --check-leaks test/",
|
||||
"test-cov": "istanbul cover node_modules/mocha/bin/_mocha -- --reporter dot --check-leaks test/"
|
||||
"test-ci": "nyc --reporter=lcovonly --reporter=text npm test",
|
||||
"test-cov": "nyc --reporter=html --reporter=text npm test"
|
||||
}
|
||||
}
|
||||
|
|
|
@ -0,0 +1,20 @@
|
|||
root = true
|
||||
|
||||
[*]
|
||||
indent_style = tab
|
||||
indent_size = 4
|
||||
end_of_line = lf
|
||||
charset = utf-8
|
||||
trim_trailing_whitespace = true
|
||||
insert_final_newline = true
|
||||
max_line_length = 120
|
||||
|
||||
[CHANGELOG.md]
|
||||
indent_style = space
|
||||
indent_size = 2
|
||||
|
||||
[*.json]
|
||||
max_line_length = off
|
||||
|
||||
[Makefile]
|
||||
max_line_length = off
|
|
@ -0,0 +1,15 @@
|
|||
{
|
||||
"root": true,
|
||||
|
||||
"extends": "@ljharb",
|
||||
|
||||
"rules": {
|
||||
"func-name-matching": 0,
|
||||
"indent": [2, 4],
|
||||
"max-nested-callbacks": [2, 3],
|
||||
"max-params": [2, 3],
|
||||
"max-statements": [2, 20],
|
||||
"no-new-func": [1],
|
||||
"strict": [0]
|
||||
}
|
||||
}
|
|
@ -0,0 +1,176 @@
|
|||
{
|
||||
"es3": true,
|
||||
|
||||
"additionalRules": [],
|
||||
|
||||
"requireSemicolons": true,
|
||||
|
||||
"disallowMultipleSpaces": true,
|
||||
|
||||
"disallowIdentifierNames": [],
|
||||
|
||||
"requireCurlyBraces": {
|
||||
"allExcept": [],
|
||||
"keywords": ["if", "else", "for", "while", "do", "try", "catch"]
|
||||
},
|
||||
|
||||
"requireSpaceAfterKeywords": ["if", "else", "for", "while", "do", "switch", "return", "try", "catch", "function"],
|
||||
|
||||
"disallowSpaceAfterKeywords": [],
|
||||
|
||||
"disallowSpaceBeforeComma": true,
|
||||
"disallowSpaceAfterComma": false,
|
||||
"disallowSpaceBeforeSemicolon": true,
|
||||
|
||||
"disallowNodeTypes": [
|
||||
"DebuggerStatement",
|
||||
"ForInStatement",
|
||||
"LabeledStatement",
|
||||
"SwitchCase",
|
||||
"SwitchStatement",
|
||||
"WithStatement"
|
||||
],
|
||||
|
||||
"requireObjectKeysOnNewLine": { "allExcept": ["sameLine"] },
|
||||
|
||||
"requireSpacesInAnonymousFunctionExpression": { "beforeOpeningRoundBrace": true, "beforeOpeningCurlyBrace": true },
|
||||
"requireSpacesInNamedFunctionExpression": { "beforeOpeningCurlyBrace": true },
|
||||
"disallowSpacesInNamedFunctionExpression": { "beforeOpeningRoundBrace": true },
|
||||
"requireSpacesInFunctionDeclaration": { "beforeOpeningCurlyBrace": true },
|
||||
"disallowSpacesInFunctionDeclaration": { "beforeOpeningRoundBrace": true },
|
||||
|
||||
"requireSpaceBetweenArguments": true,
|
||||
|
||||
"disallowSpacesInsideParentheses": true,
|
||||
|
||||
"disallowSpacesInsideArrayBrackets": true,
|
||||
|
||||
"disallowQuotedKeysInObjects": { "allExcept": ["reserved"] },
|
||||
|
||||
"disallowSpaceAfterObjectKeys": true,
|
||||
|
||||
"requireCommaBeforeLineBreak": true,
|
||||
|
||||
"disallowSpaceAfterPrefixUnaryOperators": ["++", "--", "+", "-", "~", "!"],
|
||||
"requireSpaceAfterPrefixUnaryOperators": [],
|
||||
|
||||
"disallowSpaceBeforePostfixUnaryOperators": ["++", "--"],
|
||||
"requireSpaceBeforePostfixUnaryOperators": [],
|
||||
|
||||
"disallowSpaceBeforeBinaryOperators": [],
|
||||
"requireSpaceBeforeBinaryOperators": ["+", "-", "/", "*", "=", "==", "===", "!=", "!=="],
|
||||
|
||||
"requireSpaceAfterBinaryOperators": ["+", "-", "/", "*", "=", "==", "===", "!=", "!=="],
|
||||
"disallowSpaceAfterBinaryOperators": [],
|
||||
|
||||
"disallowImplicitTypeConversion": ["binary", "string"],
|
||||
|
||||
"disallowKeywords": ["with", "eval"],
|
||||
|
||||
"requireKeywordsOnNewLine": [],
|
||||
"disallowKeywordsOnNewLine": ["else"],
|
||||
|
||||
"requireLineFeedAtFileEnd": true,
|
||||
|
||||
"disallowTrailingWhitespace": true,
|
||||
|
||||
"disallowTrailingComma": true,
|
||||
|
||||
"excludeFiles": ["node_modules/**", "vendor/**"],
|
||||
|
||||
"disallowMultipleLineStrings": true,
|
||||
|
||||
"requireDotNotation": { "allExcept": ["keywords"] },
|
||||
|
||||
"requireParenthesesAroundIIFE": true,
|
||||
|
||||
"validateLineBreaks": "LF",
|
||||
|
||||
"validateQuoteMarks": {
|
||||
"escape": true,
|
||||
"mark": "'"
|
||||
},
|
||||
|
||||
"disallowOperatorBeforeLineBreak": [],
|
||||
|
||||
"requireSpaceBeforeKeywords": [
|
||||
"do",
|
||||
"for",
|
||||
"if",
|
||||
"else",
|
||||
"switch",
|
||||
"case",
|
||||
"try",
|
||||
"catch",
|
||||
"finally",
|
||||
"while",
|
||||
"with",
|
||||
"return"
|
||||
],
|
||||
|
||||
"validateAlignedFunctionParameters": {
|
||||
"lineBreakAfterOpeningBraces": true,
|
||||
"lineBreakBeforeClosingBraces": true
|
||||
},
|
||||
|
||||
"requirePaddingNewLinesBeforeExport": true,
|
||||
|
||||
"validateNewlineAfterArrayElements": {
|
||||
"maximum": 8
|
||||
},
|
||||
|
||||
"requirePaddingNewLinesAfterUseStrict": true,
|
||||
|
||||
"disallowArrowFunctions": true,
|
||||
|
||||
"disallowMultiLineTernary": true,
|
||||
|
||||
"validateOrderInObjectKeys": "asc-insensitive",
|
||||
|
||||
"disallowIdenticalDestructuringNames": true,
|
||||
|
||||
"disallowNestedTernaries": { "maxLevel": 1 },
|
||||
|
||||
"requireSpaceAfterComma": { "allExcept": ["trailing"] },
|
||||
"requireAlignedMultilineParams": false,
|
||||
|
||||
"requireSpacesInGenerator": {
|
||||
"afterStar": true
|
||||
},
|
||||
|
||||
"disallowSpacesInGenerator": {
|
||||
"beforeStar": true
|
||||
},
|
||||
|
||||
"disallowVar": false,
|
||||
|
||||
"requireArrayDestructuring": false,
|
||||
|
||||
"requireEnhancedObjectLiterals": false,
|
||||
|
||||
"requireObjectDestructuring": false,
|
||||
|
||||
"requireEarlyReturn": false,
|
||||
|
||||
"requireCapitalizedConstructorsNew": {
|
||||
"allExcept": ["Function", "String", "Object", "Symbol", "Number", "Date", "RegExp", "Error", "Boolean", "Array"]
|
||||
},
|
||||
|
||||
"requireImportAlphabetized": false,
|
||||
|
||||
"requireSpaceBeforeObjectValues": true,
|
||||
"requireSpaceBeforeDestructuredValues": true,
|
||||
|
||||
"disallowSpacesInsideTemplateStringPlaceholders": true,
|
||||
|
||||
"disallowArrayDestructuringReturn": false,
|
||||
|
||||
"requireNewlineBeforeSingleStatementsInIf": false,
|
||||
|
||||
"disallowUnusedVariables": true,
|
||||
|
||||
"requireSpacesInsideImportedObjectBraces": true,
|
||||
|
||||
"requireUseStrict": true
|
||||
}
|
||||
|
|
@ -0,0 +1,22 @@
|
|||
# gitignore
|
||||
.DS_Store
|
||||
.monitor
|
||||
.*.swp
|
||||
.nodemonignore
|
||||
releases
|
||||
*.log
|
||||
*.err
|
||||
fleet.json
|
||||
public/browserify
|
||||
bin/*.json
|
||||
.bin
|
||||
build
|
||||
compile
|
||||
.lock-wscript
|
||||
coverage
|
||||
node_modules
|
||||
|
||||
# Only apps should have lockfiles
|
||||
npm-shrinkwrap.json
|
||||
package-lock.json
|
||||
yarn.lock
|
|
@ -0,0 +1,168 @@
|
|||
language: node_js
|
||||
os:
|
||||
- linux
|
||||
node_js:
|
||||
- "8.4"
|
||||
- "7.10"
|
||||
- "6.11"
|
||||
- "5.12"
|
||||
- "4.8"
|
||||
- "iojs-v3.3"
|
||||
- "iojs-v2.5"
|
||||
- "iojs-v1.8"
|
||||
- "0.12"
|
||||
- "0.10"
|
||||
- "0.8"
|
||||
before_install:
|
||||
- 'if [ "${TRAVIS_NODE_VERSION}" = "0.6" ]; then npm install -g npm@1.3 ; elif [ "${TRAVIS_NODE_VERSION}" != "0.9" ]; then case "$(npm --version)" in 1.*) npm install -g npm@1.4.28 ;; 2.*) npm install -g npm@2 ;; esac ; fi'
|
||||
- 'if [ "${TRAVIS_NODE_VERSION}" != "0.6" ] && [ "${TRAVIS_NODE_VERSION}" != "0.9" ]; then if [ "${TRAVIS_NODE_VERSION%${TRAVIS_NODE_VERSION#[0-9]}}" = "0" ] || [ "${TRAVIS_NODE_VERSION:0:4}" = "iojs" ]; then npm install -g npm@4.5 ; else npm install -g npm; fi; fi'
|
||||
install:
|
||||
- 'if [ "${TRAVIS_NODE_VERSION}" = "0.6" ]; then nvm install 0.8 && npm install -g npm@1.3 && npm install -g npm@1.4.28 && npm install -g npm@2 && npm install && nvm use "${TRAVIS_NODE_VERSION}"; else npm install; fi;'
|
||||
script:
|
||||
- 'if [ -n "${PRETEST-}" ]; then npm run pretest ; fi'
|
||||
- 'if [ -n "${POSTTEST-}" ]; then npm run posttest ; fi'
|
||||
- 'if [ -n "${COVERAGE-}" ]; then npm run coverage ; fi'
|
||||
- 'if [ -n "${TEST-}" ]; then npm run tests-only ; fi'
|
||||
sudo: false
|
||||
env:
|
||||
- TEST=true
|
||||
matrix:
|
||||
fast_finish: true
|
||||
include:
|
||||
- node_js: "node"
|
||||
env: PRETEST=true
|
||||
- node_js: "4"
|
||||
env: COVERAGE=true
|
||||
- node_js: "8.3"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "8.2"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "8.1"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "8.0"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "7.9"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "7.8"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "7.7"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "7.6"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "7.5"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "7.4"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "7.3"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "7.2"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "7.1"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "7.0"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "6.10"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "6.9"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "6.8"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "6.7"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "6.6"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "6.5"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "6.4"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "6.3"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "6.2"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "6.1"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "6.0"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "5.11"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "5.10"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "5.9"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "5.8"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "5.7"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "5.6"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "5.5"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "5.4"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "5.3"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "5.2"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "5.1"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "5.0"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "4.7"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "4.6"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "4.5"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "4.4"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "4.3"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "4.2"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "4.1"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "4.0"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "iojs-v3.2"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "iojs-v3.1"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "iojs-v3.0"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "iojs-v2.4"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "iojs-v2.3"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "iojs-v2.2"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "iojs-v2.1"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "iojs-v2.0"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "iojs-v1.7"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "iojs-v1.6"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "iojs-v1.5"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "iojs-v1.4"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "iojs-v1.3"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "iojs-v1.2"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "iojs-v1.1"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "iojs-v1.0"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "0.11"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "0.9"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "0.6"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
- node_js: "0.4"
|
||||
env: TEST=true ALLOW_FAILURE=true
|
||||
allow_failures:
|
||||
- os: osx
|
||||
- env: TEST=true ALLOW_FAILURE=true
|
|
@ -0,0 +1,20 @@
|
|||
Copyright (c) 2013 Raynos.
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
of this software and associated documentation files (the "Software"), to deal
|
||||
in the Software without restriction, including without limitation the rights
|
||||
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
copies of the Software, and to permit persons to whom the Software is
|
||||
furnished to do so, subject to the following conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be included in
|
||||
all copies or substantial portions of the Software.
|
||||
|
||||
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
|
||||
THE SOFTWARE.
|
||||
|
|
@ -0,0 +1,48 @@
|
|||
# function-bind
|
||||
|
||||
<!--
|
||||
[![build status][travis-svg]][travis-url]
|
||||
[![NPM version][npm-badge-svg]][npm-url]
|
||||
[![Coverage Status][5]][6]
|
||||
[![gemnasium Dependency Status][7]][8]
|
||||
[![Dependency status][deps-svg]][deps-url]
|
||||
[![Dev Dependency status][dev-deps-svg]][dev-deps-url]
|
||||
-->
|
||||
|
||||
<!-- [![browser support][11]][12] -->
|
||||
|
||||
Implementation of function.prototype.bind
|
||||
|
||||
## Example
|
||||
|
||||
I mainly do this for unit tests I run on phantomjs.
|
||||
PhantomJS does not have Function.prototype.bind :(
|
||||
|
||||
```js
|
||||
Function.prototype.bind = require("function-bind")
|
||||
```
|
||||
|
||||
## Installation
|
||||
|
||||
`npm install function-bind`
|
||||
|
||||
## Contributors
|
||||
|
||||
- Raynos
|
||||
|
||||
## MIT Licenced
|
||||
|
||||
[travis-svg]: https://travis-ci.org/Raynos/function-bind.svg
|
||||
[travis-url]: https://travis-ci.org/Raynos/function-bind
|
||||
[npm-badge-svg]: https://badge.fury.io/js/function-bind.svg
|
||||
[npm-url]: https://npmjs.org/package/function-bind
|
||||
[5]: https://coveralls.io/repos/Raynos/function-bind/badge.png
|
||||
[6]: https://coveralls.io/r/Raynos/function-bind
|
||||
[7]: https://gemnasium.com/Raynos/function-bind.png
|
||||
[8]: https://gemnasium.com/Raynos/function-bind
|
||||
[deps-svg]: https://david-dm.org/Raynos/function-bind.svg
|
||||
[deps-url]: https://david-dm.org/Raynos/function-bind
|
||||
[dev-deps-svg]: https://david-dm.org/Raynos/function-bind/dev-status.svg
|
||||
[dev-deps-url]: https://david-dm.org/Raynos/function-bind#info=devDependencies
|
||||
[11]: https://ci.testling.com/Raynos/function-bind.png
|
||||
[12]: https://ci.testling.com/Raynos/function-bind
|
|
@ -0,0 +1,52 @@
|
|||
'use strict';
|
||||
|
||||
/* eslint no-invalid-this: 1 */
|
||||
|
||||
var ERROR_MESSAGE = 'Function.prototype.bind called on incompatible ';
|
||||
var slice = Array.prototype.slice;
|
||||
var toStr = Object.prototype.toString;
|
||||
var funcType = '[object Function]';
|
||||
|
||||
module.exports = function bind(that) {
|
||||
var target = this;
|
||||
if (typeof target !== 'function' || toStr.call(target) !== funcType) {
|
||||
throw new TypeError(ERROR_MESSAGE + target);
|
||||
}
|
||||
var args = slice.call(arguments, 1);
|
||||
|
||||
var bound;
|
||||
var binder = function () {
|
||||
if (this instanceof bound) {
|
||||
var result = target.apply(
|
||||
this,
|
||||
args.concat(slice.call(arguments))
|
||||
);
|
||||
if (Object(result) === result) {
|
||||
return result;
|
||||
}
|
||||
return this;
|
||||
} else {
|
||||
return target.apply(
|
||||
that,
|
||||
args.concat(slice.call(arguments))
|
||||
);
|
||||
}
|
||||
};
|
||||
|
||||
var boundLength = Math.max(0, target.length - args.length);
|
||||
var boundArgs = [];
|
||||
for (var i = 0; i < boundLength; i++) {
|
||||
boundArgs.push('$' + i);
|
||||
}
|
||||
|
||||
bound = Function('binder', 'return function (' + boundArgs.join(',') + '){ return binder.apply(this,arguments); }')(binder);
|
||||
|
||||
if (target.prototype) {
|
||||
var Empty = function Empty() {};
|
||||
Empty.prototype = target.prototype;
|
||||
bound.prototype = new Empty();
|
||||
Empty.prototype = null;
|
||||
}
|
||||
|
||||
return bound;
|
||||
};
|
|
@ -0,0 +1,5 @@
|
|||
'use strict';
|
||||
|
||||
var implementation = require('./implementation');
|
||||
|
||||
module.exports = Function.prototype.bind || implementation;
|
|
@ -0,0 +1,63 @@
|
|||
{
|
||||
"name": "function-bind",
|
||||
"version": "1.1.1",
|
||||
"description": "Implementation of Function.prototype.bind",
|
||||
"keywords": [
|
||||
"function",
|
||||
"bind",
|
||||
"shim",
|
||||
"es5"
|
||||
],
|
||||
"author": "Raynos <raynos2@gmail.com>",
|
||||
"repository": "git://github.com/Raynos/function-bind.git",
|
||||
"main": "index",
|
||||
"homepage": "https://github.com/Raynos/function-bind",
|
||||
"contributors": [
|
||||
{
|
||||
"name": "Raynos"
|
||||
},
|
||||
{
|
||||
"name": "Jordan Harband",
|
||||
"url": "https://github.com/ljharb"
|
||||
}
|
||||
],
|
||||
"bugs": {
|
||||
"url": "https://github.com/Raynos/function-bind/issues",
|
||||
"email": "raynos2@gmail.com"
|
||||
},
|
||||
"dependencies": {},
|
||||
"devDependencies": {
|
||||
"@ljharb/eslint-config": "^12.2.1",
|
||||
"covert": "^1.1.0",
|
||||
"eslint": "^4.5.0",
|
||||
"jscs": "^3.0.7",
|
||||
"tape": "^4.8.0"
|
||||
},
|
||||
"license": "MIT",
|
||||
"scripts": {
|
||||
"pretest": "npm run lint",
|
||||
"test": "npm run tests-only",
|
||||
"posttest": "npm run coverage -- --quiet",
|
||||
"tests-only": "node test",
|
||||
"coverage": "covert test/*.js",
|
||||
"lint": "npm run jscs && npm run eslint",
|
||||
"jscs": "jscs *.js */*.js",
|
||||
"eslint": "eslint *.js */*.js"
|
||||
},
|
||||
"testling": {
|
||||
"files": "test/index.js",
|
||||
"browsers": [
|
||||
"ie/8..latest",
|
||||
"firefox/16..latest",
|
||||
"firefox/nightly",
|
||||
"chrome/22..latest",
|
||||
"chrome/canary",
|
||||
"opera/12..latest",
|
||||
"opera/next",
|
||||
"safari/5.1..latest",
|
||||
"ipad/6.0..latest",
|
||||
"iphone/6.0..latest",
|
||||
"android-browser/4.2..latest"
|
||||
]
|
||||
}
|
||||
}
|
|
@ -0,0 +1,9 @@
|
|||
{
|
||||
"rules": {
|
||||
"array-bracket-newline": 0,
|
||||
"array-element-newline": 0,
|
||||
"max-statements-per-line": [2, { "max": 2 }],
|
||||
"no-invalid-this": 0,
|
||||
"no-magic-numbers": 0,
|
||||
}
|
||||
}
|
|
@ -0,0 +1,252 @@
|
|||
// jscs:disable requireUseStrict
|
||||
|
||||
var test = require('tape');
|
||||
|
||||
var functionBind = require('../implementation');
|
||||
var getCurrentContext = function () { return this; };
|
||||
|
||||
test('functionBind is a function', function (t) {
|
||||
t.equal(typeof functionBind, 'function');
|
||||
t.end();
|
||||
});
|
||||
|
||||
test('non-functions', function (t) {
|
||||
var nonFunctions = [true, false, [], {}, 42, 'foo', NaN, /a/g];
|
||||
t.plan(nonFunctions.length);
|
||||
for (var i = 0; i < nonFunctions.length; ++i) {
|
||||
try { functionBind.call(nonFunctions[i]); } catch (ex) {
|
||||
t.ok(ex instanceof TypeError, 'throws when given ' + String(nonFunctions[i]));
|
||||
}
|
||||
}
|
||||
t.end();
|
||||
});
|
||||
|
||||
test('without a context', function (t) {
|
||||
t.test('binds properly', function (st) {
|
||||
var args, context;
|
||||
var namespace = {
|
||||
func: functionBind.call(function () {
|
||||
args = Array.prototype.slice.call(arguments);
|
||||
context = this;
|
||||
})
|
||||
};
|
||||
namespace.func(1, 2, 3);
|
||||
st.deepEqual(args, [1, 2, 3]);
|
||||
st.equal(context, getCurrentContext.call());
|
||||
st.end();
|
||||
});
|
||||
|
||||
t.test('binds properly, and still supplies bound arguments', function (st) {
|
||||
var args, context;
|
||||
var namespace = {
|
||||
func: functionBind.call(function () {
|
||||
args = Array.prototype.slice.call(arguments);
|
||||
context = this;
|
||||
}, undefined, 1, 2, 3)
|
||||
};
|
||||
namespace.func(4, 5, 6);
|
||||
st.deepEqual(args, [1, 2, 3, 4, 5, 6]);
|
||||
st.equal(context, getCurrentContext.call());
|
||||
st.end();
|
||||
});
|
||||
|
||||
t.test('returns properly', function (st) {
|
||||
var args;
|
||||
var namespace = {
|
||||
func: functionBind.call(function () {
|
||||
args = Array.prototype.slice.call(arguments);
|
||||
return this;
|
||||
}, null)
|
||||
};
|
||||
var context = namespace.func(1, 2, 3);
|
||||
st.equal(context, getCurrentContext.call(), 'returned context is namespaced context');
|
||||
st.deepEqual(args, [1, 2, 3], 'passed arguments are correct');
|
||||
st.end();
|
||||
});
|
||||
|
||||
t.test('returns properly with bound arguments', function (st) {
|
||||
var args;
|
||||
var namespace = {
|
||||
func: functionBind.call(function () {
|
||||
args = Array.prototype.slice.call(arguments);
|
||||
return this;
|
||||
}, null, 1, 2, 3)
|
||||
};
|
||||
var context = namespace.func(4, 5, 6);
|
||||
st.equal(context, getCurrentContext.call(), 'returned context is namespaced context');
|
||||
st.deepEqual(args, [1, 2, 3, 4, 5, 6], 'passed arguments are correct');
|
||||
st.end();
|
||||
});
|
||||
|
||||
t.test('called as a constructor', function (st) {
|
||||
var thunkify = function (value) {
|
||||
return function () { return value; };
|
||||
};
|
||||
st.test('returns object value', function (sst) {
|
||||
var expectedReturnValue = [1, 2, 3];
|
||||
var Constructor = functionBind.call(thunkify(expectedReturnValue), null);
|
||||
var result = new Constructor();
|
||||
sst.equal(result, expectedReturnValue);
|
||||
sst.end();
|
||||
});
|
||||
|
||||
st.test('does not return primitive value', function (sst) {
|
||||
var Constructor = functionBind.call(thunkify(42), null);
|
||||
var result = new Constructor();
|
||||
sst.notEqual(result, 42);
|
||||
sst.end();
|
||||
});
|
||||
|
||||
st.test('object from bound constructor is instance of original and bound constructor', function (sst) {
|
||||
var A = function (x) {
|
||||
this.name = x || 'A';
|
||||
};
|
||||
var B = functionBind.call(A, null, 'B');
|
||||
|
||||
var result = new B();
|
||||
sst.ok(result instanceof B, 'result is instance of bound constructor');
|
||||
sst.ok(result instanceof A, 'result is instance of original constructor');
|
||||
sst.end();
|
||||
});
|
||||
|
||||
st.end();
|
||||
});
|
||||
|
||||
t.end();
|
||||
});
|
||||
|
||||
test('with a context', function (t) {
|
||||
t.test('with no bound arguments', function (st) {
|
||||
var args, context;
|
||||
var boundContext = {};
|
||||
var namespace = {
|
||||
func: functionBind.call(function () {
|
||||
args = Array.prototype.slice.call(arguments);
|
||||
context = this;
|
||||
}, boundContext)
|
||||
};
|
||||
namespace.func(1, 2, 3);
|
||||
st.equal(context, boundContext, 'binds a context properly');
|
||||
st.deepEqual(args, [1, 2, 3], 'supplies passed arguments');
|
||||
st.end();
|
||||
});
|
||||
|
||||
t.test('with bound arguments', function (st) {
|
||||
var args, context;
|
||||
var boundContext = {};
|
||||
var namespace = {
|
||||
func: functionBind.call(function () {
|
||||
args = Array.prototype.slice.call(arguments);
|
||||
context = this;
|
||||
}, boundContext, 1, 2, 3)
|
||||
};
|
||||
namespace.func(4, 5, 6);
|
||||
st.equal(context, boundContext, 'binds a context properly');
|
||||
st.deepEqual(args, [1, 2, 3, 4, 5, 6], 'supplies bound and passed arguments');
|
||||
st.end();
|
||||
});
|
||||
|
||||
t.test('returns properly', function (st) {
|
||||
var boundContext = {};
|
||||
var args;
|
||||
var namespace = {
|
||||
func: functionBind.call(function () {
|
||||
args = Array.prototype.slice.call(arguments);
|
||||
return this;
|
||||
}, boundContext)
|
||||
};
|
||||
var context = namespace.func(1, 2, 3);
|
||||
st.equal(context, boundContext, 'returned context is bound context');
|
||||
st.notEqual(context, getCurrentContext.call(), 'returned context is not lexical context');
|
||||
st.deepEqual(args, [1, 2, 3], 'passed arguments are correct');
|
||||
st.end();
|
||||
});
|
||||
|
||||
t.test('returns properly with bound arguments', function (st) {
|
||||
var boundContext = {};
|
||||
var args;
|
||||
var namespace = {
|
||||
func: functionBind.call(function () {
|
||||
args = Array.prototype.slice.call(arguments);
|
||||
return this;
|
||||
}, boundContext, 1, 2, 3)
|
||||
};
|
||||
var context = namespace.func(4, 5, 6);
|
||||
st.equal(context, boundContext, 'returned context is bound context');
|
||||
st.notEqual(context, getCurrentContext.call(), 'returned context is not lexical context');
|
||||
st.deepEqual(args, [1, 2, 3, 4, 5, 6], 'passed arguments are correct');
|
||||
st.end();
|
||||
});
|
||||
|
||||
t.test('passes the correct arguments when called as a constructor', function (st) {
|
||||
var expected = { name: 'Correct' };
|
||||
var namespace = {
|
||||
Func: functionBind.call(function (arg) {
|
||||
return arg;
|
||||
}, { name: 'Incorrect' })
|
||||
};
|
||||
var returned = new namespace.Func(expected);
|
||||
st.equal(returned, expected, 'returns the right arg when called as a constructor');
|
||||
st.end();
|
||||
});
|
||||
|
||||
t.test('has the new instance\'s context when called as a constructor', function (st) {
|
||||
var actualContext;
|
||||
var expectedContext = { foo: 'bar' };
|
||||
var namespace = {
|
||||
Func: functionBind.call(function () {
|
||||
actualContext = this;
|
||||
}, expectedContext)
|
||||
};
|
||||
var result = new namespace.Func();
|
||||
st.equal(result instanceof namespace.Func, true);
|
||||
st.notEqual(actualContext, expectedContext);
|
||||
st.end();
|
||||
});
|
||||
|
||||
t.end();
|
||||
});
|
||||
|
||||
test('bound function length', function (t) {
|
||||
t.test('sets a correct length without thisArg', function (st) {
|
||||
var subject = functionBind.call(function (a, b, c) { return a + b + c; });
|
||||
st.equal(subject.length, 3);
|
||||
st.equal(subject(1, 2, 3), 6);
|
||||
st.end();
|
||||
});
|
||||
|
||||
t.test('sets a correct length with thisArg', function (st) {
|
||||
var subject = functionBind.call(function (a, b, c) { return a + b + c; }, {});
|
||||
st.equal(subject.length, 3);
|
||||
st.equal(subject(1, 2, 3), 6);
|
||||
st.end();
|
||||
});
|
||||
|
||||
t.test('sets a correct length without thisArg and first argument', function (st) {
|
||||
var subject = functionBind.call(function (a, b, c) { return a + b + c; }, undefined, 1);
|
||||
st.equal(subject.length, 2);
|
||||
st.equal(subject(2, 3), 6);
|
||||
st.end();
|
||||
});
|
||||
|
||||
t.test('sets a correct length with thisArg and first argument', function (st) {
|
||||
var subject = functionBind.call(function (a, b, c) { return a + b + c; }, {}, 1);
|
||||
st.equal(subject.length, 2);
|
||||
st.equal(subject(2, 3), 6);
|
||||
st.end();
|
||||
});
|
||||
|
||||
t.test('sets a correct length without thisArg and too many arguments', function (st) {
|
||||
var subject = functionBind.call(function (a, b, c) { return a + b + c; }, undefined, 1, 2, 3, 4);
|
||||
st.equal(subject.length, 0);
|
||||
st.equal(subject(), 6);
|
||||
st.end();
|
||||
});
|
||||
|
||||
t.test('sets a correct length with thisArg and too many arguments', function (st) {
|
||||
var subject = functionBind.call(function (a, b, c) { return a + b + c; }, {}, 1, 2, 3, 4);
|
||||
st.equal(subject.length, 0);
|
||||
st.equal(subject(), 6);
|
||||
st.end();
|
||||
});
|
||||
});
|
|
@ -0,0 +1,37 @@
|
|||
{
|
||||
"root": true,
|
||||
|
||||
"extends": "@ljharb",
|
||||
|
||||
"env": {
|
||||
"es6": true,
|
||||
"es2017": true,
|
||||
"es2020": true,
|
||||
"es2021": true,
|
||||
"es2022": true,
|
||||
},
|
||||
|
||||
"rules": {
|
||||
"array-bracket-newline": 0,
|
||||
"complexity": 0,
|
||||
"eqeqeq": [2, "allow-null"],
|
||||
"func-name-matching": 0,
|
||||
"id-length": 0,
|
||||
"max-lines-per-function": [2, 90],
|
||||
"max-params": [2, 4],
|
||||
"max-statements": 0,
|
||||
"max-statements-per-line": [2, { "max": 2 }],
|
||||
"multiline-comment-style": 0,
|
||||
"no-magic-numbers": 0,
|
||||
"sort-keys": 0,
|
||||
},
|
||||
|
||||
"overrides": [
|
||||
{
|
||||
"files": "test/**",
|
||||
"rules": {
|
||||
"new-cap": 0,
|
||||
},
|
||||
},
|
||||
],
|
||||
}
|
|
@ -0,0 +1,12 @@
|
|||
# These are supported funding model platforms
|
||||
|
||||
github: [ljharb]
|
||||
patreon: # Replace with a single Patreon username
|
||||
open_collective: # Replace with a single Open Collective username
|
||||
ko_fi: # Replace with a single Ko-fi username
|
||||
tidelift: npm/get-intrinsic
|
||||
community_bridge: # Replace with a single Community Bridge project-name e.g., cloud-foundry
|
||||
liberapay: # Replace with a single Liberapay username
|
||||
issuehunt: # Replace with a single IssueHunt username
|
||||
otechie: # Replace with a single Otechie username
|
||||
custom: # Replace with up to 4 custom sponsorship URLs e.g., ['link1', 'link2']
|
|
@ -0,0 +1,9 @@
|
|||
{
|
||||
"all": true,
|
||||
"check-coverage": false,
|
||||
"reporter": ["text-summary", "text", "html", "json"],
|
||||
"exclude": [
|
||||
"coverage",
|
||||
"test"
|
||||
]
|
||||
}
|
|
@ -0,0 +1,98 @@
|
|||
# Changelog
|
||||
|
||||
All notable changes to this project will be documented in this file.
|
||||
|
||||
The format is based on [Keep a Changelog](https://keepachangelog.com/en/1.0.0/)
|
||||
and this project adheres to [Semantic Versioning](https://semver.org/spec/v2.0.0.html).
|
||||
|
||||
## [v1.1.3](https://github.com/ljharb/get-intrinsic/compare/v1.1.2...v1.1.3) - 2022-09-12
|
||||
|
||||
### Commits
|
||||
|
||||
- [Dev Deps] update `es-abstract`, `es-value-fixtures`, `tape` [`07ff291`](https://github.com/ljharb/get-intrinsic/commit/07ff291816406ebe5a12d7f16965bde0942dd688)
|
||||
- [Fix] properly check for % signs [`50ac176`](https://github.com/ljharb/get-intrinsic/commit/50ac1760fe99c227e64eabde76e9c0e44cd881b5)
|
||||
|
||||
## [v1.1.2](https://github.com/ljharb/get-intrinsic/compare/v1.1.1...v1.1.2) - 2022-06-08
|
||||
|
||||
### Fixed
|
||||
|
||||
- [Fix] properly validate against extra % signs [`#16`](https://github.com/ljharb/get-intrinsic/issues/16)
|
||||
|
||||
### Commits
|
||||
|
||||
- [actions] reuse common workflows [`0972547`](https://github.com/ljharb/get-intrinsic/commit/0972547efd0abc863fe4c445a6ca7eb4f8c6901d)
|
||||
- [meta] use `npmignore` to autogenerate an npmignore file [`5ba0b51`](https://github.com/ljharb/get-intrinsic/commit/5ba0b51d8d8d4f1c31d426d74abc0770fd106bad)
|
||||
- [actions] use `node/install` instead of `node/run`; use `codecov` action [`c364492`](https://github.com/ljharb/get-intrinsic/commit/c364492af4af51333e6f81c0bf21fd3d602c3661)
|
||||
- [Dev Deps] update `eslint`, `@ljharb/eslint-config`, `aud`, `auto-changelog`, `es-abstract`, `object-inspect`, `tape` [`dc04dad`](https://github.com/ljharb/get-intrinsic/commit/dc04dad86f6e5608775a2640cb0db5927ae29ed9)
|
||||
- [Dev Deps] update `eslint`, `@ljharb/eslint-config`, `es-abstract`, `object-inspect`, `safe-publish-latest`, `tape` [`1c14059`](https://github.com/ljharb/get-intrinsic/commit/1c1405984e86dd2dc9366c15d8a0294a96a146a5)
|
||||
- [Tests] use `mock-property` [`b396ef0`](https://github.com/ljharb/get-intrinsic/commit/b396ef05bb73b1d699811abd64b0d9b97997fdda)
|
||||
- [Dev Deps] update `eslint`, `@ljharb/eslint-config`, `aud`, `auto-changelog`, `object-inspect`, `tape` [`c2c758d`](https://github.com/ljharb/get-intrinsic/commit/c2c758d3b90af4fef0a76910d8d3c292ec8d1d3e)
|
||||
- [Dev Deps] update `eslint`, `@ljharb/eslint-config`, `aud`, `es-abstract`, `es-value-fixtures`, `object-inspect`, `tape` [`29e3c09`](https://github.com/ljharb/get-intrinsic/commit/29e3c091c2bf3e17099969847e8729d0e46896de)
|
||||
- [actions] update codecov uploader [`8cbc141`](https://github.com/ljharb/get-intrinsic/commit/8cbc1418940d7a8941f3a7985cbc4ac095c5e13d)
|
||||
- [Dev Deps] update `@ljharb/eslint-config`, `es-abstract`, `es-value-fixtures`, `object-inspect`, `tape` [`10b6f5c`](https://github.com/ljharb/get-intrinsic/commit/10b6f5c02593fb3680c581d696ac124e30652932)
|
||||
- [readme] add github actions/codecov badges [`4e25400`](https://github.com/ljharb/get-intrinsic/commit/4e25400d9f51ae9eb059cbe22d9144e70ea214e8)
|
||||
- [Tests] use `for-each` instead of `foreach` [`c05b957`](https://github.com/ljharb/get-intrinsic/commit/c05b957ad9a7bc7721af7cc9e9be1edbfe057496)
|
||||
- [Dev Deps] update `es-abstract` [`29b05ae`](https://github.com/ljharb/get-intrinsic/commit/29b05aec3e7330e9ad0b8e0f685a9112c20cdd97)
|
||||
- [meta] use `prepublishOnly` script for npm 7+ [`95c285d`](https://github.com/ljharb/get-intrinsic/commit/95c285da810516057d3bbfa871176031af38f05d)
|
||||
- [Deps] update `has-symbols` [`593cb4f`](https://github.com/ljharb/get-intrinsic/commit/593cb4fb38e7922e40e42c183f45274b636424cd)
|
||||
- [readme] fix repo URLs [`1c8305b`](https://github.com/ljharb/get-intrinsic/commit/1c8305b5365827c9b6fc785434aac0e1328ff2f5)
|
||||
- [Deps] update `has-symbols` [`c7138b6`](https://github.com/ljharb/get-intrinsic/commit/c7138b6c6d73132d859471fb8c13304e1e7c8b20)
|
||||
- [Dev Deps] remove unused `has-bigints` [`bd63aff`](https://github.com/ljharb/get-intrinsic/commit/bd63aff6ad8f3a986c557fcda2914187bdaab359)
|
||||
|
||||
## [v1.1.1](https://github.com/ljharb/get-intrinsic/compare/v1.1.0...v1.1.1) - 2021-02-03
|
||||
|
||||
### Fixed
|
||||
|
||||
- [meta] export `./package.json` [`#9`](https://github.com/ljharb/get-intrinsic/issues/9)
|
||||
|
||||
### Commits
|
||||
|
||||
- [readme] flesh out the readme; use `evalmd` [`d12f12c`](https://github.com/ljharb/get-intrinsic/commit/d12f12c15345a0a0772cc65a7c64369529abd614)
|
||||
- [eslint] set up proper globals config [`5a8c098`](https://github.com/ljharb/get-intrinsic/commit/5a8c0984e3319d1ac0e64b102f8ec18b64e79f36)
|
||||
- [Dev Deps] update `eslint` [`7b9a5c0`](https://github.com/ljharb/get-intrinsic/commit/7b9a5c0d31a90ca1a1234181c74988fb046701cd)
|
||||
|
||||
## [v1.1.0](https://github.com/ljharb/get-intrinsic/compare/v1.0.2...v1.1.0) - 2021-01-25
|
||||
|
||||
### Fixed
|
||||
|
||||
- [Refactor] delay `Function` eval until syntax-derived values are requested [`#3`](https://github.com/ljharb/get-intrinsic/issues/3)
|
||||
|
||||
### Commits
|
||||
|
||||
- [Tests] migrate tests to Github Actions [`2ab762b`](https://github.com/ljharb/get-intrinsic/commit/2ab762b48164aea8af37a40ba105bbc8246ab8c4)
|
||||
- [meta] do not publish github action workflow files [`5e7108e`](https://github.com/ljharb/get-intrinsic/commit/5e7108e4768b244d48d9567ba4f8a6cab9c65b8e)
|
||||
- [Tests] add some coverage [`01ac7a8`](https://github.com/ljharb/get-intrinsic/commit/01ac7a87ac29738567e8524cd8c9e026b1fa8cb3)
|
||||
- [Dev Deps] update `eslint`, `@ljharb/eslint-config`, `call-bind`, `es-abstract`, `tape`; add `call-bind` [`911b672`](https://github.com/ljharb/get-intrinsic/commit/911b672fbffae433a96924c6ce013585e425f4b7)
|
||||
- [Refactor] rearrange evalled constructors a bit [`7e7e4bf`](https://github.com/ljharb/get-intrinsic/commit/7e7e4bf583f3799c8ac1c6c5e10d2cb553957347)
|
||||
- [meta] add Automatic Rebase and Require Allow Edits workflows [`0199968`](https://github.com/ljharb/get-intrinsic/commit/01999687a263ffce0a3cb011dfbcb761754aedbc)
|
||||
|
||||
## [v1.0.2](https://github.com/ljharb/get-intrinsic/compare/v1.0.1...v1.0.2) - 2020-12-17
|
||||
|
||||
### Commits
|
||||
|
||||
- [Fix] Throw for non‑existent intrinsics [`68f873b`](https://github.com/ljharb/get-intrinsic/commit/68f873b013c732a05ad6f5fc54f697e55515461b)
|
||||
- [Fix] Throw for non‑existent segments in the intrinsic path [`8325dee`](https://github.com/ljharb/get-intrinsic/commit/8325deee43128f3654d3399aa9591741ebe17b21)
|
||||
- [Dev Deps] update `eslint`, `@ljharb/eslint-config`, `aud`, `has-bigints`, `object-inspect` [`0c227a7`](https://github.com/ljharb/get-intrinsic/commit/0c227a7d8b629166f25715fd242553892e458525)
|
||||
- [meta] do not lint coverage output [`70d2419`](https://github.com/ljharb/get-intrinsic/commit/70d24199b620043cd9110fc5f426d214ebe21dc9)
|
||||
|
||||
## [v1.0.1](https://github.com/ljharb/get-intrinsic/compare/v1.0.0...v1.0.1) - 2020-10-30
|
||||
|
||||
### Commits
|
||||
|
||||
- [Tests] gather coverage data on every job [`d1d280d`](https://github.com/ljharb/get-intrinsic/commit/d1d280dec714e3f0519cc877dbcb193057d9cac6)
|
||||
- [Fix] add missing dependencies [`5031771`](https://github.com/ljharb/get-intrinsic/commit/5031771bb1095b38be88ce7c41d5de88718e432e)
|
||||
- [Tests] use `es-value-fixtures` [`af48765`](https://github.com/ljharb/get-intrinsic/commit/af48765a23c5323fb0b6b38dbf00eb5099c7bebc)
|
||||
|
||||
## v1.0.0 - 2020-10-29
|
||||
|
||||
### Commits
|
||||
|
||||
- Implementation [`bbce57c`](https://github.com/ljharb/get-intrinsic/commit/bbce57c6f33d05b2d8d3efa273ceeb3ee01127bb)
|
||||
- Tests [`17b4f0d`](https://github.com/ljharb/get-intrinsic/commit/17b4f0d56dea6b4059b56fc30ef3ee4d9500ebc2)
|
||||
- Initial commit [`3153294`](https://github.com/ljharb/get-intrinsic/commit/31532948de363b0a27dd9fd4649e7b7028ec4b44)
|
||||
- npm init [`fb326c4`](https://github.com/ljharb/get-intrinsic/commit/fb326c4d2817c8419ec31de1295f06bb268a7902)
|
||||
- [meta] add Automatic Rebase and Require Allow Edits workflows [`48862fb`](https://github.com/ljharb/get-intrinsic/commit/48862fb2508c8f6a57968e6d08b7c883afc9d550)
|
||||
- [meta] add `auto-changelog` [`5f28ad0`](https://github.com/ljharb/get-intrinsic/commit/5f28ad019e060a353d8028f9f2591a9cc93074a1)
|
||||
- [meta] add "funding"; create `FUNDING.yml` [`c2bbdde`](https://github.com/ljharb/get-intrinsic/commit/c2bbddeba73a875be61484ee4680b129a6d4e0a1)
|
||||
- [Tests] add `npm run lint` [`0a84b98`](https://github.com/ljharb/get-intrinsic/commit/0a84b98b22b7cf7a748666f705b0003a493c35fd)
|
||||
- Only apps should have lockfiles [`9586c75`](https://github.com/ljharb/get-intrinsic/commit/9586c75866c1ee678e4d5d4dbbdef6997e511b05)
|
|
@ -0,0 +1,21 @@
|
|||
MIT License
|
||||
|
||||
Copyright (c) 2020 Jordan Harband
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
of this software and associated documentation files (the "Software"), to deal
|
||||
in the Software without restriction, including without limitation the rights
|
||||
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
copies of the Software, and to permit persons to whom the Software is
|
||||
furnished to do so, subject to the following conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be included in all
|
||||
copies or substantial portions of the Software.
|
||||
|
||||
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
SOFTWARE.
|
|
@ -0,0 +1,71 @@
|
|||
# get-intrinsic <sup>[![Version Badge][npm-version-svg]][package-url]</sup>
|
||||
|
||||
[![github actions][actions-image]][actions-url]
|
||||
[![coverage][codecov-image]][codecov-url]
|
||||
[![dependency status][deps-svg]][deps-url]
|
||||
[![dev dependency status][dev-deps-svg]][dev-deps-url]
|
||||
[![License][license-image]][license-url]
|
||||
[![Downloads][downloads-image]][downloads-url]
|
||||
|
||||
[![npm badge][npm-badge-png]][package-url]
|
||||
|
||||
Get and robustly cache all JS language-level intrinsics at first require time.
|
||||
|
||||
See the syntax described [in the JS spec](https://tc39.es/ecma262/#sec-well-known-intrinsic-objects) for reference.
|
||||
|
||||
## Example
|
||||
|
||||
```js
|
||||
var GetIntrinsic = require('get-intrinsic');
|
||||
var assert = require('assert');
|
||||
|
||||
// static methods
|
||||
assert.equal(GetIntrinsic('%Math.pow%'), Math.pow);
|
||||
assert.equal(Math.pow(2, 3), 8);
|
||||
assert.equal(GetIntrinsic('%Math.pow%')(2, 3), 8);
|
||||
delete Math.pow;
|
||||
assert.equal(GetIntrinsic('%Math.pow%')(2, 3), 8);
|
||||
|
||||
// instance methods
|
||||
var arr = [1];
|
||||
assert.equal(GetIntrinsic('%Array.prototype.push%'), Array.prototype.push);
|
||||
assert.deepEqual(arr, [1]);
|
||||
|
||||
arr.push(2);
|
||||
assert.deepEqual(arr, [1, 2]);
|
||||
|
||||
GetIntrinsic('%Array.prototype.push%').call(arr, 3);
|
||||
assert.deepEqual(arr, [1, 2, 3]);
|
||||
|
||||
delete Array.prototype.push;
|
||||
GetIntrinsic('%Array.prototype.push%').call(arr, 4);
|
||||
assert.deepEqual(arr, [1, 2, 3, 4]);
|
||||
|
||||
// missing features
|
||||
delete JSON.parse; // to simulate a real intrinsic that is missing in the environment
|
||||
assert.throws(() => GetIntrinsic('%JSON.parse%'));
|
||||
assert.equal(undefined, GetIntrinsic('%JSON.parse%', true));
|
||||
```
|
||||
|
||||
## Tests
|
||||
Simply clone the repo, `npm install`, and run `npm test`
|
||||
|
||||
## Security
|
||||
|
||||
Please email [@ljharb](https://github.com/ljharb) or see https://tidelift.com/security if you have a potential security vulnerability to report.
|
||||
|
||||
[package-url]: https://npmjs.org/package/get-intrinsic
|
||||
[npm-version-svg]: https://versionbadg.es/ljharb/get-intrinsic.svg
|
||||
[deps-svg]: https://david-dm.org/ljharb/get-intrinsic.svg
|
||||
[deps-url]: https://david-dm.org/ljharb/get-intrinsic
|
||||
[dev-deps-svg]: https://david-dm.org/ljharb/get-intrinsic/dev-status.svg
|
||||
[dev-deps-url]: https://david-dm.org/ljharb/get-intrinsic#info=devDependencies
|
||||
[npm-badge-png]: https://nodei.co/npm/get-intrinsic.png?downloads=true&stars=true
|
||||
[license-image]: https://img.shields.io/npm/l/get-intrinsic.svg
|
||||
[license-url]: LICENSE
|
||||
[downloads-image]: https://img.shields.io/npm/dm/get-intrinsic.svg
|
||||
[downloads-url]: https://npm-stat.com/charts.html?package=get-intrinsic
|
||||
[codecov-image]: https://codecov.io/gh/ljharb/get-intrinsic/branch/main/graphs/badge.svg
|
||||
[codecov-url]: https://app.codecov.io/gh/ljharb/get-intrinsic/
|
||||
[actions-image]: https://img.shields.io/endpoint?url=https://github-actions-badge-u3jn4tfpocch.runkit.sh/ljharb/get-intrinsic
|
||||
[actions-url]: https://github.com/ljharb/get-intrinsic/actions
|
|
@ -0,0 +1,334 @@
|
|||
'use strict';
|
||||
|
||||
var undefined;
|
||||
|
||||
var $SyntaxError = SyntaxError;
|
||||
var $Function = Function;
|
||||
var $TypeError = TypeError;
|
||||
|
||||
// eslint-disable-next-line consistent-return
|
||||
var getEvalledConstructor = function (expressionSyntax) {
|
||||
try {
|
||||
return $Function('"use strict"; return (' + expressionSyntax + ').constructor;')();
|
||||
} catch (e) {}
|
||||
};
|
||||
|
||||
var $gOPD = Object.getOwnPropertyDescriptor;
|
||||
if ($gOPD) {
|
||||
try {
|
||||
$gOPD({}, '');
|
||||
} catch (e) {
|
||||
$gOPD = null; // this is IE 8, which has a broken gOPD
|
||||
}
|
||||
}
|
||||
|
||||
var throwTypeError = function () {
|
||||
throw new $TypeError();
|
||||
};
|
||||
var ThrowTypeError = $gOPD
|
||||
? (function () {
|
||||
try {
|
||||
// eslint-disable-next-line no-unused-expressions, no-caller, no-restricted-properties
|
||||
arguments.callee; // IE 8 does not throw here
|
||||
return throwTypeError;
|
||||
} catch (calleeThrows) {
|
||||
try {
|
||||
// IE 8 throws on Object.getOwnPropertyDescriptor(arguments, '')
|
||||
return $gOPD(arguments, 'callee').get;
|
||||
} catch (gOPDthrows) {
|
||||
return throwTypeError;
|
||||
}
|
||||
}
|
||||
}())
|
||||
: throwTypeError;
|
||||
|
||||
var hasSymbols = require('has-symbols')();
|
||||
|
||||
var getProto = Object.getPrototypeOf || function (x) { return x.__proto__; }; // eslint-disable-line no-proto
|
||||
|
||||
var needsEval = {};
|
||||
|
||||
var TypedArray = typeof Uint8Array === 'undefined' ? undefined : getProto(Uint8Array);
|
||||
|
||||
var INTRINSICS = {
|
||||
'%AggregateError%': typeof AggregateError === 'undefined' ? undefined : AggregateError,
|
||||
'%Array%': Array,
|
||||
'%ArrayBuffer%': typeof ArrayBuffer === 'undefined' ? undefined : ArrayBuffer,
|
||||
'%ArrayIteratorPrototype%': hasSymbols ? getProto([][Symbol.iterator]()) : undefined,
|
||||
'%AsyncFromSyncIteratorPrototype%': undefined,
|
||||
'%AsyncFunction%': needsEval,
|
||||
'%AsyncGenerator%': needsEval,
|
||||
'%AsyncGeneratorFunction%': needsEval,
|
||||
'%AsyncIteratorPrototype%': needsEval,
|
||||
'%Atomics%': typeof Atomics === 'undefined' ? undefined : Atomics,
|
||||
'%BigInt%': typeof BigInt === 'undefined' ? undefined : BigInt,
|
||||
'%Boolean%': Boolean,
|
||||
'%DataView%': typeof DataView === 'undefined' ? undefined : DataView,
|
||||
'%Date%': Date,
|
||||
'%decodeURI%': decodeURI,
|
||||
'%decodeURIComponent%': decodeURIComponent,
|
||||
'%encodeURI%': encodeURI,
|
||||
'%encodeURIComponent%': encodeURIComponent,
|
||||
'%Error%': Error,
|
||||
'%eval%': eval, // eslint-disable-line no-eval
|
||||
'%EvalError%': EvalError,
|
||||
'%Float32Array%': typeof Float32Array === 'undefined' ? undefined : Float32Array,
|
||||
'%Float64Array%': typeof Float64Array === 'undefined' ? undefined : Float64Array,
|
||||
'%FinalizationRegistry%': typeof FinalizationRegistry === 'undefined' ? undefined : FinalizationRegistry,
|
||||
'%Function%': $Function,
|
||||
'%GeneratorFunction%': needsEval,
|
||||
'%Int8Array%': typeof Int8Array === 'undefined' ? undefined : Int8Array,
|
||||
'%Int16Array%': typeof Int16Array === 'undefined' ? undefined : Int16Array,
|
||||
'%Int32Array%': typeof Int32Array === 'undefined' ? undefined : Int32Array,
|
||||
'%isFinite%': isFinite,
|
||||
'%isNaN%': isNaN,
|
||||
'%IteratorPrototype%': hasSymbols ? getProto(getProto([][Symbol.iterator]())) : undefined,
|
||||
'%JSON%': typeof JSON === 'object' ? JSON : undefined,
|
||||
'%Map%': typeof Map === 'undefined' ? undefined : Map,
|
||||
'%MapIteratorPrototype%': typeof Map === 'undefined' || !hasSymbols ? undefined : getProto(new Map()[Symbol.iterator]()),
|
||||
'%Math%': Math,
|
||||
'%Number%': Number,
|
||||
'%Object%': Object,
|
||||
'%parseFloat%': parseFloat,
|
||||
'%parseInt%': parseInt,
|
||||
'%Promise%': typeof Promise === 'undefined' ? undefined : Promise,
|
||||
'%Proxy%': typeof Proxy === 'undefined' ? undefined : Proxy,
|
||||
'%RangeError%': RangeError,
|
||||
'%ReferenceError%': ReferenceError,
|
||||
'%Reflect%': typeof Reflect === 'undefined' ? undefined : Reflect,
|
||||
'%RegExp%': RegExp,
|
||||
'%Set%': typeof Set === 'undefined' ? undefined : Set,
|
||||
'%SetIteratorPrototype%': typeof Set === 'undefined' || !hasSymbols ? undefined : getProto(new Set()[Symbol.iterator]()),
|
||||
'%SharedArrayBuffer%': typeof SharedArrayBuffer === 'undefined' ? undefined : SharedArrayBuffer,
|
||||
'%String%': String,
|
||||
'%StringIteratorPrototype%': hasSymbols ? getProto(''[Symbol.iterator]()) : undefined,
|
||||
'%Symbol%': hasSymbols ? Symbol : undefined,
|
||||
'%SyntaxError%': $SyntaxError,
|
||||
'%ThrowTypeError%': ThrowTypeError,
|
||||
'%TypedArray%': TypedArray,
|
||||
'%TypeError%': $TypeError,
|
||||
'%Uint8Array%': typeof Uint8Array === 'undefined' ? undefined : Uint8Array,
|
||||
'%Uint8ClampedArray%': typeof Uint8ClampedArray === 'undefined' ? undefined : Uint8ClampedArray,
|
||||
'%Uint16Array%': typeof Uint16Array === 'undefined' ? undefined : Uint16Array,
|
||||
'%Uint32Array%': typeof Uint32Array === 'undefined' ? undefined : Uint32Array,
|
||||
'%URIError%': URIError,
|
||||
'%WeakMap%': typeof WeakMap === 'undefined' ? undefined : WeakMap,
|
||||
'%WeakRef%': typeof WeakRef === 'undefined' ? undefined : WeakRef,
|
||||
'%WeakSet%': typeof WeakSet === 'undefined' ? undefined : WeakSet
|
||||
};
|
||||
|
||||
var doEval = function doEval(name) {
|
||||
var value;
|
||||
if (name === '%AsyncFunction%') {
|
||||
value = getEvalledConstructor('async function () {}');
|
||||
} else if (name === '%GeneratorFunction%') {
|
||||
value = getEvalledConstructor('function* () {}');
|
||||
} else if (name === '%AsyncGeneratorFunction%') {
|
||||
value = getEvalledConstructor('async function* () {}');
|
||||
} else if (name === '%AsyncGenerator%') {
|
||||
var fn = doEval('%AsyncGeneratorFunction%');
|
||||
if (fn) {
|
||||
value = fn.prototype;
|
||||
}
|
||||
} else if (name === '%AsyncIteratorPrototype%') {
|
||||
var gen = doEval('%AsyncGenerator%');
|
||||
if (gen) {
|
||||
value = getProto(gen.prototype);
|
||||
}
|
||||
}
|
||||
|
||||
INTRINSICS[name] = value;
|
||||
|
||||
return value;
|
||||
};
|
||||
|
||||
var LEGACY_ALIASES = {
|
||||
'%ArrayBufferPrototype%': ['ArrayBuffer', 'prototype'],
|
||||
'%ArrayPrototype%': ['Array', 'prototype'],
|
||||
'%ArrayProto_entries%': ['Array', 'prototype', 'entries'],
|
||||
'%ArrayProto_forEach%': ['Array', 'prototype', 'forEach'],
|
||||
'%ArrayProto_keys%': ['Array', 'prototype', 'keys'],
|
||||
'%ArrayProto_values%': ['Array', 'prototype', 'values'],
|
||||
'%AsyncFunctionPrototype%': ['AsyncFunction', 'prototype'],
|
||||
'%AsyncGenerator%': ['AsyncGeneratorFunction', 'prototype'],
|
||||
'%AsyncGeneratorPrototype%': ['AsyncGeneratorFunction', 'prototype', 'prototype'],
|
||||
'%BooleanPrototype%': ['Boolean', 'prototype'],
|
||||
'%DataViewPrototype%': ['DataView', 'prototype'],
|
||||
'%DatePrototype%': ['Date', 'prototype'],
|
||||
'%ErrorPrototype%': ['Error', 'prototype'],
|
||||
'%EvalErrorPrototype%': ['EvalError', 'prototype'],
|
||||
'%Float32ArrayPrototype%': ['Float32Array', 'prototype'],
|
||||
'%Float64ArrayPrototype%': ['Float64Array', 'prototype'],
|
||||
'%FunctionPrototype%': ['Function', 'prototype'],
|
||||
'%Generator%': ['GeneratorFunction', 'prototype'],
|
||||
'%GeneratorPrototype%': ['GeneratorFunction', 'prototype', 'prototype'],
|
||||
'%Int8ArrayPrototype%': ['Int8Array', 'prototype'],
|
||||
'%Int16ArrayPrototype%': ['Int16Array', 'prototype'],
|
||||
'%Int32ArrayPrototype%': ['Int32Array', 'prototype'],
|
||||
'%JSONParse%': ['JSON', 'parse'],
|
||||
'%JSONStringify%': ['JSON', 'stringify'],
|
||||
'%MapPrototype%': ['Map', 'prototype'],
|
||||
'%NumberPrototype%': ['Number', 'prototype'],
|
||||
'%ObjectPrototype%': ['Object', 'prototype'],
|
||||
'%ObjProto_toString%': ['Object', 'prototype', 'toString'],
|
||||
'%ObjProto_valueOf%': ['Object', 'prototype', 'valueOf'],
|
||||
'%PromisePrototype%': ['Promise', 'prototype'],
|
||||
'%PromiseProto_then%': ['Promise', 'prototype', 'then'],
|
||||
'%Promise_all%': ['Promise', 'all'],
|
||||
'%Promise_reject%': ['Promise', 'reject'],
|
||||
'%Promise_resolve%': ['Promise', 'resolve'],
|
||||
'%RangeErrorPrototype%': ['RangeError', 'prototype'],
|
||||
'%ReferenceErrorPrototype%': ['ReferenceError', 'prototype'],
|
||||
'%RegExpPrototype%': ['RegExp', 'prototype'],
|
||||
'%SetPrototype%': ['Set', 'prototype'],
|
||||
'%SharedArrayBufferPrototype%': ['SharedArrayBuffer', 'prototype'],
|
||||
'%StringPrototype%': ['String', 'prototype'],
|
||||
'%SymbolPrototype%': ['Symbol', 'prototype'],
|
||||
'%SyntaxErrorPrototype%': ['SyntaxError', 'prototype'],
|
||||
'%TypedArrayPrototype%': ['TypedArray', 'prototype'],
|
||||
'%TypeErrorPrototype%': ['TypeError', 'prototype'],
|
||||
'%Uint8ArrayPrototype%': ['Uint8Array', 'prototype'],
|
||||
'%Uint8ClampedArrayPrototype%': ['Uint8ClampedArray', 'prototype'],
|
||||
'%Uint16ArrayPrototype%': ['Uint16Array', 'prototype'],
|
||||
'%Uint32ArrayPrototype%': ['Uint32Array', 'prototype'],
|
||||
'%URIErrorPrototype%': ['URIError', 'prototype'],
|
||||
'%WeakMapPrototype%': ['WeakMap', 'prototype'],
|
||||
'%WeakSetPrototype%': ['WeakSet', 'prototype']
|
||||
};
|
||||
|
||||
var bind = require('function-bind');
|
||||
var hasOwn = require('has');
|
||||
var $concat = bind.call(Function.call, Array.prototype.concat);
|
||||
var $spliceApply = bind.call(Function.apply, Array.prototype.splice);
|
||||
var $replace = bind.call(Function.call, String.prototype.replace);
|
||||
var $strSlice = bind.call(Function.call, String.prototype.slice);
|
||||
var $exec = bind.call(Function.call, RegExp.prototype.exec);
|
||||
|
||||
/* adapted from https://github.com/lodash/lodash/blob/4.17.15/dist/lodash.js#L6735-L6744 */
|
||||
var rePropName = /[^%.[\]]+|\[(?:(-?\d+(?:\.\d+)?)|(["'])((?:(?!\2)[^\\]|\\.)*?)\2)\]|(?=(?:\.|\[\])(?:\.|\[\]|%$))/g;
|
||||
var reEscapeChar = /\\(\\)?/g; /** Used to match backslashes in property paths. */
|
||||
var stringToPath = function stringToPath(string) {
|
||||
var first = $strSlice(string, 0, 1);
|
||||
var last = $strSlice(string, -1);
|
||||
if (first === '%' && last !== '%') {
|
||||
throw new $SyntaxError('invalid intrinsic syntax, expected closing `%`');
|
||||
} else if (last === '%' && first !== '%') {
|
||||
throw new $SyntaxError('invalid intrinsic syntax, expected opening `%`');
|
||||
}
|
||||
var result = [];
|
||||
$replace(string, rePropName, function (match, number, quote, subString) {
|
||||
result[result.length] = quote ? $replace(subString, reEscapeChar, '$1') : number || match;
|
||||
});
|
||||
return result;
|
||||
};
|
||||
/* end adaptation */
|
||||
|
||||
var getBaseIntrinsic = function getBaseIntrinsic(name, allowMissing) {
|
||||
var intrinsicName = name;
|
||||
var alias;
|
||||
if (hasOwn(LEGACY_ALIASES, intrinsicName)) {
|
||||
alias = LEGACY_ALIASES[intrinsicName];
|
||||
intrinsicName = '%' + alias[0] + '%';
|
||||
}
|
||||
|
||||
if (hasOwn(INTRINSICS, intrinsicName)) {
|
||||
var value = INTRINSICS[intrinsicName];
|
||||
if (value === needsEval) {
|
||||
value = doEval(intrinsicName);
|
||||
}
|
||||
if (typeof value === 'undefined' && !allowMissing) {
|
||||
throw new $TypeError('intrinsic ' + name + ' exists, but is not available. Please file an issue!');
|
||||
}
|
||||
|
||||
return {
|
||||
alias: alias,
|
||||
name: intrinsicName,
|
||||
value: value
|
||||
};
|
||||
}
|
||||
|
||||
throw new $SyntaxError('intrinsic ' + name + ' does not exist!');
|
||||
};
|
||||
|
||||
module.exports = function GetIntrinsic(name, allowMissing) {
|
||||
if (typeof name !== 'string' || name.length === 0) {
|
||||
throw new $TypeError('intrinsic name must be a non-empty string');
|
||||
}
|
||||
if (arguments.length > 1 && typeof allowMissing !== 'boolean') {
|
||||
throw new $TypeError('"allowMissing" argument must be a boolean');
|
||||
}
|
||||
|
||||
if ($exec(/^%?[^%]*%?$/, name) === null) {
|
||||
throw new $SyntaxError('`%` may not be present anywhere but at the beginning and end of the intrinsic name');
|
||||
}
|
||||
var parts = stringToPath(name);
|
||||
var intrinsicBaseName = parts.length > 0 ? parts[0] : '';
|
||||
|
||||
var intrinsic = getBaseIntrinsic('%' + intrinsicBaseName + '%', allowMissing);
|
||||
var intrinsicRealName = intrinsic.name;
|
||||
var value = intrinsic.value;
|
||||
var skipFurtherCaching = false;
|
||||
|
||||
var alias = intrinsic.alias;
|
||||
if (alias) {
|
||||
intrinsicBaseName = alias[0];
|
||||
$spliceApply(parts, $concat([0, 1], alias));
|
||||
}
|
||||
|
||||
for (var i = 1, isOwn = true; i < parts.length; i += 1) {
|
||||
var part = parts[i];
|
||||
var first = $strSlice(part, 0, 1);
|
||||
var last = $strSlice(part, -1);
|
||||
if (
|
||||
(
|
||||
(first === '"' || first === "'" || first === '`')
|
||||
|| (last === '"' || last === "'" || last === '`')
|
||||
)
|
||||
&& first !== last
|
||||
) {
|
||||
throw new $SyntaxError('property names with quotes must have matching quotes');
|
||||
}
|
||||
if (part === 'constructor' || !isOwn) {
|
||||
skipFurtherCaching = true;
|
||||
}
|
||||
|
||||
intrinsicBaseName += '.' + part;
|
||||
intrinsicRealName = '%' + intrinsicBaseName + '%';
|
||||
|
||||
if (hasOwn(INTRINSICS, intrinsicRealName)) {
|
||||
value = INTRINSICS[intrinsicRealName];
|
||||
} else if (value != null) {
|
||||
if (!(part in value)) {
|
||||
if (!allowMissing) {
|
||||
throw new $TypeError('base intrinsic for ' + name + ' exists, but the property is not available.');
|
||||
}
|
||||
return void undefined;
|
||||
}
|
||||
if ($gOPD && (i + 1) >= parts.length) {
|
||||
var desc = $gOPD(value, part);
|
||||
isOwn = !!desc;
|
||||
|
||||
// By convention, when a data property is converted to an accessor
|
||||
// property to emulate a data property that does not suffer from
|
||||
// the override mistake, that accessor's getter is marked with
|
||||
// an `originalValue` property. Here, when we detect this, we
|
||||
// uphold the illusion by pretending to see that original data
|
||||
// property, i.e., returning the value rather than the getter
|
||||
// itself.
|
||||
if (isOwn && 'get' in desc && !('originalValue' in desc.get)) {
|
||||
value = desc.get;
|
||||
} else {
|
||||
value = value[part];
|
||||
}
|
||||
} else {
|
||||
isOwn = hasOwn(value, part);
|
||||
value = value[part];
|
||||
}
|
||||
|
||||
if (isOwn && !skipFurtherCaching) {
|
||||
INTRINSICS[intrinsicRealName] = value;
|
||||
}
|
||||
}
|
||||
}
|
||||
return value;
|
||||
};
|
|
@ -0,0 +1,91 @@
|
|||
{
|
||||
"name": "get-intrinsic",
|
||||
"version": "1.1.3",
|
||||
"description": "Get and robustly cache all JS language-level intrinsics at first require time",
|
||||
"main": "index.js",
|
||||
"exports": {
|
||||
".": [
|
||||
{
|
||||
"default": "./index.js"
|
||||
},
|
||||
"./index.js"
|
||||
],
|
||||
"./package.json": "./package.json"
|
||||
},
|
||||
"scripts": {
|
||||
"prepack": "npmignore --auto --commentLines=autogenerated",
|
||||
"prepublish": "not-in-publish || npm run prepublishOnly",
|
||||
"prepublishOnly": "safe-publish-latest",
|
||||
"prelint": "evalmd README.md",
|
||||
"lint": "eslint --ext=.js,.mjs .",
|
||||
"pretest": "npm run lint",
|
||||
"tests-only": "nyc tape 'test/**/*.js'",
|
||||
"test": "npm run tests-only",
|
||||
"posttest": "aud --production",
|
||||
"version": "auto-changelog && git add CHANGELOG.md",
|
||||
"postversion": "auto-changelog && git add CHANGELOG.md && git commit --no-edit --amend && git tag -f \"v$(node -e \"console.log(require('./package.json').version)\")\""
|
||||
},
|
||||
"repository": {
|
||||
"type": "git",
|
||||
"url": "git+https://github.com/ljharb/get-intrinsic.git"
|
||||
},
|
||||
"keywords": [
|
||||
"javascript",
|
||||
"ecmascript",
|
||||
"es",
|
||||
"js",
|
||||
"intrinsic",
|
||||
"getintrinsic",
|
||||
"es-abstract"
|
||||
],
|
||||
"author": "Jordan Harband <ljharb@gmail.com>",
|
||||
"funding": {
|
||||
"url": "https://github.com/sponsors/ljharb"
|
||||
},
|
||||
"license": "MIT",
|
||||
"bugs": {
|
||||
"url": "https://github.com/ljharb/get-intrinsic/issues"
|
||||
},
|
||||
"homepage": "https://github.com/ljharb/get-intrinsic#readme",
|
||||
"devDependencies": {
|
||||
"@ljharb/eslint-config": "^21.0.0",
|
||||
"aud": "^2.0.0",
|
||||
"auto-changelog": "^2.4.0",
|
||||
"call-bind": "^1.0.2",
|
||||
"es-abstract": "^1.20.2",
|
||||
"es-value-fixtures": "^1.4.2",
|
||||
"eslint": "=8.8.0",
|
||||
"evalmd": "^0.0.19",
|
||||
"for-each": "^0.3.3",
|
||||
"make-async-function": "^1.0.0",
|
||||
"make-async-generator-function": "^1.0.0",
|
||||
"make-generator-function": "^2.0.0",
|
||||
"mock-property": "^1.0.0",
|
||||
"npmignore": "^0.3.0",
|
||||
"nyc": "^10.3.2",
|
||||
"object-inspect": "^1.12.2",
|
||||
"safe-publish-latest": "^2.0.0",
|
||||
"tape": "^5.6.0"
|
||||
},
|
||||
"auto-changelog": {
|
||||
"output": "CHANGELOG.md",
|
||||
"template": "keepachangelog",
|
||||
"unreleased": false,
|
||||
"commitLimit": false,
|
||||
"backfillLimit": false,
|
||||
"hideCredit": true
|
||||
},
|
||||
"dependencies": {
|
||||
"function-bind": "^1.1.1",
|
||||
"has": "^1.0.3",
|
||||
"has-symbols": "^1.0.3"
|
||||
},
|
||||
"testling": {
|
||||
"files": "test/GetIntrinsic.js"
|
||||
},
|
||||
"publishConfig": {
|
||||
"ignore": [
|
||||
".github/workflows"
|
||||
]
|
||||
}
|
||||
}
|
|
@ -0,0 +1,274 @@
|
|||
'use strict';
|
||||
|
||||
var GetIntrinsic = require('../');
|
||||
|
||||
var test = require('tape');
|
||||
var forEach = require('for-each');
|
||||
var debug = require('object-inspect');
|
||||
var generatorFns = require('make-generator-function')();
|
||||
var asyncFns = require('make-async-function').list();
|
||||
var asyncGenFns = require('make-async-generator-function')();
|
||||
var mockProperty = require('mock-property');
|
||||
|
||||
var callBound = require('call-bind/callBound');
|
||||
var v = require('es-value-fixtures');
|
||||
var $gOPD = require('es-abstract/helpers/getOwnPropertyDescriptor');
|
||||
var DefinePropertyOrThrow = require('es-abstract/2021/DefinePropertyOrThrow');
|
||||
|
||||
var $isProto = callBound('%Object.prototype.isPrototypeOf%');
|
||||
|
||||
test('export', function (t) {
|
||||
t.equal(typeof GetIntrinsic, 'function', 'it is a function');
|
||||
t.equal(GetIntrinsic.length, 2, 'function has length of 2');
|
||||
|
||||
t.end();
|
||||
});
|
||||
|
||||
test('throws', function (t) {
|
||||
t['throws'](
|
||||
function () { GetIntrinsic('not an intrinsic'); },
|
||||
SyntaxError,
|
||||
'nonexistent intrinsic throws a syntax error'
|
||||
);
|
||||
|
||||
t['throws'](
|
||||
function () { GetIntrinsic(''); },
|
||||
TypeError,
|
||||
'empty string intrinsic throws a type error'
|
||||
);
|
||||
|
||||
t['throws'](
|
||||
function () { GetIntrinsic('.'); },
|
||||
SyntaxError,
|
||||
'"just a dot" intrinsic throws a syntax error'
|
||||
);
|
||||
|
||||
t['throws'](
|
||||
function () { GetIntrinsic('%String'); },
|
||||
SyntaxError,
|
||||
'Leading % without trailing % throws a syntax error'
|
||||
);
|
||||
|
||||
t['throws'](
|
||||
function () { GetIntrinsic('String%'); },
|
||||
SyntaxError,
|
||||
'Trailing % without leading % throws a syntax error'
|
||||
);
|
||||
|
||||
t['throws'](
|
||||
function () { GetIntrinsic("String['prototype]"); },
|
||||
SyntaxError,
|
||||
'Dynamic property access is disallowed for intrinsics (unterminated string)'
|
||||
);
|
||||
|
||||
t['throws'](
|
||||
function () { GetIntrinsic('%Proxy.prototype.undefined%'); },
|
||||
TypeError,
|
||||
"Throws when middle part doesn't exist (%Proxy.prototype.undefined%)"
|
||||
);
|
||||
|
||||
t['throws'](
|
||||
function () { GetIntrinsic('%Array.prototype%garbage%'); },
|
||||
SyntaxError,
|
||||
'Throws with extra percent signs'
|
||||
);
|
||||
|
||||
t['throws'](
|
||||
function () { GetIntrinsic('%Array.prototype%push%'); },
|
||||
SyntaxError,
|
||||
'Throws with extra percent signs, even on an existing intrinsic'
|
||||
);
|
||||
|
||||
forEach(v.nonStrings, function (nonString) {
|
||||
t['throws'](
|
||||
function () { GetIntrinsic(nonString); },
|
||||
TypeError,
|
||||
debug(nonString) + ' is not a String'
|
||||
);
|
||||
});
|
||||
|
||||
forEach(v.nonBooleans, function (nonBoolean) {
|
||||
t['throws'](
|
||||
function () { GetIntrinsic('%', nonBoolean); },
|
||||
TypeError,
|
||||
debug(nonBoolean) + ' is not a Boolean'
|
||||
);
|
||||
});
|
||||
|
||||
forEach([
|
||||
'toString',
|
||||
'propertyIsEnumerable',
|
||||
'hasOwnProperty'
|
||||
], function (objectProtoMember) {
|
||||
t['throws'](
|
||||
function () { GetIntrinsic(objectProtoMember); },
|
||||
SyntaxError,
|
||||
debug(objectProtoMember) + ' is not an intrinsic'
|
||||
);
|
||||
});
|
||||
|
||||
t.end();
|
||||
});
|
||||
|
||||
test('base intrinsics', function (t) {
|
||||
t.equal(GetIntrinsic('%Object%'), Object, '%Object% yields Object');
|
||||
t.equal(GetIntrinsic('Object'), Object, 'Object yields Object');
|
||||
t.equal(GetIntrinsic('%Array%'), Array, '%Array% yields Array');
|
||||
t.equal(GetIntrinsic('Array'), Array, 'Array yields Array');
|
||||
|
||||
t.end();
|
||||
});
|
||||
|
||||
test('dotted paths', function (t) {
|
||||
t.equal(GetIntrinsic('%Object.prototype.toString%'), Object.prototype.toString, '%Object.prototype.toString% yields Object.prototype.toString');
|
||||
t.equal(GetIntrinsic('Object.prototype.toString'), Object.prototype.toString, 'Object.prototype.toString yields Object.prototype.toString');
|
||||
t.equal(GetIntrinsic('%Array.prototype.push%'), Array.prototype.push, '%Array.prototype.push% yields Array.prototype.push');
|
||||
t.equal(GetIntrinsic('Array.prototype.push'), Array.prototype.push, 'Array.prototype.push yields Array.prototype.push');
|
||||
|
||||
test('underscore paths are aliases for dotted paths', { skip: !Object.isFrozen || Object.isFrozen(Object.prototype) }, function (st) {
|
||||
var original = GetIntrinsic('%ObjProto_toString%');
|
||||
|
||||
forEach([
|
||||
'%Object.prototype.toString%',
|
||||
'Object.prototype.toString',
|
||||
'%ObjectPrototype.toString%',
|
||||
'ObjectPrototype.toString',
|
||||
'%ObjProto_toString%',
|
||||
'ObjProto_toString'
|
||||
], function (name) {
|
||||
DefinePropertyOrThrow(Object.prototype, 'toString', {
|
||||
'[[Value]]': function toString() {
|
||||
return original.apply(this, arguments);
|
||||
}
|
||||
});
|
||||
st.equal(GetIntrinsic(name), original, name + ' yields original Object.prototype.toString');
|
||||
});
|
||||
|
||||
DefinePropertyOrThrow(Object.prototype, 'toString', { '[[Value]]': original });
|
||||
st.end();
|
||||
});
|
||||
|
||||
test('dotted paths cache', { skip: !Object.isFrozen || Object.isFrozen(Object.prototype) }, function (st) {
|
||||
var original = GetIntrinsic('%Object.prototype.propertyIsEnumerable%');
|
||||
|
||||
forEach([
|
||||
'%Object.prototype.propertyIsEnumerable%',
|
||||
'Object.prototype.propertyIsEnumerable',
|
||||
'%ObjectPrototype.propertyIsEnumerable%',
|
||||
'ObjectPrototype.propertyIsEnumerable'
|
||||
], function (name) {
|
||||
var restore = mockProperty(Object.prototype, 'propertyIsEnumerable', {
|
||||
value: function propertyIsEnumerable() {
|
||||
return original.apply(this, arguments);
|
||||
}
|
||||
});
|
||||
st.equal(GetIntrinsic(name), original, name + ' yields cached Object.prototype.propertyIsEnumerable');
|
||||
|
||||
restore();
|
||||
});
|
||||
|
||||
st.end();
|
||||
});
|
||||
|
||||
test('dotted path reports correct error', function (st) {
|
||||
st['throws'](function () {
|
||||
GetIntrinsic('%NonExistentIntrinsic.prototype.property%');
|
||||
}, /%NonExistentIntrinsic%/, 'The base intrinsic of %NonExistentIntrinsic.prototype.property% is %NonExistentIntrinsic%');
|
||||
|
||||
st['throws'](function () {
|
||||
GetIntrinsic('%NonExistentIntrinsicPrototype.property%');
|
||||
}, /%NonExistentIntrinsicPrototype%/, 'The base intrinsic of %NonExistentIntrinsicPrototype.property% is %NonExistentIntrinsicPrototype%');
|
||||
|
||||
st.end();
|
||||
});
|
||||
|
||||
t.end();
|
||||
});
|
||||
|
||||
test('accessors', { skip: !$gOPD || typeof Map !== 'function' }, function (t) {
|
||||
var actual = $gOPD(Map.prototype, 'size');
|
||||
t.ok(actual, 'Map.prototype.size has a descriptor');
|
||||
t.equal(typeof actual.get, 'function', 'Map.prototype.size has a getter function');
|
||||
t.equal(GetIntrinsic('%Map.prototype.size%'), actual.get, '%Map.prototype.size% yields the getter for it');
|
||||
t.equal(GetIntrinsic('Map.prototype.size'), actual.get, 'Map.prototype.size yields the getter for it');
|
||||
|
||||
t.end();
|
||||
});
|
||||
|
||||
test('generator functions', { skip: !generatorFns.length }, function (t) {
|
||||
var $GeneratorFunction = GetIntrinsic('%GeneratorFunction%');
|
||||
var $GeneratorFunctionPrototype = GetIntrinsic('%Generator%');
|
||||
var $GeneratorPrototype = GetIntrinsic('%GeneratorPrototype%');
|
||||
|
||||
forEach(generatorFns, function (genFn) {
|
||||
var fnName = genFn.name;
|
||||
fnName = fnName ? "'" + fnName + "'" : 'genFn';
|
||||
|
||||
t.ok(genFn instanceof $GeneratorFunction, fnName + ' instanceof %GeneratorFunction%');
|
||||
t.ok($isProto($GeneratorFunctionPrototype, genFn), '%Generator% is prototype of ' + fnName);
|
||||
t.ok($isProto($GeneratorPrototype, genFn.prototype), '%GeneratorPrototype% is prototype of ' + fnName + '.prototype');
|
||||
});
|
||||
|
||||
t.end();
|
||||
});
|
||||
|
||||
test('async functions', { skip: !asyncFns.length }, function (t) {
|
||||
var $AsyncFunction = GetIntrinsic('%AsyncFunction%');
|
||||
var $AsyncFunctionPrototype = GetIntrinsic('%AsyncFunctionPrototype%');
|
||||
|
||||
forEach(asyncFns, function (asyncFn) {
|
||||
var fnName = asyncFn.name;
|
||||
fnName = fnName ? "'" + fnName + "'" : 'asyncFn';
|
||||
|
||||
t.ok(asyncFn instanceof $AsyncFunction, fnName + ' instanceof %AsyncFunction%');
|
||||
t.ok($isProto($AsyncFunctionPrototype, asyncFn), '%AsyncFunctionPrototype% is prototype of ' + fnName);
|
||||
});
|
||||
|
||||
t.end();
|
||||
});
|
||||
|
||||
test('async generator functions', { skip: asyncGenFns.length === 0 }, function (t) {
|
||||
var $AsyncGeneratorFunction = GetIntrinsic('%AsyncGeneratorFunction%');
|
||||
var $AsyncGeneratorFunctionPrototype = GetIntrinsic('%AsyncGenerator%');
|
||||
var $AsyncGeneratorPrototype = GetIntrinsic('%AsyncGeneratorPrototype%');
|
||||
|
||||
forEach(asyncGenFns, function (asyncGenFn) {
|
||||
var fnName = asyncGenFn.name;
|
||||
fnName = fnName ? "'" + fnName + "'" : 'asyncGenFn';
|
||||
|
||||
t.ok(asyncGenFn instanceof $AsyncGeneratorFunction, fnName + ' instanceof %AsyncGeneratorFunction%');
|
||||
t.ok($isProto($AsyncGeneratorFunctionPrototype, asyncGenFn), '%AsyncGenerator% is prototype of ' + fnName);
|
||||
t.ok($isProto($AsyncGeneratorPrototype, asyncGenFn.prototype), '%AsyncGeneratorPrototype% is prototype of ' + fnName + '.prototype');
|
||||
});
|
||||
|
||||
t.end();
|
||||
});
|
||||
|
||||
test('%ThrowTypeError%', function (t) {
|
||||
var $ThrowTypeError = GetIntrinsic('%ThrowTypeError%');
|
||||
|
||||
t.equal(typeof $ThrowTypeError, 'function', 'is a function');
|
||||
t['throws'](
|
||||
$ThrowTypeError,
|
||||
TypeError,
|
||||
'%ThrowTypeError% throws a TypeError'
|
||||
);
|
||||
|
||||
t.end();
|
||||
});
|
||||
|
||||
test('allowMissing', { skip: asyncGenFns.length > 0 }, function (t) {
|
||||
t['throws'](
|
||||
function () { GetIntrinsic('%AsyncGeneratorPrototype%'); },
|
||||
TypeError,
|
||||
'throws when missing'
|
||||
);
|
||||
|
||||
t.equal(
|
||||
GetIntrinsic('%AsyncGeneratorPrototype%', true),
|
||||
undefined,
|
||||
'does not throw when allowMissing'
|
||||
);
|
||||
|
||||
t.end();
|
||||
});
|
|
@ -0,0 +1,11 @@
|
|||
{
|
||||
"root": true,
|
||||
|
||||
"extends": "@ljharb",
|
||||
|
||||
"rules": {
|
||||
"max-statements-per-line": [2, { "max": 2 }],
|
||||
"no-magic-numbers": 0,
|
||||
"multiline-comment-style": 0,
|
||||
}
|
||||
}
|
|
@ -0,0 +1,12 @@
|
|||
# These are supported funding model platforms
|
||||
|
||||
github: [ljharb]
|
||||
patreon: # Replace with a single Patreon username
|
||||
open_collective: # Replace with a single Open Collective username
|
||||
ko_fi: # Replace with a single Ko-fi username
|
||||
tidelift: npm/has-symbols
|
||||
community_bridge: # Replace with a single Community Bridge project-name e.g., cloud-foundry
|
||||
liberapay: # Replace with a single Liberapay username
|
||||
issuehunt: # Replace with a single IssueHunt username
|
||||
otechie: # Replace with a single Otechie username
|
||||
custom: # Replace with up to 4 custom sponsorship URLs e.g., ['link1', 'link2']
|
|
@ -0,0 +1,9 @@
|
|||
{
|
||||
"all": true,
|
||||
"check-coverage": false,
|
||||
"reporter": ["text-summary", "text", "html", "json"],
|
||||
"exclude": [
|
||||
"coverage",
|
||||
"test"
|
||||
]
|
||||
}
|
|
@ -0,0 +1,75 @@
|
|||
# Changelog
|
||||
|
||||
All notable changes to this project will be documented in this file.
|
||||
|
||||
The format is based on [Keep a Changelog](https://keepachangelog.com/en/1.0.0/)
|
||||
and this project adheres to [Semantic Versioning](https://semver.org/spec/v2.0.0.html).
|
||||
|
||||
## [v1.0.3](https://github.com/inspect-js/has-symbols/compare/v1.0.2...v1.0.3) - 2022-03-01
|
||||
|
||||
### Commits
|
||||
|
||||
- [actions] use `node/install` instead of `node/run`; use `codecov` action [`518b28f`](https://github.com/inspect-js/has-symbols/commit/518b28f6c5a516cbccae30794e40aa9f738b1693)
|
||||
- [meta] add `bugs` and `homepage` fields; reorder package.json [`c480b13`](https://github.com/inspect-js/has-symbols/commit/c480b13fd6802b557e1cef9749872cb5fdeef744)
|
||||
- [actions] reuse common workflows [`01d0ee0`](https://github.com/inspect-js/has-symbols/commit/01d0ee0a8d97c0947f5edb73eb722027a77b2b07)
|
||||
- [actions] update codecov uploader [`6424ebe`](https://github.com/inspect-js/has-symbols/commit/6424ebe86b2c9c7c3d2e9bd4413a4e4f168cb275)
|
||||
- [Dev Deps] update `eslint`, `@ljharb/eslint-config`, `aud`, `auto-changelog`, `tape` [`dfa7e7f`](https://github.com/inspect-js/has-symbols/commit/dfa7e7ff38b594645d8c8222aab895157fa7e282)
|
||||
- [Dev Deps] update `eslint`, `@ljharb/eslint-config`, `safe-publish-latest`, `tape` [`0c8d436`](https://github.com/inspect-js/has-symbols/commit/0c8d43685c45189cea9018191d4fd7eca91c9d02)
|
||||
- [Dev Deps] update `eslint`, `@ljharb/eslint-config`, `aud`, `tape` [`9026554`](https://github.com/inspect-js/has-symbols/commit/902655442a1bf88e72b42345494ef0c60f5d36ab)
|
||||
- [readme] add actions and codecov badges [`eaa9682`](https://github.com/inspect-js/has-symbols/commit/eaa9682f990f481d3acf7a1c7600bec36f7b3adc)
|
||||
- [Dev Deps] update `eslint`, `tape` [`bc7a3ba`](https://github.com/inspect-js/has-symbols/commit/bc7a3ba46f27b7743f8a2579732d59d1b9ac791e)
|
||||
- [Dev Deps] update `eslint`, `auto-changelog` [`0ace00a`](https://github.com/inspect-js/has-symbols/commit/0ace00af08a88cdd1e6ce0d60357d941c60c2d9f)
|
||||
- [meta] use `prepublishOnly` script for npm 7+ [`093f72b`](https://github.com/inspect-js/has-symbols/commit/093f72bc2b0ed00c781f444922a5034257bf561d)
|
||||
- [Tests] test on all 16 minors [`9b80d3d`](https://github.com/inspect-js/has-symbols/commit/9b80d3d9102529f04c20ec5b1fcc6e38426c6b03)
|
||||
|
||||
## [v1.0.2](https://github.com/inspect-js/has-symbols/compare/v1.0.1...v1.0.2) - 2021-02-27
|
||||
|
||||
### Fixed
|
||||
|
||||
- [Fix] use a universal way to get the original Symbol [`#11`](https://github.com/inspect-js/has-symbols/issues/11)
|
||||
|
||||
### Commits
|
||||
|
||||
- [Tests] migrate tests to Github Actions [`90ae798`](https://github.com/inspect-js/has-symbols/commit/90ae79820bdfe7bc703d67f5f3c5e205f98556d3)
|
||||
- [meta] do not publish github action workflow files [`29e60a1`](https://github.com/inspect-js/has-symbols/commit/29e60a1b7c25c7f1acf7acff4a9320d0d10c49b4)
|
||||
- [Tests] run `nyc` on all tests [`8476b91`](https://github.com/inspect-js/has-symbols/commit/8476b915650d360915abe2522505abf4b0e8f0ae)
|
||||
- [readme] fix repo URLs, remove defunct badges [`126288e`](https://github.com/inspect-js/has-symbols/commit/126288ecc1797c0a40247a6b78bcb2e0bc5d7036)
|
||||
- [Dev Deps] update `eslint`, `@ljharb/eslint-config`, `aud`, `auto-changelog`, `core-js`, `get-own-property-symbols` [`d84bdfa`](https://github.com/inspect-js/has-symbols/commit/d84bdfa48ac5188abbb4904b42614cd6c030940a)
|
||||
- [Tests] fix linting errors [`0df3070`](https://github.com/inspect-js/has-symbols/commit/0df3070b981b6c9f2ee530c09189a7f5c6def839)
|
||||
- [actions] add "Allow Edits" workflow [`1e6bc29`](https://github.com/inspect-js/has-symbols/commit/1e6bc29b188f32b9648657b07eda08504be5aa9c)
|
||||
- [Dev Deps] update `eslint`, `@ljharb/eslint-config`, `tape` [`36cea2a`](https://github.com/inspect-js/has-symbols/commit/36cea2addd4e6ec435f35a2656b4e9ef82498e9b)
|
||||
- [Dev Deps] update `eslint`, `@ljharb/eslint-config`, `aud`, `tape` [`1278338`](https://github.com/inspect-js/has-symbols/commit/127833801865fbc2cc8979beb9ca869c7bfe8222)
|
||||
- [Dev Deps] update `eslint`, `@ljharb/eslint-config`, `aud`, `tape` [`1493254`](https://github.com/inspect-js/has-symbols/commit/1493254eda13db5fb8fc5e4a3e8324b3d196029d)
|
||||
- [Dev Deps] update `eslint`, `@ljharb/eslint-config`, `core-js` [`b090bf2`](https://github.com/inspect-js/has-symbols/commit/b090bf214d3679a30edc1e2d729d466ab5183e1d)
|
||||
- [actions] switch Automatic Rebase workflow to `pull_request_target` event [`4addb7a`](https://github.com/inspect-js/has-symbols/commit/4addb7ab4dc73f927ae99928d68817554fc21dc0)
|
||||
- [Dev Deps] update `auto-changelog`, `tape` [`81d0baf`](https://github.com/inspect-js/has-symbols/commit/81d0baf3816096a89a8558e8043895f7a7d10d8b)
|
||||
- [Dev Deps] update `auto-changelog`; add `aud` [`1a4e561`](https://github.com/inspect-js/has-symbols/commit/1a4e5612c25d91c3a03d509721d02630bc4fe3da)
|
||||
- [readme] remove unused testling URLs [`3000941`](https://github.com/inspect-js/has-symbols/commit/3000941f958046e923ed8152edb1ef4a599e6fcc)
|
||||
- [Tests] only audit prod deps [`692e974`](https://github.com/inspect-js/has-symbols/commit/692e9743c912410e9440207631a643a34b4741a1)
|
||||
- [Dev Deps] update `@ljharb/eslint-config` [`51c946c`](https://github.com/inspect-js/has-symbols/commit/51c946c7f6baa793ec5390bb5a45cdce16b4ba76)
|
||||
|
||||
## [v1.0.1](https://github.com/inspect-js/has-symbols/compare/v1.0.0...v1.0.1) - 2019-11-16
|
||||
|
||||
### Commits
|
||||
|
||||
- [Tests] use shared travis-ci configs [`ce396c9`](https://github.com/inspect-js/has-symbols/commit/ce396c9419ff11c43d0da5d05cdbb79f7fb42229)
|
||||
- [Tests] up to `node` `v12.4`, `v11.15`, `v10.15`, `v9.11`, `v8.15`, `v7.10`, `v6.17`, `v4.9`; use `nvm install-latest-npm` [`0690732`](https://github.com/inspect-js/has-symbols/commit/0690732801f47ab429f39ba1962f522d5c462d6b)
|
||||
- [meta] add `auto-changelog` [`2163d0b`](https://github.com/inspect-js/has-symbols/commit/2163d0b7f36343076b8f947cd1667dd1750f26fc)
|
||||
- [Dev Deps] update `eslint`, `@ljharb/eslint-config`, `core-js`, `safe-publish-latest`, `tape` [`8e0951f`](https://github.com/inspect-js/has-symbols/commit/8e0951f1a7a2e52068222b7bb73511761e6e4d9c)
|
||||
- [actions] add automatic rebasing / merge commit blocking [`b09cdb7`](https://github.com/inspect-js/has-symbols/commit/b09cdb7cd7ee39e7a769878f56e2d6066f5ccd1d)
|
||||
- [Dev Deps] update `eslint`, `@ljharb/eslint-config`, `safe-publish-latest`, `core-js`, `get-own-property-symbols`, `tape` [`1dd42cd`](https://github.com/inspect-js/has-symbols/commit/1dd42cd86183ed0c50f99b1062345c458babca91)
|
||||
- [meta] create FUNDING.yml [`aa57a17`](https://github.com/inspect-js/has-symbols/commit/aa57a17b19708906d1927f821ea8e73394d84ca4)
|
||||
- Only apps should have lockfiles [`a2d8bea`](https://github.com/inspect-js/has-symbols/commit/a2d8bea23a97d15c09eaf60f5b107fcf9a4d57aa)
|
||||
- [Tests] use `npx aud` instead of `nsp` or `npm audit` with hoops [`9e96cb7`](https://github.com/inspect-js/has-symbols/commit/9e96cb783746cbed0c10ef78e599a8eaa7ebe193)
|
||||
- [meta] add `funding` field [`a0b32cf`](https://github.com/inspect-js/has-symbols/commit/a0b32cf68e803f963c1639b6d47b0a9d6440bab0)
|
||||
- [Dev Deps] update `safe-publish-latest` [`cb9f0a5`](https://github.com/inspect-js/has-symbols/commit/cb9f0a521a3a1790f1064d437edd33bb6c3d6af0)
|
||||
|
||||
## v1.0.0 - 2016-09-19
|
||||
|
||||
### Commits
|
||||
|
||||
- Tests. [`ecb6eb9`](https://github.com/inspect-js/has-symbols/commit/ecb6eb934e4883137f3f93b965ba5e0a98df430d)
|
||||
- package.json [`88a337c`](https://github.com/inspect-js/has-symbols/commit/88a337cee0864a0da35f5d19e69ff0ef0150e46a)
|
||||
- Initial commit [`42e1e55`](https://github.com/inspect-js/has-symbols/commit/42e1e5502536a2b8ac529c9443984acd14836b1c)
|
||||
- Initial implementation. [`33f5cc6`](https://github.com/inspect-js/has-symbols/commit/33f5cc6cdff86e2194b081ee842bfdc63caf43fb)
|
||||
- read me [`01f1170`](https://github.com/inspect-js/has-symbols/commit/01f1170188ff7cb1558aa297f6ba5b516c6d7b0c)
|
|
@ -0,0 +1,21 @@
|
|||
MIT License
|
||||
|
||||
Copyright (c) 2016 Jordan Harband
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
of this software and associated documentation files (the "Software"), to deal
|
||||
in the Software without restriction, including without limitation the rights
|
||||
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
copies of the Software, and to permit persons to whom the Software is
|
||||
furnished to do so, subject to the following conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be included in all
|
||||
copies or substantial portions of the Software.
|
||||
|
||||
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
SOFTWARE.
|
|
@ -0,0 +1,46 @@
|
|||
# has-symbols <sup>[![Version Badge][2]][1]</sup>
|
||||
|
||||
[![github actions][actions-image]][actions-url]
|
||||
[![coverage][codecov-image]][codecov-url]
|
||||
[![dependency status][5]][6]
|
||||
[![dev dependency status][7]][8]
|
||||
[![License][license-image]][license-url]
|
||||
[![Downloads][downloads-image]][downloads-url]
|
||||
|
||||
[![npm badge][11]][1]
|
||||
|
||||
Determine if the JS environment has Symbol support. Supports spec, or shams.
|
||||
|
||||
## Example
|
||||
|
||||
```js
|
||||
var hasSymbols = require('has-symbols');
|
||||
|
||||
hasSymbols() === true; // if the environment has native Symbol support. Not polyfillable, not forgeable.
|
||||
|
||||
var hasSymbolsKinda = require('has-symbols/shams');
|
||||
hasSymbolsKinda() === true; // if the environment has a Symbol sham that mostly follows the spec.
|
||||
```
|
||||
|
||||
## Supported Symbol shams
|
||||
- get-own-property-symbols [npm](https://www.npmjs.com/package/get-own-property-symbols) | [github](https://github.com/WebReflection/get-own-property-symbols)
|
||||
- core-js [npm](https://www.npmjs.com/package/core-js) | [github](https://github.com/zloirock/core-js)
|
||||
|
||||
## Tests
|
||||
Simply clone the repo, `npm install`, and run `npm test`
|
||||
|
||||
[1]: https://npmjs.org/package/has-symbols
|
||||
[2]: https://versionbadg.es/inspect-js/has-symbols.svg
|
||||
[5]: https://david-dm.org/inspect-js/has-symbols.svg
|
||||
[6]: https://david-dm.org/inspect-js/has-symbols
|
||||
[7]: https://david-dm.org/inspect-js/has-symbols/dev-status.svg
|
||||
[8]: https://david-dm.org/inspect-js/has-symbols#info=devDependencies
|
||||
[11]: https://nodei.co/npm/has-symbols.png?downloads=true&stars=true
|
||||
[license-image]: https://img.shields.io/npm/l/has-symbols.svg
|
||||
[license-url]: LICENSE
|
||||
[downloads-image]: https://img.shields.io/npm/dm/has-symbols.svg
|
||||
[downloads-url]: https://npm-stat.com/charts.html?package=has-symbols
|
||||
[codecov-image]: https://codecov.io/gh/inspect-js/has-symbols/branch/main/graphs/badge.svg
|
||||
[codecov-url]: https://app.codecov.io/gh/inspect-js/has-symbols/
|
||||
[actions-image]: https://img.shields.io/endpoint?url=https://github-actions-badge-u3jn4tfpocch.runkit.sh/inspect-js/has-symbols
|
||||
[actions-url]: https://github.com/inspect-js/has-symbols/actions
|
|
@ -0,0 +1,13 @@
|
|||
'use strict';
|
||||
|
||||
var origSymbol = typeof Symbol !== 'undefined' && Symbol;
|
||||
var hasSymbolSham = require('./shams');
|
||||
|
||||
module.exports = function hasNativeSymbols() {
|
||||
if (typeof origSymbol !== 'function') { return false; }
|
||||
if (typeof Symbol !== 'function') { return false; }
|
||||
if (typeof origSymbol('foo') !== 'symbol') { return false; }
|
||||
if (typeof Symbol('bar') !== 'symbol') { return false; }
|
||||
|
||||
return hasSymbolSham();
|
||||
};
|
|
@ -0,0 +1,101 @@
|
|||
{
|
||||
"name": "has-symbols",
|
||||
"version": "1.0.3",
|
||||
"description": "Determine if the JS environment has Symbol support. Supports spec, or shams.",
|
||||
"main": "index.js",
|
||||
"scripts": {
|
||||
"prepublishOnly": "safe-publish-latest",
|
||||
"prepublish": "not-in-publish || npm run prepublishOnly",
|
||||
"pretest": "npm run --silent lint",
|
||||
"test": "npm run tests-only",
|
||||
"posttest": "aud --production",
|
||||
"tests-only": "npm run test:stock && npm run test:staging && npm run test:shams",
|
||||
"test:stock": "nyc node test",
|
||||
"test:staging": "nyc node --harmony --es-staging test",
|
||||
"test:shams": "npm run --silent test:shams:getownpropertysymbols && npm run --silent test:shams:corejs",
|
||||
"test:shams:corejs": "nyc node test/shams/core-js.js",
|
||||
"test:shams:getownpropertysymbols": "nyc node test/shams/get-own-property-symbols.js",
|
||||
"lint": "eslint --ext=js,mjs .",
|
||||
"version": "auto-changelog && git add CHANGELOG.md",
|
||||
"postversion": "auto-changelog && git add CHANGELOG.md && git commit --no-edit --amend && git tag -f \"v$(node -e \"console.log(require('./package.json').version)\")\""
|
||||
},
|
||||
"repository": {
|
||||
"type": "git",
|
||||
"url": "git://github.com/inspect-js/has-symbols.git"
|
||||
},
|
||||
"keywords": [
|
||||
"Symbol",
|
||||
"symbols",
|
||||
"typeof",
|
||||
"sham",
|
||||
"polyfill",
|
||||
"native",
|
||||
"core-js",
|
||||
"ES6"
|
||||
],
|
||||
"author": {
|
||||
"name": "Jordan Harband",
|
||||
"email": "ljharb@gmail.com",
|
||||
"url": "http://ljharb.codes"
|
||||
},
|
||||
"contributors": [
|
||||
{
|
||||
"name": "Jordan Harband",
|
||||
"email": "ljharb@gmail.com",
|
||||
"url": "http://ljharb.codes"
|
||||
}
|
||||
],
|
||||
"funding": {
|
||||
"url": "https://github.com/sponsors/ljharb"
|
||||
},
|
||||
"license": "MIT",
|
||||
"bugs": {
|
||||
"url": "https://github.com/ljharb/has-symbols/issues"
|
||||
},
|
||||
"homepage": "https://github.com/ljharb/has-symbols#readme",
|
||||
"devDependencies": {
|
||||
"@ljharb/eslint-config": "^20.2.3",
|
||||
"aud": "^2.0.0",
|
||||
"auto-changelog": "^2.4.0",
|
||||
"core-js": "^2.6.12",
|
||||
"eslint": "=8.8.0",
|
||||
"get-own-property-symbols": "^0.9.5",
|
||||
"nyc": "^10.3.2",
|
||||
"safe-publish-latest": "^2.0.0",
|
||||
"tape": "^5.5.2"
|
||||
},
|
||||
"testling": {
|
||||
"files": "test/index.js",
|
||||
"browsers": [
|
||||
"iexplore/6.0..latest",
|
||||
"firefox/3.0..6.0",
|
||||
"firefox/15.0..latest",
|
||||
"firefox/nightly",
|
||||
"chrome/4.0..10.0",
|
||||
"chrome/20.0..latest",
|
||||
"chrome/canary",
|
||||
"opera/10.0..latest",
|
||||
"opera/next",
|
||||
"safari/4.0..latest",
|
||||
"ipad/6.0..latest",
|
||||
"iphone/6.0..latest",
|
||||
"android-browser/4.2"
|
||||
]
|
||||
},
|
||||
"engines": {
|
||||
"node": ">= 0.4"
|
||||
},
|
||||
"auto-changelog": {
|
||||
"output": "CHANGELOG.md",
|
||||
"template": "keepachangelog",
|
||||
"unreleased": false,
|
||||
"commitLimit": false,
|
||||
"backfillLimit": false,
|
||||
"hideCredit": true
|
||||
},
|
||||
"greenkeeper": {
|
||||
"ignore": [
|
||||
"core-js"
|
||||
]
|
||||
}
|
||||
}
|
|
@ -0,0 +1,42 @@
|
|||
'use strict';
|
||||
|
||||
/* eslint complexity: [2, 18], max-statements: [2, 33] */
|
||||
module.exports = function hasSymbols() {
|
||||
if (typeof Symbol !== 'function' || typeof Object.getOwnPropertySymbols !== 'function') { return false; }
|
||||
if (typeof Symbol.iterator === 'symbol') { return true; }
|
||||
|
||||
var obj = {};
|
||||
var sym = Symbol('test');
|
||||
var symObj = Object(sym);
|
||||
if (typeof sym === 'string') { return false; }
|
||||
|
||||
if (Object.prototype.toString.call(sym) !== '[object Symbol]') { return false; }
|
||||
if (Object.prototype.toString.call(symObj) !== '[object Symbol]') { return false; }
|
||||
|
||||
// temp disabled per https://github.com/ljharb/object.assign/issues/17
|
||||
// if (sym instanceof Symbol) { return false; }
|
||||
// temp disabled per https://github.com/WebReflection/get-own-property-symbols/issues/4
|
||||
// if (!(symObj instanceof Symbol)) { return false; }
|
||||
|
||||
// if (typeof Symbol.prototype.toString !== 'function') { return false; }
|
||||
// if (String(sym) !== Symbol.prototype.toString.call(sym)) { return false; }
|
||||
|
||||
var symVal = 42;
|
||||
obj[sym] = symVal;
|
||||
for (sym in obj) { return false; } // eslint-disable-line no-restricted-syntax, no-unreachable-loop
|
||||
if (typeof Object.keys === 'function' && Object.keys(obj).length !== 0) { return false; }
|
||||
|
||||
if (typeof Object.getOwnPropertyNames === 'function' && Object.getOwnPropertyNames(obj).length !== 0) { return false; }
|
||||
|
||||
var syms = Object.getOwnPropertySymbols(obj);
|
||||
if (syms.length !== 1 || syms[0] !== sym) { return false; }
|
||||
|
||||
if (!Object.prototype.propertyIsEnumerable.call(obj, sym)) { return false; }
|
||||
|
||||
if (typeof Object.getOwnPropertyDescriptor === 'function') {
|
||||
var descriptor = Object.getOwnPropertyDescriptor(obj, sym);
|
||||
if (descriptor.value !== symVal || descriptor.enumerable !== true) { return false; }
|
||||
}
|
||||
|
||||
return true;
|
||||
};
|
|
@ -0,0 +1,22 @@
|
|||
'use strict';
|
||||
|
||||
var test = require('tape');
|
||||
var hasSymbols = require('../');
|
||||
var runSymbolTests = require('./tests');
|
||||
|
||||
test('interface', function (t) {
|
||||
t.equal(typeof hasSymbols, 'function', 'is a function');
|
||||
t.equal(typeof hasSymbols(), 'boolean', 'returns a boolean');
|
||||
t.end();
|
||||
});
|
||||
|
||||
test('Symbols are supported', { skip: !hasSymbols() }, function (t) {
|
||||
runSymbolTests(t);
|
||||
t.end();
|
||||
});
|
||||
|
||||
test('Symbols are not supported', { skip: hasSymbols() }, function (t) {
|
||||
t.equal(typeof Symbol, 'undefined', 'global Symbol is undefined');
|
||||
t.equal(typeof Object.getOwnPropertySymbols, 'undefined', 'Object.getOwnPropertySymbols does not exist');
|
||||
t.end();
|
||||
});
|
|
@ -0,0 +1,28 @@
|
|||
'use strict';
|
||||
|
||||
var test = require('tape');
|
||||
|
||||
if (typeof Symbol === 'function' && typeof Symbol() === 'symbol') {
|
||||
test('has native Symbol support', function (t) {
|
||||
t.equal(typeof Symbol, 'function');
|
||||
t.equal(typeof Symbol(), 'symbol');
|
||||
t.end();
|
||||
});
|
||||
return;
|
||||
}
|
||||
|
||||
var hasSymbols = require('../../shams');
|
||||
|
||||
test('polyfilled Symbols', function (t) {
|
||||
/* eslint-disable global-require */
|
||||
t.equal(hasSymbols(), false, 'hasSymbols is false before polyfilling');
|
||||
require('core-js/fn/symbol');
|
||||
require('core-js/fn/symbol/to-string-tag');
|
||||
|
||||
require('../tests')(t);
|
||||
|
||||
var hasSymbolsAfter = hasSymbols();
|
||||
t.equal(hasSymbolsAfter, true, 'hasSymbols is true after polyfilling');
|
||||
/* eslint-enable global-require */
|
||||
t.end();
|
||||
});
|
28
node_modules/has-symbols/test/shams/get-own-property-symbols.js
generated
vendored
Executable file
28
node_modules/has-symbols/test/shams/get-own-property-symbols.js
generated
vendored
Executable file
|
@ -0,0 +1,28 @@
|
|||
'use strict';
|
||||
|
||||
var test = require('tape');
|
||||
|
||||
if (typeof Symbol === 'function' && typeof Symbol() === 'symbol') {
|
||||
test('has native Symbol support', function (t) {
|
||||
t.equal(typeof Symbol, 'function');
|
||||
t.equal(typeof Symbol(), 'symbol');
|
||||
t.end();
|
||||
});
|
||||
return;
|
||||
}
|
||||
|
||||
var hasSymbols = require('../../shams');
|
||||
|
||||
test('polyfilled Symbols', function (t) {
|
||||
/* eslint-disable global-require */
|
||||
t.equal(hasSymbols(), false, 'hasSymbols is false before polyfilling');
|
||||
|
||||
require('get-own-property-symbols');
|
||||
|
||||
require('../tests')(t);
|
||||
|
||||
var hasSymbolsAfter = hasSymbols();
|
||||
t.equal(hasSymbolsAfter, true, 'hasSymbols is true after polyfilling');
|
||||
/* eslint-enable global-require */
|
||||
t.end();
|
||||
});
|
|
@ -0,0 +1,56 @@
|
|||
'use strict';
|
||||
|
||||
// eslint-disable-next-line consistent-return
|
||||
module.exports = function runSymbolTests(t) {
|
||||
t.equal(typeof Symbol, 'function', 'global Symbol is a function');
|
||||
|
||||
if (typeof Symbol !== 'function') { return false; }
|
||||
|
||||
t.notEqual(Symbol(), Symbol(), 'two symbols are not equal');
|
||||
|
||||
/*
|
||||
t.equal(
|
||||
Symbol.prototype.toString.call(Symbol('foo')),
|
||||
Symbol.prototype.toString.call(Symbol('foo')),
|
||||
'two symbols with the same description stringify the same'
|
||||
);
|
||||
*/
|
||||
|
||||
/*
|
||||
var foo = Symbol('foo');
|
||||
|
||||
t.notEqual(
|
||||
String(foo),
|
||||
String(Symbol('bar')),
|
||||
'two symbols with different descriptions do not stringify the same'
|
||||
);
|
||||
*/
|
||||
|
||||
t.equal(typeof Symbol.prototype.toString, 'function', 'Symbol#toString is a function');
|
||||
// t.equal(String(foo), Symbol.prototype.toString.call(foo), 'Symbol#toString equals String of the same symbol');
|
||||
|
||||
t.equal(typeof Object.getOwnPropertySymbols, 'function', 'Object.getOwnPropertySymbols is a function');
|
||||
|
||||
var obj = {};
|
||||
var sym = Symbol('test');
|
||||
var symObj = Object(sym);
|
||||
t.notEqual(typeof sym, 'string', 'Symbol is not a string');
|
||||
t.equal(Object.prototype.toString.call(sym), '[object Symbol]', 'symbol primitive Object#toStrings properly');
|
||||
t.equal(Object.prototype.toString.call(symObj), '[object Symbol]', 'symbol primitive Object#toStrings properly');
|
||||
|
||||
var symVal = 42;
|
||||
obj[sym] = symVal;
|
||||
// eslint-disable-next-line no-restricted-syntax
|
||||
for (sym in obj) { t.fail('symbol property key was found in for..in of object'); }
|
||||
|
||||
t.deepEqual(Object.keys(obj), [], 'no enumerable own keys on symbol-valued object');
|
||||
t.deepEqual(Object.getOwnPropertyNames(obj), [], 'no own names on symbol-valued object');
|
||||
t.deepEqual(Object.getOwnPropertySymbols(obj), [sym], 'one own symbol on symbol-valued object');
|
||||
t.equal(Object.prototype.propertyIsEnumerable.call(obj, sym), true, 'symbol is enumerable');
|
||||
t.deepEqual(Object.getOwnPropertyDescriptor(obj, sym), {
|
||||
configurable: true,
|
||||
enumerable: true,
|
||||
value: 42,
|
||||
writable: true
|
||||
}, 'property descriptor is correct');
|
||||
};
|
|
@ -0,0 +1,22 @@
|
|||
Copyright (c) 2013 Thiago de Arruda
|
||||
|
||||
Permission is hereby granted, free of charge, to any person
|
||||
obtaining a copy of this software and associated documentation
|
||||
files (the "Software"), to deal in the Software without
|
||||
restriction, including without limitation the rights to use,
|
||||
copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
copies of the Software, and to permit persons to whom the
|
||||
Software is furnished to do so, subject to the following
|
||||
conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be
|
||||
included in all copies or substantial portions of the Software.
|
||||
|
||||
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES
|
||||
OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT
|
||||
HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY,
|
||||
WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
|
||||
FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR
|
||||
OTHER DEALINGS IN THE SOFTWARE.
|
|
@ -0,0 +1,18 @@
|
|||
# has
|
||||
|
||||
> Object.prototype.hasOwnProperty.call shortcut
|
||||
|
||||
## Installation
|
||||
|
||||
```sh
|
||||
npm install --save has
|
||||
```
|
||||
|
||||
## Usage
|
||||
|
||||
```js
|
||||
var has = require('has');
|
||||
|
||||
has({}, 'hasOwnProperty'); // false
|
||||
has(Object.prototype, 'hasOwnProperty'); // true
|
||||
```
|
|
@ -0,0 +1,48 @@
|
|||
{
|
||||
"name": "has",
|
||||
"description": "Object.prototype.hasOwnProperty.call shortcut",
|
||||
"version": "1.0.3",
|
||||
"homepage": "https://github.com/tarruda/has",
|
||||
"author": {
|
||||
"name": "Thiago de Arruda",
|
||||
"email": "tpadilha84@gmail.com"
|
||||
},
|
||||
"contributors": [
|
||||
{
|
||||
"name": "Jordan Harband",
|
||||
"email": "ljharb@gmail.com",
|
||||
"url": "http://ljharb.codes"
|
||||
}
|
||||
],
|
||||
"repository": {
|
||||
"type": "git",
|
||||
"url": "git://github.com/tarruda/has.git"
|
||||
},
|
||||
"bugs": {
|
||||
"url": "https://github.com/tarruda/has/issues"
|
||||
},
|
||||
"license": "MIT",
|
||||
"licenses": [
|
||||
{
|
||||
"type": "MIT",
|
||||
"url": "https://github.com/tarruda/has/blob/master/LICENSE-MIT"
|
||||
}
|
||||
],
|
||||
"main": "./src",
|
||||
"dependencies": {
|
||||
"function-bind": "^1.1.1"
|
||||
},
|
||||
"devDependencies": {
|
||||
"@ljharb/eslint-config": "^12.2.1",
|
||||
"eslint": "^4.19.1",
|
||||
"tape": "^4.9.0"
|
||||
},
|
||||
"engines": {
|
||||
"node": ">= 0.4.0"
|
||||
},
|
||||
"scripts": {
|
||||
"lint": "eslint .",
|
||||
"pretest": "npm run lint",
|
||||
"test": "tape test"
|
||||
}
|
||||
}
|
|
@ -0,0 +1,5 @@
|
|||
'use strict';
|
||||
|
||||
var bind = require('function-bind');
|
||||
|
||||
module.exports = bind.call(Function.call, Object.prototype.hasOwnProperty);
|
|
@ -0,0 +1,10 @@
|
|||
'use strict';
|
||||
|
||||
var test = require('tape');
|
||||
var has = require('../');
|
||||
|
||||
test('has', function (t) {
|
||||
t.equal(has({}, 'hasOwnProperty'), false, 'object literal does not have own property "hasOwnProperty"');
|
||||
t.equal(has(Object.prototype, 'hasOwnProperty'), true, 'Object.prototype has own property "hasOwnProperty"');
|
||||
t.end();
|
||||
});
|
|
@ -1,3 +1,18 @@
|
|||
2.0.0 / 2021-12-17
|
||||
==================
|
||||
|
||||
* Drop support for Node.js 0.6
|
||||
* Remove `I'mateapot` export; use `ImATeapot` instead
|
||||
* Remove support for status being non-first argument
|
||||
* Rename `UnorderedCollection` constructor to `TooEarly`
|
||||
* deps: depd@2.0.0
|
||||
- Replace internal `eval` usage with `Function` constructor
|
||||
- Use instance methods on `process` to check for listeners
|
||||
* deps: statuses@2.0.1
|
||||
- Fix messaging casing of `418 I'm a Teapot`
|
||||
- Remove code 306
|
||||
- Rename `425 Unordered Collection` to standard `425 Too Early`
|
||||
|
||||
2021-11-14 / 1.8.1
|
||||
==================
|
||||
|
||||
|
|
|
@ -14,7 +14,7 @@ This is a [Node.js](https://nodejs.org/en/) module available through the
|
|||
[npm registry](https://www.npmjs.com/). Installation is done using the
|
||||
[`npm install` command](https://docs.npmjs.com/getting-started/installing-npm-packages-locally):
|
||||
|
||||
```bash
|
||||
```console
|
||||
$ npm install http-errors
|
||||
```
|
||||
|
||||
|
@ -133,7 +133,7 @@ var err = new createError.NotFound()
|
|||
|422 |UnprocessableEntity |
|
||||
|423 |Locked |
|
||||
|424 |FailedDependency |
|
||||
|425 |UnorderedCollection |
|
||||
|425 |TooEarly |
|
||||
|426 |UpgradeRequired |
|
||||
|428 |PreconditionRequired |
|
||||
|429 |TooManyRequests |
|
||||
|
|
Some files were not shown because too many files have changed in this diff Show More
Loading…
Reference in New Issue